I need this to do some finishing work like to save files.
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
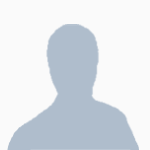
recognize server restarts
Started by Tuvok91, 24 August 2013 - 07:22 AMPosted 24 August 2013 - 09:22 AM
is there a way to recognize a server restart? (something like an event)
I need this to do some finishing work like to save files.
I need this to do some finishing work like to save files.
Posted 24 August 2013 - 12:49 PM
Split into new topic.
No, this is not possible.
No, this is not possible.
Posted 24 August 2013 - 06:12 PM
I believe you can use file.flush to save the file but not close it.
Example:
This way, you don't need to worry about server restarts because everything will be saved.
Example:
local f = fs.open("test", "w")
f.write("Stuff")
f.flush()
f.write("More stuff")
f.close()
This way, you don't need to worry about server restarts because everything will be saved.
Posted 24 August 2013 - 11:11 PM
what ever your doing make it save every x amount of seconds or minutes… seconds more precise to when it shuts down but can be laggy
Posted 25 August 2013 - 02:57 AM
The usual way to safely replace a file in real-world programming is to create a new file with a temporary name, write the data into it, and then rename it on top of the original file. That way, you’re guaranteed that no matter where your program stops running (e.g. crashes), either the old file exists intact or else the new file is fully written—there is no case where you lose the file completely (if you add an fsync call, this is even safe across power failures). I suspect doing something similar in ComputerCraft—writing a temporary file and fs.move-ing it on top of the original—is probably about the best way to be safe while writing files.
Posted 25 August 2013 - 07:58 AM
The usual way to safely replace a file in real-world programming is to create a new file with a temporary name, write the data into it, and then rename it on top of the original file. That way, you’re guaranteed that no matter where your program stops running (e.g. crashes), either the old file exists intact or else the new file is fully written—there is no case where you lose the file completely (if you add an fsync call, this is even safe across power failures). I suspect doing something similar in ComputerCraft—writing a temporary file and fs.move-ing it on top of the original—is probably about the best way to be safe while writing files.
Or just use file.flush(), which saves the file and allows you to continue using it…
See here (Not CC Lua, but it still applies): http://www.lua.org/pil/21.3.html
Posted 25 August 2013 - 12:38 PM
Or just use file.flush(), which saves the file and allows you to continue using it…
See here (Not CC Lua, but it still applies): http://www.lua.org/pil/21.3.html
Not safely, you can’t. What if I do write(x); write(y); flush()? What if the server goes down after write(x) but before write(y)? It might be the case that the original contents of the file are still there. It might be the case that the file is empty, because it was truncated when opening in write mode but you never even got to the write(x). It might be empty because the open truncated it, the code did get to write(x) and write(y), but the shutdown happened before you got to the flush. Or, alternatively, maybe it only has x in it but not y, because the system decided to flush buffers after the write of x (maybe x was fairly large). In all these cases, you see data loss, and flush is not sufficient to safely replace a file. The only safe solution is to write the new data to a different file and be sure it’s fully on-disk before deleting the old file—flush is part of that, but not all of it.
Posted 25 August 2013 - 01:02 PM
Not safely, you can’t. What if I do write(x); write(y); flush()? What if the server goes down after write(x) but before write(y)?
write(x) flush()
write(y) flush()
The point is that you use flush after each write.It might be the case that the original contents of the file are still there. It might be the case that the file is empty, because it was truncated when opening in write mode but you never even got to the write(x). It might be empty because the open truncated it, the code did get to write(x) and write(y), but the shutdown happened before you got to the flush. Or, alternatively, maybe it only has x in it but not y, because the system decided to flush buffers after the write of x (maybe x was fairly large). In all these cases, you see data loss, and flush is not sufficient to safely replace a file.
What do you think append mode is for? You will experience no data loss if you open the file in append mode. Other than the new data that you're trying to write. This of course would be sad, but it is not something that opening a new file and writing would be able to fix anyway.
The only safe solution is to write the new data to a different file and be sure it’s fully on-disk before deleting the old file—flush is part of that, but not all of it.
This takes more time than a simple flush does (though I'm sure that either way the difference is negligible). What I'm saying is not that you're wrong, per se, it's just that you are not using the most efficient method. Overall, flush is the fastest, easiest, and most reasonable way to save the new data. A flush after each write will ensure that the data is saved, and it has the added bonus of not needing a temporary file.
