Like if i wanted to make a config for something and wanted to read from a certain line of that config how would I do that without having to use something like a filler variable?
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
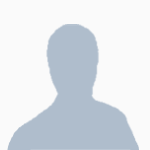
Read A Specific Line?
Started by Dejected, 23 September 2013 - 12:46 PMPosted 23 September 2013 - 02:46 PM
I know about the fs.readLine when a file is open and it reads the next line, but is there a way to read a specific line from the file.
Like if i wanted to make a config for something and wanted to read from a certain line of that config how would I do that without having to use something like a filler variable?
Like if i wanted to make a config for something and wanted to read from a certain line of that config how would I do that without having to use something like a filler variable?
Posted 23 September 2013 - 02:50 PM
Read all of the lines into a table and then index the specific entry you're interested in:
local linesTable = {}
local handle = io.open("config", "r")
if handle then
for line in handle:lines() do
table.insert(linesTable, line)
end
handle:close()
end
local myLine = ""
if #linesTable > 0 then --# make sure the file was read
myLine = linesTable[3] --# get the third line
end
Posted 23 September 2013 - 03:01 PM
Thank you very much!Read all of the lines into a table and then index the specific entry you're interested in:local linesTable = {} local handle = io.open("config", "r") if handle then for line in handle:lines() do table.insert(linesTable, line) end handle:close() end local myLine = "" if #linesTable > 0 then --# make sure the file was read myLine = linesTable[3] --# get the third line end
Wasn't really expecting anyone to answer so fast.
Posted 25 September 2013 - 06:00 PM
Can you explain this part a little more?for line in handle:lines() do
I don't know what that does
Posted 25 September 2013 - 06:44 PM
if handle then
This is used to make sure that the handle isn't a nil reference, meaning that io.open returned nil. This stops us from doing things with a nil variable and causing and error.
for line in handle:lines() do
table.insert (linesTable, line)
end
handle:close()
'lines' is a function which is part of the table returned by io.open. It allows us to iterate over every line in the file. Each line is stored in the variable line and is then stored in the lines table at the next numerical index in 'linesTable'.After the loop ends (all lines are iterated over), the handle is closed.
Posted 25 September 2013 - 06:53 PM
That you, you cleared it up for meThis is used to make sure that the handle isn't a nil reference, meaning that io.open returned nil. This stops us from doing things with a nil variable and causing and error.if handle then
'lines' is a function which is part of the table returned by io.open. It allows us to iterate over every line in the file. Each line is stored in the variable line and is then stored in the lines table at the next numerical index in 'linesTable'.for line in handle:lines() do table.insert (linesTable, line) end handle:close()
After the loop ends (all lines are iterated over), the handle is closed.
Posted 25 September 2013 - 06:54 PM
If I want to store a new value in a config and keep the one there how would I do that?
Nevermind this is how I did it tell me if there is a better way
local options = {}
local x = io.open("Config","r")
for line in x:lines() do
table.insert(options,line)
end
x:close()
local x = fs.open("Config","w"(
for i = 1,#options do
x.writeLine(options[i])
end
ans = read()
x.writeLine(ans)
x.close()
That was only as a test but that works right?
Posted 25 September 2013 - 07:54 PM
If you want to add a new value that you know doesn't exist in the file yet, you could open the file in append mode, write just the new value, and close it.
Posted 25 September 2013 - 08:06 PM
Thanks I can use that but I was looking for a way to save emails into a file and if you don't have an email you can create one. then it connects to the server and checks if that email already exists or not.If you want to add a new value that you know doesn't exist in the file yet, you could open the file in append mode, write just the new value, and close it.