This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
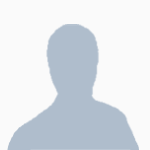
How To Reset Variables?
Started by cmckain14, 20 September 2013 - 05:23 PMPosted 20 September 2013 - 07:23 PM
I am trying to write a farming program and I have a function to run six planting functions but in that function is a loop that uses a variable that needs to reset to the original value after finishing the loop. How would I do this?
Posted 20 September 2013 - 08:46 PM
You simply back it up:
local var = 5
local oldVar = var
for I = 1, 10 do
var = var + I
end
var = oldVar
Posted 20 September 2013 - 09:24 PM
Thanks, but just to ask, what is the point of adding "local" to it?
EDIT: Another question, how would you set up multiple arguments?
EDIT: Another question, how would you set up multiple arguments?
Edited on 20 September 2013 - 07:54 PM
Posted 21 September 2013 - 03:23 AM
"local" prevents you from accidentally interfering with other variables using the same name. If you don't know what it does yet, you probably shouldn't use it.
Multiple arguments to a function or a program?
Multiple arguments to a function or a program?
Posted 21 September 2013 - 07:26 AM
The way I understand it, you should always declare your variables with the local directive, because all of the programs on your computer share a global table. If for some reason there are more than one program running on your computer or turtle and you don't use the local directive then it is highly likely that you could accidentally overwrite the value of a variable in another program with invalid data thus causing that other program to crash and burn.
In summary, in computercraft global variables are not variables that are defined outside of functions, they are variables that are defined without the local directive and they are shared amongst all the programs on the computer.
Meaning I could do the following on one computer and it would be legal;
a program named "animal":
a program named "dog":
I know that is a simple way to put but it is how I understand it and you can readily see that if both of those programs were running and let's say the one called dog also had
Here's hoping I understand the concept. :)/>
Meaning that if you used the local directive on the program called "animal" such that:
All of this makes me think that you could easily setup interprocess communication between programs like you can do with REXX scripting language.
In summary, in computercraft global variables are not variables that are defined outside of functions, they are variables that are defined without the local directive and they are shared amongst all the programs on the computer.
Meaning I could do the following on one computer and it would be legal;
a program named "animal":
aBobCat = "furry"
print("My bobcat is " .. aBobCat)
a program named "dog":
print("My dog is not " .. aBobCat)
I know that is a simple way to put but it is how I understand it and you can readily see that if both of those programs were running and let's say the one called dog also had
if aBobCat == 1 then
print("I am a bobcat!")
end
in it that it would crash and burn horribly with something about comparing a string to a number.Here's hoping I understand the concept. :)/>
Meaning that if you used the local directive on the program called "animal" such that:
local aBobCat = "furry"
print("My bobcat is " .. aBobCat)
then in the program named "dog" you would have a nil error because aBobCat is now undefined.All of this makes me think that you could easily setup interprocess communication between programs like you can do with REXX scripting language.
Posted 22 September 2013 - 05:44 AM
There aren't processes, so interprocess communication should be very difficult.All of this makes me think that you could easily setup interprocess communication between programs like you can do with REXX scripting language.
Posted 22 September 2013 - 05:51 AM
Global variables don't come into _G, only in the environment of that program. Locals don't come anywhere, they don't get saved so to say
Posted 23 September 2013 - 06:05 PM
You make the variable equal to what it was at the beginning of the script, say if the variable was equal to zero at the beginning of the script, then say at the end: (var) = 0, then bring it back to the start of the script again.