1) New file? Open in write mode. You have nothing to lose.
2) Adding data? Open in append mode. You will not lose the original content.
3) Modifying old data (as in completely overwriting, not adding) is the only case I can think of where you would want to use temporary files.
Oh and you seem to assume that a crash will occur at the same exact moment as writing to a file. What are the chances of that? Quite low. Flush will save the file in the event that a crash occurs at a later instance, but you still want to use the same file handle, which is I think all that the OP is asking.
Posted 25 August 2013 - 03:15 PM
It might be the case that the original contents of the file are still there. It might be the case that the file is empty, because it was truncated when opening in write mode but you never even got to the write(x). It might be empty because the open truncated it, the code did get to write(x) and write(y), but the shutdown happened before you got to the flush. Or, alternatively, maybe it only has x in it but not y, because the system decided to flush buffers after the write of x (maybe x was fairly large). In all these cases, you see data loss, and flush is not sufficient to safely replace a file.
What do you think append mode is for? You will experience no data loss if you open the file in append mode. Other than the new data that you're trying to write. This of course would be sad, but it is not something that opening a new file and writing would be able to fix anyway.
Well, if you wanted to keep the original data as well as the new data, then sure, use append mode, and none of this matters. Normally one doesn’t though. Most of the time you have some data (maybe a serialized table), and once in a while you decide to update it, and you want to replace the old data with the new data.
The only safe solution is to write the new data to a different file and be sure it’s fully on-disk before deleting the old file—flush is part of that, but not all of it.
This takes more time than a simple flush does (though I'm sure that either way the difference is negligible). What I'm saying is not that you're wrong, per se, it's just that you are not using the most efficient method. Overall, flush is the fastest, easiest, and most reasonable way to save the new data. A flush after each write will ensure that the data is saved, and it has the added bonus of not needing a temporary file.
1) New file? Open in write mode. You have nothing to lose.
2) Adding data? Open in append mode. You will not lose the original content.3) Modifying old data (as in completely overwriting, not adding) is the only case I can think of where you would want to use temporary files.
Oh and you seem to assume that a crash will occur at the same exact moment as writing to a file. What are the chances of that? Quite low. Flush will save the file in the event that a crash occurs at a later instance, but you still want to use the same file handle, which is I think all that the OP is asking.
Of course, for a new file or appending data, you don’t need to do this. For replacing the contents of a file, you do. Also, of course the chance of the server going down at the exact moment you’re writing new data but haven’t finished yet is pretty low, but then, good software engineering practice should be about building software that works, not just software that works most of the time.
Posted 13 September 2013 - 01:52 AM
Sorry for Necro-ing but I am interested in this concept as well. The server I play on restarts every 5 hours. What I want to do is send turn on a redstone signal before the server restarts.
Usually I could just make the startup file:
However, direwolf20 pack is a little buggy, such that when the server restarts, the world will not load until a player is in that spot, even when I have my areas loaded with chunk loaders.
So, does anyone have any ideas? Can I check what real world time the server restarted maybe and modify the sleep duration to whatever is left of that 5hrs?
Usually I could just make the startup file:
sleep(<however many seconds 4.9hrs is>)
rs.setOutput("<side>", true)
However, direwolf20 pack is a little buggy, such that when the server restarts, the world will not load until a player is in that spot, even when I have my areas loaded with chunk loaders.
So, does anyone have any ideas? Can I check what real world time the server restarted maybe and modify the sleep duration to whatever is left of that 5hrs?
Posted 13 September 2013 - 02:06 PM
If the server regularly posts a warning in chat, you could use that "chat" peripheral people keep talking about.
Posted 14 September 2013 - 11:35 AM
I googled "computercraft chat peripheral" and came up with nothing… do you have a link? This sounds like the perfect solution :)/>
Posted 14 September 2013 - 12:19 PM
Try the Chat Box peripheral from MiscPeripherals.
http://www.computercraft.info/forums2/index.php?/topic/4587-cc153mc152-miscperipherals-33/
http://www.computercraft.info/forums2/index.php?/topic/4587-cc153mc152-miscperipherals-33/
Posted 14 September 2013 - 10:19 PM
You're actually amazing! :D/>
Posted 16 September 2013 - 01:37 PM
Thanks! :D/>