thank you in advance.
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
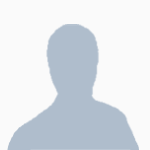
Convert String To Binary?
Started by bfbc2jb, 21 August 2013 - 09:31 PMPosted 21 August 2013 - 11:31 PM
So how do i go about converting a string to a binary sequence, i need this to securely transmit strings over rednet.
thank you in advance.
thank you in advance.
Posted 22 August 2013 - 12:01 AM
You could use string.byte on each character in the string and calculate the binary equivalent of that decimal number.
local function decimalToBinary (number)
-- Put your code here. There is more than one algorithm for this :P/>
end
local _string = "Test."
local bin = ""
for character in _string:gmatch ('.') do
bin = bin .. decimalToBinary (character:byte())
end
Posted 22 August 2013 - 12:05 AM
You can use string.byte to know the value of a char. After that you can convert it to binary or encode it, there is many ways.
Here I give you Decimal to Binary and Binary to Decimal. I use that in one of my program.
Note: When I posted this, I didn't saw Grim Reaper message because I was creating this message when he post.
Here I give you Decimal to Binary and Binary to Decimal. I use that in one of my program.
function DB(dec)
local result = ""
repeat
local divres = dec / 2
local int, frac = math.modf(divres)
dec = int
result = math.ceil(frac) .. result
until dec == 0
local StrNumber
StrNumber = string.format(result, "s")
local nbZero
nbZero = 16 - string.len(StrNumber)
local Sresult
Sresult = string.rep("0", nbZero)..StrNumber
return Sresult
end
function BD(bin)
local num = 0
local ex = string.len(bin) - 1
local l = 0
l = ex + 1
for i = 1, l do
b = string.sub(bin, i ,i)
if b == "1" then
num = num + 2^ex
end
ex = ex - 1
end
--return string.format("%u", num) -- If you want a string
return num
end
Note: When I posted this, I didn't saw Grim Reaper message because I was creating this message when he post.
Posted 22 August 2013 - 01:41 AM
Note: When I posted this, I didn't saw Grim Reaper message because I was creating this message when he post.
That, my friend, is called being ninja'd :)/>
Posted 22 August 2013 - 02:13 AM
That's cool, man. You did offer a more thorough solution than I did, after all ;)/>
Posted 22 August 2013 - 08:19 AM
thanks, also clueless i tried your decimal to binary code and it just does nothing, is there anything im missing?You could use string.byte on each character in the string and calculate the binary equivalent of that decimal number.local function decimalToBinary (number) -- Put your code here. There is more than one algorithm for this :P/>/>/> end local _string = "Test." local bin = "" for character in _string:gmatch ('.') do bin = bin .. decimalToBinary (character:byte()) end
Posted 22 August 2013 - 12:37 PM
all of these are excellent suggestions, except for one minor thing. Lua can already do this natively.
tonumber takes an optional 2nd argument, the base of the string to convert.
ex, tonumber("10101",2) would give you 21.
The 2nd arg can be up to base 36 (0-1 plus A-Z).
tonumber takes an optional 2nd argument, the base of the string to convert.
ex, tonumber("10101",2) would give you 21.
The 2nd arg can be up to base 36 (0-1 plus A-Z).
Posted 22 August 2013 - 01:52 PM
Unfortunately, I don't think that lua's tonumber can convert from decimal into binary. Generally, tonumber is converting its first parameter into a lua number which is a double, so I don't think that tonumber converts from base 10 into any other base.
For example, running this code in the lua command line:
However, I'm not entirely sure if this is correct or not. I might just be confusing the parameters when converting from decimal to binary :P/>
Here's a function written by Jajnick to convert a decimal number into a binary string.
For example, running this code in the lua command line:
print (tonumber ("5", 2))
prints nil.However, I'm not entirely sure if this is correct or not. I might just be confusing the parameters when converting from decimal to binary :P/>
Here's a function written by Jajnick to convert a decimal number into a binary string.
function bin(dec)
local result = ""
repeat
local divres = dec / 2
local int, frac = math.modf(divres)
dec = int
result = math.ceil(frac) .. result
until dec == 0
return result
end
From his api: http://www.computercraft.info/forums2/index.php?/topic/4841-decbinhex-api-convert-decimal-numbers-to-binary-and-hexadecimal/Posted 22 August 2013 - 02:46 PM
Yeah, you'll still need a function to convert a number to a binary string. Jajnick's looks good, here's another I've used before…
local hexToBin_lut={ ["0"]="0000", ["1"]="0001", ["2"]="0010", ["3"]="0011", ["4"]="0100", ["5"]="0101", ["6"]="0110", ["7"]="0111", ["8"]="1000", ["9"]="1001", ["a"]="1010", ["b"]="1011", ["c"]="1100", ["d"]="1101", ["e"]="1110", ["f"]="1111", }
local function toBinary(num)
return string.format("%x",num):gsub(".",function(v) return hexToBin_lut[v] end):match("^[0]*(%d*)$")
end
Posted 30 August 2014 - 12:32 AM
code
--lua text => binary ascii codes
function string:split(delimiter)
local result = { }
local from = 1
local delim_from, delim_to = string.find( self, delimiter, from )
while delim_from do
table.insert( result, string.sub( self, from , delim_from-1 ) )
from = delim_to + 1
delim_from, delim_to = string.find( self, delimiter, from )
end
table.insert( result, string.sub( self, from ) )
return result
end
function toBits(num)
local t={}
while num>0 do
rest=math.fmod(num,2)
t[#t+1]=rest
num=(num-rest)/2
end
return t
end
function makeEight( t )
return ("0"):rep( 8 - #t ) .. t
end
function textToBinary( txt2 )
local txt = ""
for i=1, #txt2 do
txt = txt .. makeEight( table.concat( toBits( string.byte( txt2:sub( i, i ) ) ) ):reverse() )
end
return txt
end
function binaryToText( txt )
local txt2 = ""
local t = txt:split( "\n" )
for k, v in pairs( t ) do
q = 1
for i=1, #v / 8 do
txt2 = txt2 .. string.char( tonumber( v:sub( q, q + 7), 2 ) )
q = q + 8
end
end
return txt2
end
EDIT: Oh my God is it really 2014? I'M SORRY FOR NECROING THIS
Edited on 29 August 2014 - 10:33 PM