This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
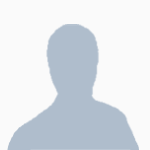
Printing a File Directory
Started by ClassicRockFan, 25 January 2014 - 11:29 PMPosted 26 January 2014 - 12:29 AM
Hello all, I'm trying to make a GUI and I want one of the functionalities of it to when an icon is clicked on, it prints a directory of all of the files on the computer. I have this part working perfectly. I want as well that the colors of the lines that the file names are printed on to have alternating colors. I also have this part working but having a bugger of a time trying to get them to work together. Here is the basic concept of the code I have for it. http://pastebin.com/339JMLMA (I know that there is an extra end at the bottom of the code, I missed this after copying the code to a new file) I also want to know if its possible to use pastebin in conjunction with the files that are created with the paint api. Any help is appreciated. Thanks all.
Posted 26 January 2014 - 02:15 AM
You almost got it, but you probably didn't think about the modulus (%) operand. Try this:
As counter increments, counter % 2 will give the remainder of counter / 2. Essentially, it will be either 0 (even) or 1 (odd). Then the conditional statement in the if control structure will allow us to change the text color accordingly. I started counter at 0, so the first line will be considered even and print in blue in my example. Odd lines will print in pink. I reset the text color to white at the end of the program.
The example I gave above makes the most sense from a the perspective of trying to wrap your brain around the logic, however, you could do the same thing without an if … else control structure like this:
As for the paint.api and pastebin, I got nothing. Somebody else will have to address that.
local FileList = fs.list("")
term.clear()
term.setCursorPos(1,1)
local counter = 0
for _, file in ipairs(FileList) do
if counter % 2 == 0 then
term.setTextColor(colors.blue) -- even lines
else
term.setTextColor(colors.pink) -- odd lines
end
print(file)
counter = counter+1
end
term.setTextColor(colors.white)
As counter increments, counter % 2 will give the remainder of counter / 2. Essentially, it will be either 0 (even) or 1 (odd). Then the conditional statement in the if control structure will allow us to change the text color accordingly. I started counter at 0, so the first line will be considered even and print in blue in my example. Odd lines will print in pink. I reset the text color to white at the end of the program.
The example I gave above makes the most sense from a the perspective of trying to wrap your brain around the logic, however, you could do the same thing without an if … else control structure like this:
local FileList = fs.list("")
term.clear()
term.setCursorPos(1,1)
local counter = 0
local color = {colors.blue,colors.pink}
for _, file in ipairs(FileList) do
term.setTextColor(color[(counter % 2) +1])
print(file)
counter = counter+1
end
term.setTextColor(colors.white)
As for the paint.api and pastebin, I got nothing. Somebody else will have to address that.
Edited on 26 January 2014 - 01:42 AM
Posted 26 January 2014 - 11:33 AM
Thank you very much. I will be sure to give this a try and let you know how it works.
Posted 26 January 2014 - 10:50 PM
Thank you, it worked perfectly. Now, for the next part of my issue, I want to be able to scroll through the files that it prints and show me a directory (if its on a cd or on the pc) and what kind of file it is (paint api file or computercraft code). Can you point me in that direction? Also, I want my startup file to check if my actual gui exists and if not to download the files from pastebin. When I tested this I got the three files I need just fine but it makes three directories, openp, openp2, and openp3. Can you tell me what this is and how to remedy it?
Edited on 26 January 2014 - 10:04 PM
Posted 26 January 2014 - 11:20 PM
You would have need to create in your gui something like this:
I did a bunch of user interface work on my [url='http://www.computercraft.info/forums2/index.php?/topic/16643-titletank-building-turtle/]Tank Building Turtle[/url] code. Take a look at it and steal liberally from whatever is useful. It handles the cursor keys and enter key, changing highlights and reprinting sections of the User Interface. It would probably be helpful to you to tear it apart a bit.
If what I have posted is useful to you (here and above), please vote up each useful post.
Let me know if that gets you started.
- A while loop to capture up, down and enter key events
- On an up or down key event, you would need to know what position you are on the screen and move the highlight text accordingly, essentially reprinting the file on that line in the correct color and highlight. You could also update an informational section on the screen giving more info about that file. As for information what type of file, you would probably have to use file extensions (because all files are text based, and lua code files often aren't given the .lua extension).
