please show me an example :)/>
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
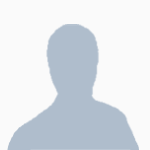
Save Text While Typing?
Started by 1234tree4321, 26 October 2013 - 08:12 AMPosted 26 October 2013 - 10:12 AM
How can i save text while typing it in?
please show me an example :)/>
please show me an example :)/>
Posted 26 October 2013 - 10:33 AM
Er, what do you mean?
Posted 26 October 2013 - 11:52 PM
Below is a basic example. It's what you've asked for, but likely isn't all you want.
You'll probably need to also have it check for line breaks, backspaces, and so on (take a good long look at os.pullEvent() and the key event + API). If you're not sure how to expand it to something more functional, you'll need to provide your current code and specify the exact bits you're not sure how to modify:
You may consider using a timer so that you're not writing the string with every keypress.
You'll probably need to also have it check for line breaks, backspaces, and so on (take a good long look at os.pullEvent() and the key event + API). If you're not sure how to expand it to something more functional, you'll need to provide your current code and specify the exact bits you're not sure how to modify:
local myFile, myText, myEvent
while true do
myEvent = {os.pullEvent()}
if myEvent[1] == "char" then
term.write(myEvent[2])
myText = myText + myEvent[2]
myFile = fs.open("TextFileName","w") -- The old file gets deleted every time.
myFile.write(myText)
myFile.close()
end
end
You may consider using a timer so that you're not writing the string with every keypress.
Posted 27 October 2013 - 12:30 AM
Since when can we concatenate with a nil value and add characters by an actual add symbol?
Initialize myText like so, and not as nil:
local myText = ""
Also we concatenate strings in Lua with double dot:
myText = myText .. myEvent[2]
Bomb bloke, you kinda disappointed me. You are always helping around and making this silly mistakes? :(/>
Initialize myText like so, and not as nil:
local myText = ""
Also we concatenate strings in Lua with double dot:
myText = myText .. myEvent[2]
Bomb bloke, you kinda disappointed me. You are always helping around and making this silly mistakes? :(/>
Posted 27 October 2013 - 12:54 AM
Below is a basic example. It's what you've asked for, but likely isn't all you want.
You'll probably need to also have it check for line breaks, backspaces, and so on (take a good long look at os.pullEvent() and the key event + API). If you're not sure how to expand it to something more functional, you'll need to provide your current code and specify the exact bits you're not sure how to modify:local myFile, myText, myEvent while true do myEvent = {os.pullEvent()} if myEvent[1] == "char" then term.write(myEvent[2]) myText = myText + myEvent[2] myFile = fs.open("TextFileName","w") -- The old file gets deleted every time. myFile.write(myText) myFile.close() end end
You may consider using a timer so that you're not writing the string with every keypress.
I'm new to programming in general but *scratches head*
wouldn't it try and open that file every key press, and sub-sequentially delete the previous data (at least in the write mode?)
So he'd only see the last character (key) pressed if he were to look in the file?
while true do
file = fs.open("myFile", "a")
file.write(io.read() .. "\n")
file.close()
end
Which would save every time you press enter, and to stop you'd need to terminate the program, but it's short and sweet :X
Another way to terminate:
msg = ""
while true do
file = fs.open("myFile", "a")
msg = io.read()
if type(string.find(string.lower(msg), "stophere")) == "number" then
file.close()
return
else
file.write(msg .. "\n")
file.close()
end
end
since string.find returns numbers (starting and ending of instance) if the string exists (and nil if it doesn't?)
So yeah, you'd type "stophere" to stop it instead…
idk, I'm kinda new so idk if it's what you're looking for or anything (started with turtles, peeked into python, present)
Posted 27 October 2013 - 01:53 AM
Not your best help provided on these forums… A bit tired? Off day?-snip-
Yes it would open that file each key press, which is oh so bad!, instead you should open once, then write and flush each loop, but as for only one character showing up in the file, no. Ignoring Bomb Blokes massive string concatenation problem it does rewrite the entire string out each time, meaning that if the file were to be opened there would be the whole text in there.wouldn't it try and open that file every key press, and sub-sequentially delete the previous data (at least in the write mode?)
So he'd only see the last character (key) pressed if he were to look in the file?
