i need him to stop at each area i have identified with blue because i have a line of code to have him dig down and compare the walls for ore.
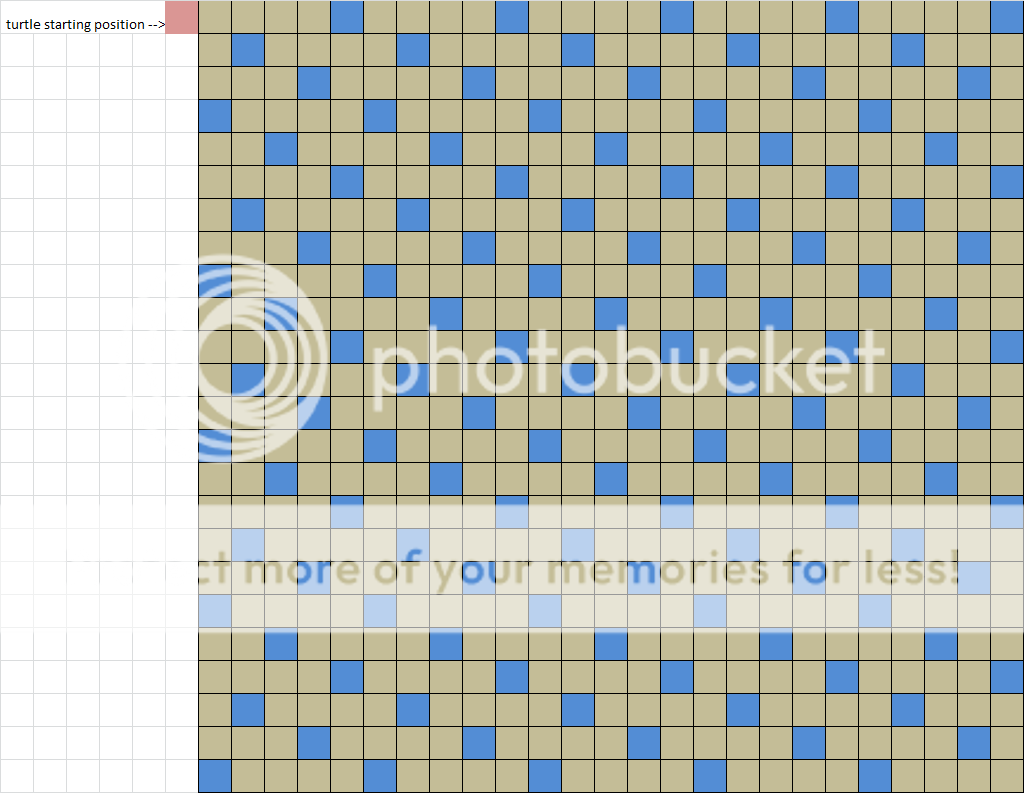
x = 0
function firstRim()
for x >= 0 do
turtle.forward()
turtle.forward()
turtle.forward()
turtle.forward()
turtle.dig()
x = x-1
end
end
firstRim()
if x == 0 then
turtle.turnRight()
firstRim()
end
local tf = turtle.forward
local function td() turtle.digDown() turtle.forward() end
local tn = turtle.turnRight
local tCom = {tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td}
local function arrayShift(tMyArray)
for i=1,2 do
table.insert(tMyArray,1,tMyArray[#tMyArray])
table.remove(tMyArray,#tMyArray)
end
return tMyArray
end
local function myDoit(tArray,dir)
if dir ~= 0 then
for i=1,#tArray do
tArray[i]()
end
else
for i=#tArray,1, -1 do
tArray[i]()
end
end
end
tf()
for i=1,25 do
myDoit(tCom,i%2)
tCom = arrayShift(tCom)
if i%2 == 0 then
tn = turtle.turnLeft
else
tn = turtle.turnRight
end
tn()
tf()
tn()
tf()
end
local alternate = true
if alternate then
turtle.turnRight()
turtle.forward()
turtle.turnRight()
else
turtle.turnLeft()
turtle.forward()
turtle.turnLeft()
end
alternate = not alternate
local function td(a,b,c,d)
-- insert anything you want
-- just end at the same height you started
-- and facing the correct direction
-- and one last thing...turtle.forward() so you don't get your steps off
end
local y = 100
local z = 0
local i = 0
local tf = turtle.forward
local function td()
for z = 1, y do
turtle.digDown()
FullStorage()
RefuelDigger()
for t = 1, 4 do
turtle.select(t)
if not turtle.compare() then
turtle.dig()
end
turtle.turnRight()
end
end
for z = 1, y do
turtle.up()
end
end
function RefuelDigger()
if turtle.getFuelLevel() < 30 then
if turtle.getItemCount(16) == 1 then
turtle.select(16)
turtle.refuel(1)
turtle.select(15)
if turtle.placeUp()then
turtle.select(16)
turtle.suckUp()
turtle.select(15)
turtle.digUp()
end
else
turtle.select(16)
turtle.refuel(1)
end
end
end
function FullStorage()
if turtle.getItemCount(13) > 0 then
turtle.select(14)
if turtle.placeUp() then
for i = 5, 13 do
turtle.select(i)
turtle.dropUp()
end
turtle.select(14)
turtle.digUp()
end
end
end
local tn = turtle.turnRight
local tCom = {tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td}