- If enter is pressed and the highlight is on a directory, change directories and repaint the screen with the new directory.
- You might need to make the first file in a directory listing ".." which would indicate the user wants to go up a directory level.
I did a bunch of user interface work on my [url='http://www.computercraft.info/forums2/index.php?/topic/16643-titletank-building-turtle/]Tank Building Turtle[/url] code. Take a look at it and steal liberally from whatever is useful. It handles the cursor keys and enter key, changing highlights and reprinting sections of the User Interface. It would probably be helpful to you to tear it apart a bit.
If what I have posted is useful to you (here and above), please vote up each useful post.
Let me know if that gets you started.
Edited on 26 January 2014 - 10:26 PM
Posted 26 January 2014 - 11:39 PM
Here is the pastebin for the startup for file. Just name it as such and all will be good. Let me know what you think. http://pastebin.com/u39xKi22 And I will look at your turtle code. Thanks for all of the help. (Username and pass = test so you can get into the system)
Edited on 26 January 2014 - 10:40 PM
Posted 26 January 2014 - 11:48 PM
I ran it and it worked fine. I can see where you are going with it. I strongly recommend you follow some indent rules for yourself so you don't get lost in the functions and control statements. Keeping some structure to Code Blocks is a good habit to get into.
The startup program correctly downloaded your three files and executed your desktop correctly.
Nice start to your project!
The startup program correctly downloaded your three files and executed your desktop correctly.
Nice start to your project!
Posted 26 January 2014 - 11:57 PM
Thank you. This is one of my first major projects so all the help and TLC is appreciated. Hmmm…. very strange. I just broke the original computer and did it and the then it appeared. But when I put it in a different location it disappeared. Oh well… I also just went back and edited my code.
Edited on 26 January 2014 - 11:01 PM
Posted 27 January 2014 - 12:01 AM
I did as you said, and I did not get the random directories. Have you deleted them and re-run your program?
Not quite sure what you are saying here.
hmmm…. very strange. I just broke the original computer and did it and the then it appeared. But when I put it in a different location it disappeared. Oh well…
Not quite sure what you are saying here.
Edited on 26 January 2014 - 10:59 PM
Posted 27 January 2014 - 12:03 AM
What I mean is I broke the original computer that I tested it on and replaced it in the same location. I then did the pastebin get jibberish startup and it produced my random directories again. However, I made a completely separate computer and did the pastebin get jibberish startup and the random directories didn't show up.
Edited on 26 January 2014 - 11:08 PM
Posted 27 January 2014 - 12:05 AM
Hmmm. You may have had some spurious code you were not aware of. Also, it is a good idea to occasionally reboot your turtle to clear out any erroneous globals you may have created.
Edited on 26 January 2014 - 11:05 PM
Posted 27 January 2014 - 12:09 AM
I was looking at your turtle code and am wondering something, is it possible to make it so that I can have it do the scroll thing but if they right-click, that they are brought back to the screen with the file icon? And at this point, these are the only 4 files on the computer and there are no globals, so who knows…
Posted 27 January 2014 - 12:12 AM
Some other notes on your code. Create a series of variables for your look and feel and use them throughout (even passing them to APIs if you need to). You can make them globals, just remember to clear them when you exit the OS altogether.
For ex.
Make sure to use these variables everywhere you set color. That way, if you change your color schema, you only change it in one place – in the declarations section.
For ex.
defaultDesktopBackground = colors.white
defaultDesktopText = colors.blue
defaultTitleBarBackground = colors.lightBlue
defaultTitlebarText = colors.white
defaultErrorBackground = colors.black
defaultErrorText = colors.red
..and so on
Make sure to use these variables everywhere you set color. That way, if you change your color schema, you only change it in one place – in the declarations section.
Posted 27 January 2014 - 12:14 AM
I will go back and do that for sure.
Posted 27 January 2014 - 12:21 AM
I was looking at your turtle code and am wondering something, is it possible to make it so that I can have it do the scroll thing but if they right-click, that they are brought back to the screen with the file icon? And at this point, these are the only 4 files on the computer and there are no globals, so who knows…
Sure – just monitor a click event in addition to the key event.
