Posted 29 October 2013 - 08:27 AM
So I want to build a turtle that will take advantage of a small exploit with oak trees. Seeing as they can grow without any height checking to make was mass lump when you plant:
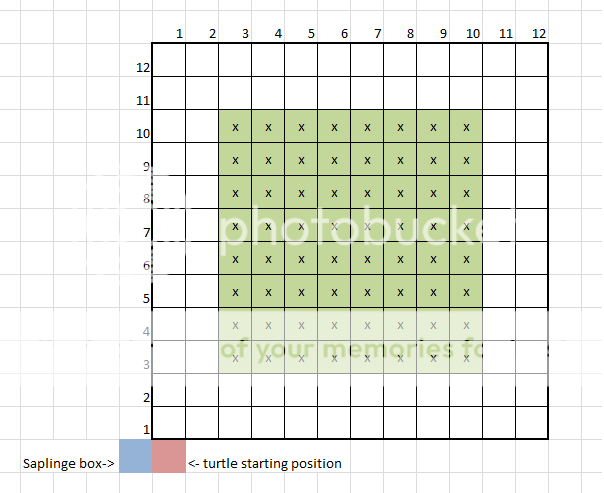
I want to make a turtle that will harvest the mass and return all it picks up then replanting everything
it built one code using my limited skills
pseudo code: http://pastebin.com/hJrVKinT
Actual code: http://pastebin.com/yZ12XKea
but it is long- clunky-
in theory no test yet. it has one issues that i can see right away it replants bottom-left to top-right. when it returns it could easily run into new trees that have grown in the few min it takes. Which really isn't a problem because it could .dig as it returns to stop from getting stuck. Pleas take a look at the code and help me hammer something out I am willing to start from scratch on the code if we need to.
i just started with computer craft 3 days ago so I am sorry for such a long request and so much information.
<any suggested changes please post the code you would use and tell me what you would replace>
i am just as eager to learn as i am to get this code working reliably and properly.
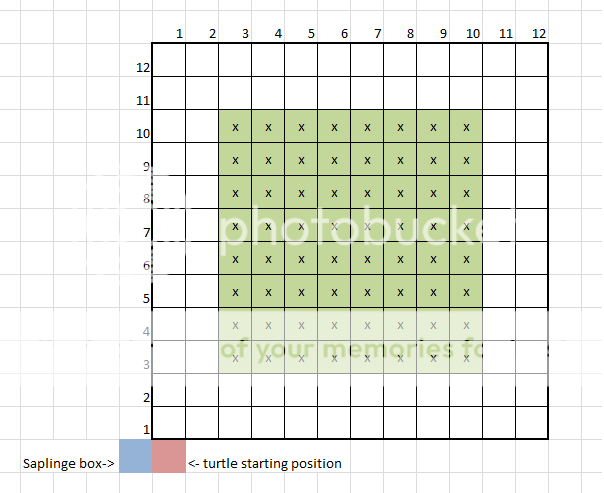
I want to make a turtle that will harvest the mass and return all it picks up then replanting everything
it built one code using my limited skills
pseudo code: http://pastebin.com/hJrVKinT
Actual code: http://pastebin.com/yZ12XKea
but it is long- clunky-
in theory no test yet. it has one issues that i can see right away it replants bottom-left to top-right. when it returns it could easily run into new trees that have grown in the few min it takes. Which really isn't a problem because it could .dig as it returns to stop from getting stuck. Pleas take a look at the code and help me hammer something out I am willing to start from scratch on the code if we need to.
i just started with computer craft 3 days ago so I am sorry for such a long request and so much information.
<any suggested changes please post the code you would use and tell me what you would replace>
i am just as eager to learn as i am to get this code working reliably and properly.