Posted 25 August 2013 - 05:31 AM
Title: Need help with a sorting turtle program.
So I've just gotten into this and I'm writing a program to have my turtles sort items. I know this code is basic and redundant, but I only know a few things, so this is what I've got:
Ok, so this robot is designed to accept an item from the robot in front of it (who has rejected it as one of his items) and either A) put it into the box to his left (positiveDrop) or B) hand it to the chest behind it (negativeDrop). I've managed to make this happen with the robot in front by using a loop almost identical to the while loop at the end only for sucking items out of chest rather than being handed to it. The problem I'm having is that for some reason on this turtle the loop won't run forever. So long as the turtle in front is actively handing it items then it works as intended, but if it sits for more than a second or two the program ends. There's no error message, it just ends as if the program completed successfully. What am I missing?
Thanks in advance!
(Also, I know this should be in pastebin or something rather then just Copy/Pasted, but I haven't found how to do that yet.)
In this image the turtle in question is the one on the bottom.
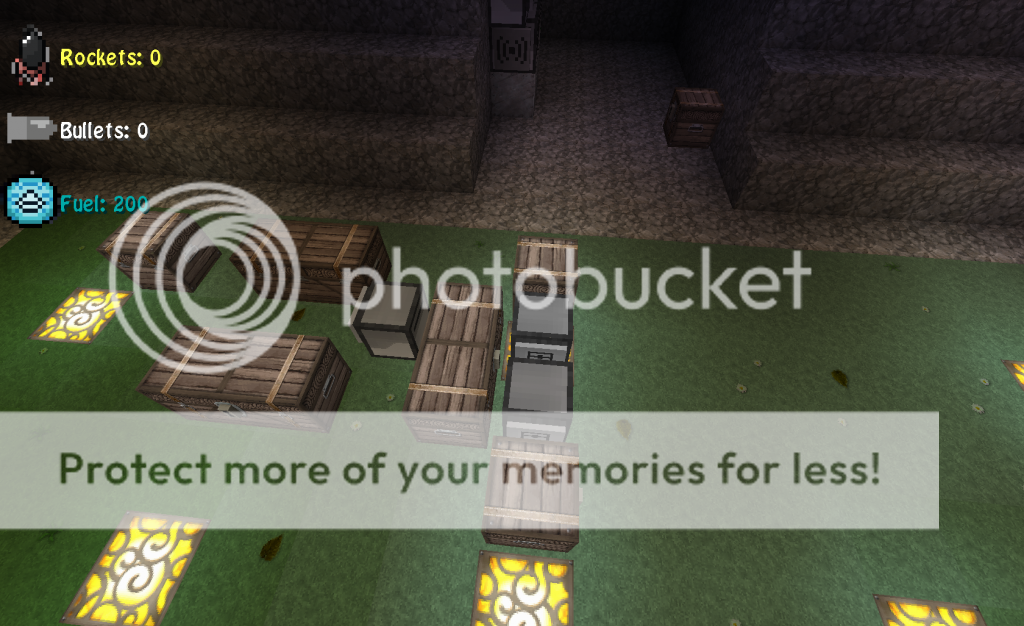
So I've just gotten into this and I'm writing a program to have my turtles sort items. I know this code is basic and redundant, but I only know a few things, so this is what I've got:
function positiveDrop()
turtle.turnLeft()
turtle.drop()
turtle.turnRight()
itemWait()
end
function negativeDrop()
turtle.turnRight()
turtle.turnRight()
turtle.drop()
turtle.turnLeft()
turtle.turnLeft()
itemWait()
end
function itemCompare()
if turtle.compareTo(2) then
positiveDrop()
elseif turtle.compareTo(3) then
positiveDrop()
elseif turtle.compareTo(4) then
positiveDrop()
elseif turtle.compareTo(5) then
positiveDrop()
elseif turtle.compareTo(6) then
positiveDrop()
elseif turtle.compareTo(7) then
positiveDrop()
elseif turtle.compareTo(8) then
positiveDrop()
elseif turtle.compareTo(9) then
positiveDrop()
elseif turtle.compareTo(10) then
positiveDrop()
elseif turtle.compareTo(11) then
positiveDrop()
elseif turtle.compareTo(12) then
positiveDrop()
elseif turtle.compareTo(13) then
positiveDrop()
elseif turtle.compareTo(14) then
positiveDrop()
elseif turtle.compareTo(15) then
positiveDrop()
elseif turtle.compareTo(16) then
positiveDrop()
elseif turtle.getItemCount(1) == 0 then
itemWait()
else negativeDrop()
end
end
function itemWait()
turtle.select(1)
while turtle.getItemCount(1) <= 0 do
itemCompare()
end
end
itemWait()
Ok, so this robot is designed to accept an item from the robot in front of it (who has rejected it as one of his items) and either A) put it into the box to his left (positiveDrop) or B) hand it to the chest behind it (negativeDrop). I've managed to make this happen with the robot in front by using a loop almost identical to the while loop at the end only for sucking items out of chest rather than being handed to it. The problem I'm having is that for some reason on this turtle the loop won't run forever. So long as the turtle in front is actively handing it items then it works as intended, but if it sits for more than a second or two the program ends. There's no error message, it just ends as if the program completed successfully. What am I missing?
Thanks in advance!
(Also, I know this should be in pastebin or something rather then just Copy/Pasted, but I haven't found how to do that yet.)
In this image the turtle in question is the one on the bottom.
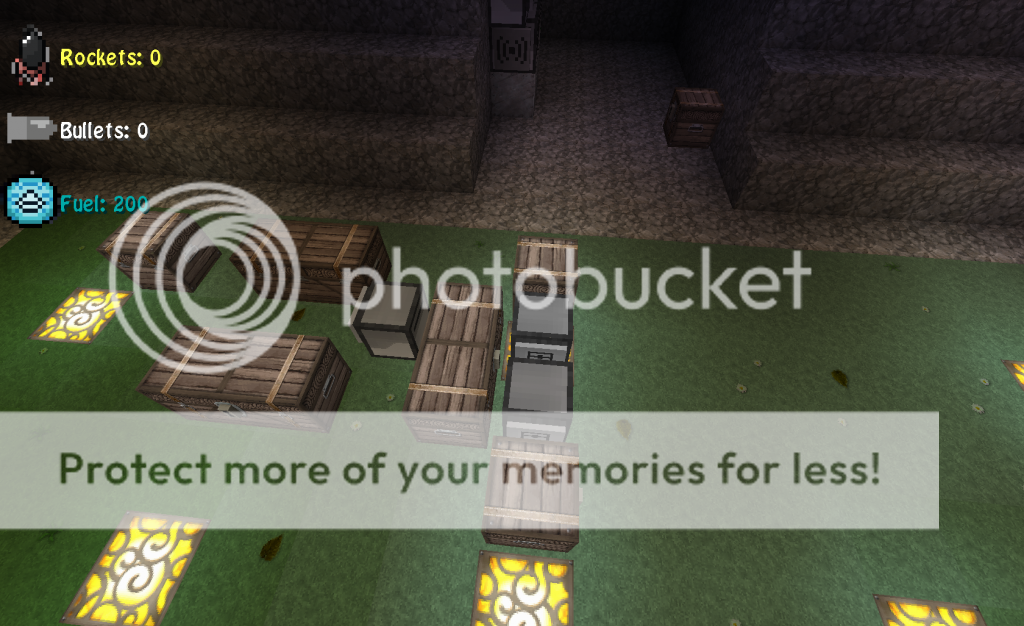
Edited by