local function arrayShift(tMyArray)
for i=1,2 do
table.insert(tMyArray,1,tMyArray[#tMyArray])
table.remove(tMyArray,#tMyArray)
end
return tMyArray
end
local function myDoit(tArray,dir)
if dir ~= 0 then
for i=1,#tArray do
tArray[i]()
end
else
for i=#tArray,1, -1 do
tArray[i]()
end
end
end
tf()
for i=1,25 do
myDoit(tCom,i%2)
tCom = arrayShift(tCom)
if i%2 == 0 then
tn = turtle.turnLeft
else
tn = turtle.turnRight
end
tn()
tf()
tn()
tf()
end
will this work?
local y = 100
local z = 0
local i = 0local tf = turtle.forward
local function td()
for z = 1, y do
turtle.digDown()
FullStorage()
RefuelDigger()
turtle.down()
for t = 1, 4 do
turtle.select(t)
if not turtle.compare() then
turtle.dig()
end
turtle.turnRight()
end
end
for z = 1, y do
turtle.up()
end
end
function RefuelDigger()
if turtle.getFuelLevel() < 30 then
if turtle.getItemCount(16) == 1 then
turtle.select(16)
turtle.refuel(1)
turtle.select(15)
if turtle.placeUp()then
turtle.select(16)
turtle.suckUp()
turtle.select(15)
turtle.digUp()
end
else
turtle.select(16)
turtle.refuel(1)
end
end
endfunction FullStorage()
if turtle.getItemCount(13) > 0 then
turtle.select(14)
if turtle.placeUp() then
for i = 5, 13 do
turtle.select(i)
turtle.dropUp()
end
turtle.select(14)
turtle.digUp()
end
end
end
local tn = turtle.turnRight
local tCom = {tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td}local function arrayShift(tMyArray)
for i=1,2 do
table.insert(tMyArray,1,tMyArray[#tMyArray])
table.remove(tMyArray,#tMyArray)
end
return tMyArray
end
local function myDoit(tArray,dir)
if dir ~= 0 then
for i=1,#tArray do
tArray[i]()
end
else
for i=#tArray,1, -1 do
tArray[i]()
end
end
endtf()
for i=1,25 do
myDoit(tCom,i%2)
tCom = arrayShift(tCom)
if i%2 == 0 then
tn = turtle.turnLeft
else
tn = turtle.turnRight
end
tn()
tf()
tn()
tf()
end
doesnt this loop account for all side checking?
for t = 1, 4 do
turtle.select(t)
if not turtle.compare() then
turtle.dig()
end
doesnt this loop account for all side checking?
for t = 1, 4 do
turtle.select(t)
if not turtle.compare() then
turtle.dig()
end
Not really, look how I fixed it and think it thru…
You face foward, only compare vs 1 of the 4 blocks, and if it isn't 1 of those four you dig it… So you're going to di a LOT of blocks.
