Posted 15 December 2013 - 11:47 AM
I made a simple program to raise the ceiling of an underground room:
The problem starts when I run the code. It runs fine, except the turtle decided to do things its own way.
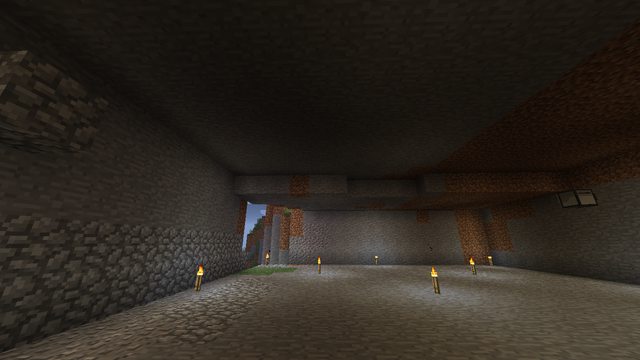
The cobblestone in the top left is where the turtle started. It's supposed to dig all the way to the end of the room, go to the next line, and come back. You can see it went about halfway instead. Also, for some reason it decided to dig an extra block after 6 lines and stopped that a little later.
Did I craft a defective turtle or am I missing something in my code?
(Extra: Originally the code was supposed to allow you to define the volume as well as to dig up or down, but that failed miserably. If you could help me do that, I'd really appreciate it.)
function dig()
for i = 1, 16 do
if turtle.detectUp() then
turtle.digUp()
else
turtle.forward()
end
end
i = 0 -- I added this to see if it would fix it.
end
for l = 1, 17 do
dig()
turtle.digUp()
if l%2 == 1 then
turtle.turnRight()
turtle.forward()
turtle.turnRight()
else
turtle.turnLeft()
turtle.forward()
turtle.turnLeft()
end
end
The problem starts when I run the code. It runs fine, except the turtle decided to do things its own way.
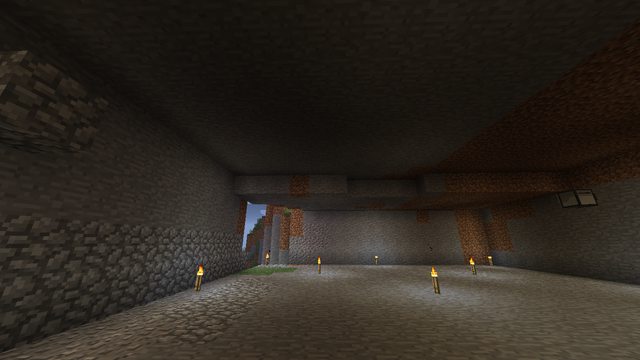
The cobblestone in the top left is where the turtle started. It's supposed to dig all the way to the end of the room, go to the next line, and come back. You can see it went about halfway instead. Also, for some reason it decided to dig an extra block after 6 lines and stopped that a little later.
Did I craft a defective turtle or am I missing something in my code?
(Extra: Originally the code was supposed to allow you to define the volume as well as to dig up or down, but that failed miserably. If you could help me do that, I'd really appreciate it.)