It wasn't very smart for Bomb Bloke to immediately assume that this is what the OP wants, he should have waited for a response to ZudoHackz question as the OP was very vague about what they wanted, for example they could just be wanting to make something like the read function, not actually save it to file.
idk how that is meant to be good in any way, I highly suggest against that, honestly its a bit of a stupid method. Here is a bit of a better solution to this odd methodAnother way to terminate:
-code snip-
local msg
local handle = fs.open("someFile", "w")
while true do
msg = read()
if (msg:lower()):find("stophere") then
handle.close()
break
end
handle.writeLine(msg)
handle.flush()
end
However I have to say, that I highly do not recommend this method.As for how it works, in Lua, any value that is not false or nil, will resolve to true in a conditional, so if find returns a number, that evaluates to true, if it returns nil, that is false.
Posted 27 October 2013 - 03:27 AM
Indeed, I was mixing languages. My mind tends to flip back to my BASIC days as both that and Lua allow you take (what I consider to be) great liberties when defining things, though what you can actually get away with does differ between the two. My bad.
Engineer's two fixes would be needed to make it work as I'd actually intended (thanks), though even with those in place, consider it more of a demonstration how to get stuff done "while" typing then something that should be used as-is.
Engineer's two fixes would be needed to make it work as I'd actually intended (thanks), though even with those in place, consider it more of a demonstration how to get stuff done "while" typing then something that should be used as-is.
Posted 27 October 2013 - 11:14 AM
neat, I didn't see see any flush things on the fs api for the wiki :(/>Not your best help provided on these forums… A bit tired? Off day?-snip-Yes it would open that file each key press, which is oh so bad!, instead you should open once, then write and flush each loop, but as for only one character showing up in the file, no. Ignoring Bomb Blokes massive string concatenation problem it does rewrite the entire string out each time, meaning that if the file were to be opened there would be the whole text in there.wouldn't it try and open that file every key press, and sub-sequentially delete the previous data (at least in the write mode?)
So he'd only see the last character (key) pressed if he were to look in the file?
It wasn't very smart for Bomb Bloke to immediately assume that this is what the OP wants, he should have waited for a response to ZudoHackz question as the OP was very vague about what they wanted, for example they could just be wanting to make something like the read function, not actually save it to file.idk how that is meant to be good in any way, I highly suggest against that, honestly its a bit of a stupid method. Here is a bit of a better solution to this odd methodAnother way to terminate:
-code snip-
what do you mean good? what's a better way to check for what a user inputs beyond find O-o
also what are the colons, how the heck does that work lol
Posted 27 October 2013 - 11:27 AM
Yeh sadly the wiki is a little outdated, the flush function was added…………. i want to say 2 or 3 versions ago….. look at that, I was right, what a guess… version 1.55 added missing IO functions.neat, I didn't see see any flush things on the fs api for the wiki :(/>
Ok so there was nothing wrong with you're find, that is actually the most efficient it will get, however the method of continuously reading and then looking for a particular keyword can be improved considerabily, unless the input can go on indefinitely, in which case your method is the only viable method. I would suggest something simpler like "stop" or "done" or multiple keywords. However the main reason that this is not a "good" answer is that it is most likely nothing to do with what the OP asked for, the OP asked for a method of saving answers while typing, not a method of asking for multiple inputs, however will will not know more until the OP has responded.what do you mean good? what's a better way to check for what a user inputs beyond find O-o
The colons are basically Object-Oriented syntactical sugar, so instead of string.find("some string", 'o') we can do ("some string"):find('o') basically it passes the variable its called on as the first argument called "self". We can define these functions ourselves like soalso what are the colons, how the heck does that work lol
local specialType = {
var = "bar",
}
function specialType:foo()
print(self.var)
end
function new()
return setmetatable(specialType, {})
end
we can then invoke the function `foo` like so
local obj = new() --# create the 'object' first
obj.foo(obj) --# prints bar
obj:foo() --# also prints bar
as you can see the second method is much simpler and easier to read. also prone to less mistakes.This is an alright chapter from the PIL to read.
EDIT: also do note we could have defined the function like so
function specialType.foo(this)
print(this.bar)
end
and it will still work with the OO syntactical sugar, just instead of using `self`, we must use `this`Edited on 27 October 2013 - 10:28 AM