You want to face, set the dig check as TRUE, and false it, if it matches any of the 4 resources you do not want.
So dig it? YES!
is it stone
is it dirt
is it gravel
is it sand
if all those came back FALSE then yes still dig it….
if any of those toggle dig it false, then it's garbage, move on.
Then turn, and loop again. It's fixed it that pastebin I posted.
local y = 2
local z = 0
local i = 0
local tf = turtle.forward
local function td()
local gooditem = true
for z = 1, y do
turtle.digDown()
while not turtle.down() do
turtle.digDown()
turtle.attackDown()
end
FullStorage()
RefuelDigger()
for i = 1, 4 do
gooditem = true
for t = 1, 4 do
turtle.select(t)
if turtle.compare() then
gooditem = false
end
end
if gooditem then
turtle.select(1)
turtle.dig()
end
turtle.turnRight()
end
end -- added end
for z = 1, y do
while not turtle.up() do
turtle.digUp()
turtle.attackUp()
end
end
turtle.forward()
end
function RefuelDigger()
if turtle.getFuelLevel() < 30 then
if turtle.getItemCount(16) == 1 then
turtle.select(16)
turtle.refuel(1)
turtle.select(15)
if turtle.placeUp()then
turtle.select(16)
turtle.suckUp()
turtle.select(15)
turtle.digUp()
end
else
turtle.select(16)
turtle.refuel(1)
end
end
end
function FullStorage()
if turtle.getItemCount(13) > 0 then
turtle.select(14)
if turtle.placeUp() then
for i = 5, 13 do
turtle.select(i)
turtle.dropUp()
end
turtle.select(14)
turtle.digUp()
end
end
end
local tn = turtle.turnRight
local tCom = {tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td,tf,tf,tf,tf,td}
local function arrayShift(tMyArray)
for i=1,2 do
table.insert(tMyArray,1,tMyArray[#tMyArray])
table.remove(tMyArray,#tMyArray)
end
return tMyArray
end
local function myDoit(tArray,dir)
if dir ~= 0 then
for i=1,#tArray do
tArray[i]()
end
else
for i=#tArray,1, -1 do
tArray[i]()
end
end
end
tf()
for i=1,25 do
myDoit(tCom,i%2)
tCom = arrayShift(tCom)
if i%2 == 0 then
tn = turtle.turnLeft
else
tn = turtle.turnRight
end
tn()
tf()
tn()
tf()
end
function FullStorage()
if turtle.getItemCount(13) > 0 then
for i = 5, 13 do
turtle.select(i)
turtle.dropUp()
end
turtle.select(14)
turtle.digUp()
end
**
local x = 0
local y = 0
local z = 0
local side = 0
function turn(times)
local i
for i=1, times, 1 do
turtle.turnRight()
side = (side +1)%4
end
end
function forward(dist)
local i
for i=1, dist, 1 do
while not turtle.forward() do
turtle.dig()
turtle.attack()
end
if side == 0 then
x = x+1
elseif side == 1 then
y = y+1
elseif side == 2 then
x = x-1
elseif side == 3 then
y = y-1
end
end
end
function mover()
if fuelcheck(10) then
if side == 0 then
if x+5 <= size then
forward(5)
elseif y+1 <= size then
local dist = size-x
forward(dist)
turn(1)
forward(1)
turn(1)
if (x-(dist+3)%5) > 0 then
forward((dist+3)%5)
else
mover()
end
else
return false
end
elseif side == 2 then
if x-5 >= 0 then
forward(5)
elseif y+1 <= size then
local dist = x
forward(x)
turn(3)
forward(1)
turn(3)
if (x-(dist+2)%5) < size then
forward((dist+2)%5)
else
mover()
end
else
return false
end
end
else
print("Not enough fuel!")
refuel()
end
return true
end
The other day, I noticed an API that handles this case well:I was wondering how to code a turtle to pick up were it leaves off for example if the turtle is unloaded or the server is restarted.