For example:
while true do
local event, arg = os.pullEvent()
if event == "mouse_click" and arg == 2 then break
elseif event =="key" then
if arg == 200 or key == 208 then
rolllDirectory(arg)
elseif key == 28 then
doEnterKeyThing()
end
end
end
goBackToWherever(indexNumber)
Does that make sense? The rollDirectory function would handle both up and down keys (it is essentially the same function), so you just pass it the key value as arg. The doEnterKeyThing(indexNumber) would be whatever that is for you, with indexNumber being the current line the highlight is on so it can use that line as a reference. The goBackToWherever() would be getting out of the file list and probably back to the desktop.
Edit: fixed syntax error in my example. And another one. Also, expanded on doEnterKeyThing function.
Edited on 26 January 2014 - 11:39 PM
Posted 27 January 2014 - 12:45 AM
Most of the changes are code based.
Edited on 26 January 2014 - 11:53 PM
Posted 27 January 2014 - 12:49 AM
Worked perfectly for me … now with green desktop background.
This is what I did:
This was the startup code:
This is what I did:
rm login
rm .background
rm .icon
pastebin get Svskf6By startup
startup
This was the startup code:
if fs.exists("login") then
shell.run("login")
else
shell.run("pastebin get 6SJkMMT4 login")
shell.run("pastebin get KaTiSRhy .icon")
shell.run("pastebin get HFYY1efQ .background")
shell.run("login")
end
Edited on 26 January 2014 - 11:52 PM
Posted 27 January 2014 - 12:51 AM
I think its my pastebin limit. Anyway to bypass? Okay it was my pastebin limit. Bypass: Create a new world Most of the changes are code based. Still bug testing and tying to implement the new feature. The line where I'm trying to do this is around line 73. Can you take a look?
Edited on 26 January 2014 - 11:56 PM
Posted 27 January 2014 - 12:56 AM
No – that is not the solution. Here is another idea:
Do you have access to the server in question? Is this running on your desktop? If so, just use a text editor (or ssh into a server and use nano or some other editor). Create the stuff in your text editor and save it to the saves/world/computer/<computerNumber>/<programName>
I guess what I am saying is that while you are developing, do as much of it locally as possible. Add the pastebin features last.
Do you have access to the server in question? Is this running on your desktop? If so, just use a text editor (or ssh into a server and use nano or some other editor). Create the stuff in your text editor and save it to the saves/world/computer/<computerNumber>/<programName>
I guess what I am saying is that while you are developing, do as much of it locally as possible. Add the pastebin features last.
Edited on 27 January 2014 - 12:00 AM
Posted 27 January 2014 - 01:01 AM
I'm not doing this on a server so I have the ability to do pretty much whatever. And I like doing it in game so I can play with it as I go along. But for some reason, now my login button doesn't work
Posted 27 January 2014 - 01:12 AM
I am suspicious of your setup a bit. It seems as if when I run it, I have no trouble. When you run it, fail. I am not sure what is happening there other than some cache issue or some failure to get refreshed files from pastebin. That is why I like to develop locally and then add remote functionality. I use a Mac, so I have all sorts of Lua development options. There are a number of Lua editors for Windows based desktops as well. Lua Edit is one I just looked up. You can keep the code open and save directly to your world/computer/0/myProgram file even while you keep the game up. That way you do not need to deal with the vagrancies of pastebin until you are ready to fully test that. If I destroy a turtle or computer accidentally, I just copy the programs over to the new computer ID or turtle ID folder.
I just tested again on a new computer and on my original computer. It worked fine.
I am calling it a night. I will check on this in the morning some time. Best of luck, you are making progress.
I just tested again on a new computer and on my original computer. It worked fine.
I am calling it a night. I will check on this in the morning some time. Best of luck, you are making progress.
Posted 27 January 2014 - 01:14 AM
Thank you very much for all of your help.
Posted 27 January 2014 - 01:16 AM
More than welcome. I have been exactly where you are – even recently… There are some great coders on this site, and they are always happy to help people who ask well-formed questions and make honest attempts at coding.
Posted 27 January 2014 - 07:25 PM
I will upload a new pastebin with the "final" product as soon as it's ready. Thank you so much
Posted 28 January 2014 - 09:39 AM
Let me know when it is done – I will look at it.
