This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
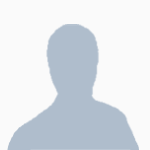
3-D Plane
Started by ilikepie3141, 09 January 2014 - 09:41 PMPosted 09 January 2014 - 10:41 PM
I have looked around and cannot seem to find a program or algorithm to create a 3 dimensional plane. I know voxel sniper has a great algorithm for filling in the area between three points, but unfortunately they are not open source. If anyone has an algorithm or program using turtles to create a 3-d plane that would be awesome.
Posted 09 January 2014 - 11:34 PM
Check out the turtle programs section. A little more detail on what exactly you're looking for would be useful. Are you just trying to create a flat, level surface? Those are pretty easy, you should be able to find plenty of programs that do that.
Posted 09 January 2014 - 11:50 PM
I'm trying to pretty much create a triangle between three points, all with different coordinates. It is not flat in any direction.
Posted 10 January 2014 - 02:39 AM
That sounds like a really fun challenge. I don't recall ever seeing anything that does this. Seems to me whatever method is used for programs that can create circles would be along the right path except you'd have to take it into a new dimension obviously.
Unfortunately I don't think I'll have time to give it a real go. Maybe I'll think about it instead of counting sheep tonight…
Unfortunately I don't think I'll have time to give it a real go. Maybe I'll think about it instead of counting sheep tonight…
Posted 10 January 2014 - 03:33 AM
That could be rather tricky to implement - do you intend to have turtles add/remove themselves from the shape as it expands/contracts? Or are your polys going to be static? Presumably you want to string them together to create more complex shapes?
Is your issue the math of determining where the turtles must go, or the logic of implementing it?
Is your issue the math of determining where the turtles must go, or the logic of implementing it?
Posted 10 January 2014 - 10:22 AM
I think he means using turtles to construct the object, not using turtles as the object.
Posted 10 January 2014 - 10:37 AM
That's a fun challenge!
Just yesterday I used the 3D variant of Pythagorean equation: r = ((x1-x2)^2 + (y1-y2)^2 + (z1-z2)^0.5) This problem would make heavy use of that, I think.
I think I would first think about how to render a diagonal line. Then think about how to construct a diagonal line. Then repeat for the second dimension.
Alternatively, I'd render the whole thing in a 3-dimensional table in memory, then do a 3D print of that. Simpler to write but less efficient for the turtle.
Hmm, I can think of some great practical uses for this, such as massive teraforming.
Just yesterday I used the 3D variant of Pythagorean equation: r = ((x1-x2)^2 + (y1-y2)^2 + (z1-z2)^0.5) This problem would make heavy use of that, I think.
I think I would first think about how to render a diagonal line. Then think about how to construct a diagonal line. Then repeat for the second dimension.
Alternatively, I'd render the whole thing in a 3-dimensional table in memory, then do a 3D print of that. Simpler to write but less efficient for the turtle.
Hmm, I can think of some great practical uses for this, such as massive teraforming.
Posted 10 January 2014 - 10:57 AM
Huh? Shouldn't that ber = ((x1-x2)^2 + (y1-y2)^2 + (z1-z2)^0.5)
d = ((x1 - x2) ^ 2 + (y1 - y2) ^ 2 + (z1 - z2) ^ 2) ^ 0.5
?Posted 10 January 2014 - 07:04 PM
Ah, that'd make sense too, I suppose. I was thinking he might've wanted to make a moving airplane out of his triangular planes. :)/>I think he means using turtles to construct the object, not using turtles as the object.
Posted 11 January 2014 - 02:28 AM
Breakthrough :D/>. There is a way to approximate 3-D lines.
(this was adapted to c++ from here)
So, approximate two sides of the triangle, then for each pair of points that share an axis (x, y, z), approximate that line. This SHOULD give you all the points that make up the plane, but it does not seem like the best way to do this, so if anyone has any better ideas, feel free to suggest.
And now I need to find a way to traverse all these points, any tips or pointers on this would also be much appreciated. For those wondering, I am trying to build an icosahedron, like so. I have built the rectangles and just need to fill in the triangles.
(this was adapted to c++ from here)
#include <iostream>
#include <cmath>
#include <array>
using namespace std;
double round(float d);
int main()
{
float p1[3] = {-476,121,-93};
float p2[3] = {-496,153,-41};
float d[3];
float s[3];
float pTrack[3];
d[0] = p2[0] - p1[0];
d[1] = p2[1] - p1[1];
d[2] = p2[2] - p1[2];
int max = 0;
for (int i = 0; i <3; i++)
{
if (abs(d[i]) > max)
max = abs(d[i]);
}
s[0] = d[0]/max;
s[1] = d[1]/max;
s[2] = d[0]/max;
pTrack[0] = round(p1[0]);
pTrack[1] = round(p1[1]);
pTrack[2] = round(p1[2]);
for (int i = 1; i < max; i++)
{
pTrack[0] = (pTrack[0] + s[0]);
pTrack[1] = (pTrack[1] + s[1]);
pTrack[2] = (pTrack[2] + s[2]);
cout << round(pTrack[0]) << " " << round(pTrack[1]) << " " << round(pTrack[2]) << endl;
}
system ("pause");
return 0;
}
I converted it to c++ because I could not quite understand the language he was using and playing around with the code helped me. Plus telling you that there is a way to do something without showing you seems kinda pointless. Anyways, it shouldn't be that hard to convert this to lua.So, approximate two sides of the triangle, then for each pair of points that share an axis (x, y, z), approximate that line. This SHOULD give you all the points that make up the plane, but it does not seem like the best way to do this, so if anyone has any better ideas, feel free to suggest.
And now I need to find a way to traverse all these points, any tips or pointers on this would also be much appreciated. For those wondering, I am trying to build an icosahedron, like so. I have built the rectangles and just need to fill in the triangles.
Posted 11 January 2014 - 09:46 AM
Yup. A mistake from typing from memory.Huh? Shouldn't that ber = ((x1-x2)^2 + (y1-y2)^2 + (z1-z2)^0.5)?d = ((x1 - x2) ^ 2 + (y1 - y2) ^ 2 + (z1 - z2) ^ 2) ^ 0.5
Posted 11 January 2014 - 09:51 AM
Use 3D interpolation to store 1 of the sides of the triangle in memory. (i.e. Get all of the voxels in the line from one vertex to another.) Let's call this line l. For each voxel in l, use linear interpolation again to draw a line to the unconnected vertex. Make sure to keep a table of all voxels so that they can be constructed later and so we don't ever have two instances of the same voxel. One optimization could be to only continue drawing lines until they intersect very close to other already drawn voxels. This way, we don't continue drawing lines wastefully.