Posted 21 February 2014 - 12:18 AM
I am experiencing some setbacks due to pastebin deleting my files so there will be a great delay as I remake my code.
Posted 21 February 2014 - 05:35 AM
You should make a pastebin account. That way you don't have to write down paste ID's (at least, not to remember them) plus you have no limit iirc.I am experiencing some setbacks due to pastebin deleting my files so there will be a great delay as I remake my code.
I really would recommend to use an external editor like Surferpup already suggested. I read this thread quickly, and saw something about a server.
Really development is way quicker in singleplayer with a proper text editor (Notepad++ or Sublime Text 2) and point the editor to: world/computer/id/program.
You don't have to reboot the computer to run your just saved program, Cc handles that perfectly fine.
One extra tip: make a logging file and log that certain things happen. It helps you with debugging and most likely speed up things.
Posted 21 February 2014 - 08:09 PM
Yeah. It's not that I have a problem with remembering the pastebin, rather that it deleted my file. Couple this with my test world (where I do my coding) getting corrupted, I'm just having some segbacks. Shouldn't be much longer to fix the damage done. And I just recently found a file editor that I like. I'm not sure I understand about the logging file however. I will make a pastebin account for sure. However, I'm not sure I understand about the logging file. Can you elaborate on that?
Edited on 21 February 2014 - 08:33 PM
Posted 22 February 2014 - 08:41 PM
Hey guys, I'm in the final stages of restoring my code and now I can't get one of my old functions to work properly. Can anyone tell me why its not working properly? The error shows up after you left-click on the file icon after the program starts. I can get the selected file(backcolor of yellow) to go up, and back down, but can t get it to go past the first file downward. Also, I want it to go to the bottom of the list if you are at the top (and push the up) and to the top if you are at the bottom (if you are pressing the down key). I have no clue why its not working properly. just download Xa0c6GiD as startup and run it. If you want to look at the code, its in the file() of the file login near line 54. The selected variable is defined near the top of the entire code. Any other tips are welcome.
Posted 22 February 2014 - 09:16 PM
For quick reference, the script in concern is here.
I would re-write the "file()" function as something like:
It still needs further work though; as-is, as soon as the amount of files exceeds the number of lines available on the screen it'll run into trouble. Hopefully it points you in the right direction.
Note that having "file()" call "redrawFile()" (a function which simply calls "file()") was much the same as just having "file()" call "file()". Generally speaking, functions should not call themselves to perform jobs they're already supposed to be doing - this is called recursion, and it fills up the stack with unfinished function calls until Lua runs out of the memory reserved for them and crashes your script.
I would re-write the "file()" function as something like:
Spoiler
function file()
local FileList = fs.list("")
selected = 1
term.setBackgroundColor(fileDirectoryBack)
term.clear()
titleBarPost()
while true do
term.setCursorPos(1,2)
for i=1,#FileList do
if i == selected then
term.setBackgroundColor(fileDirectorySelectedFileColor)
elseif i % 2 == 0 then
term.setBackgroundColor(fileDirectoryBackEven)
else
term.setBackgroundColor(fileDirectoryBackOdd)
end
term.clearLine()
print(file)
end
local event, sCode = os.pullEvent("key")
if sCode == keys.down then
selected = selected + 1
if selected > #FileList then selected = 1 end
elseif sCode == keys.up then
selected = selected - 1
if selected < 1 then selected = #FileList end
elseif sCode == keys.enter then
return selected
end
end
end
It still needs further work though; as-is, as soon as the amount of files exceeds the number of lines available on the screen it'll run into trouble. Hopefully it points you in the right direction.
Note that having "file()" call "redrawFile()" (a function which simply calls "file()") was much the same as just having "file()" call "file()". Generally speaking, functions should not call themselves to perform jobs they're already supposed to be doing - this is called recursion, and it fills up the stack with unfinished function calls until Lua runs out of the memory reserved for them and crashes your script.
Posted 22 February 2014 - 10:53 PM
Okay. Thanks for the tips. I will be adding an addition to deal with large volumes of files. Probably something to the extent of finding the max length of all of the files in the first column and setting x larger than that. Will deal with left and right selection after that. Are there any other comments you have on my script to make it run better or other function ideas?
Edited on 22 February 2014 - 09:55 PM