Sorry ahead of time I'm still trying to learn the best ways to do things and so i'm still very new but I'm trying to make this flatten program that basically you place it and it flattens everything on the level it's placed on. It clears everything upwards as well. However, I can't figure out how to make it keep track of it's columns. In the program it asks what direction do you want the columns to go either left or right. Which I can get it to do but it mines 2 col's perfectly then breaks and goes insane. is there anyway to get the turtle to keep track of what he's doing with out going super insane since I'm trying to understand what stuff does instead of just placing codes. Also does anyone know any good books to read to get good at lua to be able to do some of the crazier stuff? Thanks in advance!
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
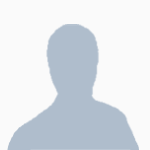
Trying to get a computer to dig in rows and columns?
Started by chardo440, 27 February 2014 - 12:45 AMPosted 27 February 2014 - 01:45 AM
http://pastebin.com/0EKFE8YK
Sorry ahead of time I'm still trying to learn the best ways to do things and so i'm still very new but I'm trying to make this flatten program that basically you place it and it flattens everything on the level it's placed on. It clears everything upwards as well. However, I can't figure out how to make it keep track of it's columns. In the program it asks what direction do you want the columns to go either left or right. Which I can get it to do but it mines 2 col's perfectly then breaks and goes insane. is there anyway to get the turtle to keep track of what he's doing with out going super insane since I'm trying to understand what stuff does instead of just placing codes. Also does anyone know any good books to read to get good at lua to be able to do some of the crazier stuff? Thanks in advance!
Sorry ahead of time I'm still trying to learn the best ways to do things and so i'm still very new but I'm trying to make this flatten program that basically you place it and it flattens everything on the level it's placed on. It clears everything upwards as well. However, I can't figure out how to make it keep track of it's columns. In the program it asks what direction do you want the columns to go either left or right. Which I can get it to do but it mines 2 col's perfectly then breaks and goes insane. is there anyway to get the turtle to keep track of what he's doing with out going super insane since I'm trying to understand what stuff does instead of just placing codes. Also does anyone know any good books to read to get good at lua to be able to do some of the crazier stuff? Thanks in advance!
Posted 27 February 2014 - 02:45 AM
You use "loc" to count upwards movements, but never reset it back to 1.
Your "go()" function is a little bit of a mess:
The basic logic is that if the turtle tries to move forward and fails, it digs, attacks, tries to go forward again… Then checks the loop condition again, which involves trying to move again (very likely fails, since we've already likely moved into the space we dug out), and so on.
Without examining the rest of the script too hard, I'd say you probably want something like this:
You could also make use of this in your leftDig()/rightDig() functions:
Edit: Oh, and don't make your leftDig()/rightDig() functions call each other. That'll loop indefinitely.
Your "go()" function is a little bit of a mess:
function go()
while not turtle.forward() -- Tries to move forward AND checks the result. (Where's the "do"?)
turtle.dig()
turtle.attack
sleep(.5)
turtle.forward() -- Tries to move forward AGAIN. Doesn't check the result, may or may not work.
vDig()
end
end
The basic logic is that if the turtle tries to move forward and fails, it digs, attacks, tries to go forward again… Then checks the loop condition again, which involves trying to move again (very likely fails, since we've already likely moved into the space we dug out), and so on.
Without examining the rest of the script too hard, I'd say you probably want something like this:
function go()
while not turtle.forward() do -- Until one movement forward is successful,
turtle.dig() -- do
turtle.attack -- this
sleep(.5) -- stuff.
end
vDig() -- And once the movement succeeds, do this.
end
You could also make use of this in your leftDig()/rightDig() functions:
function leftDig()
forward()
turtle.turnLeft()
go()
turtle.turnLeft()
end
Edit: Oh, and don't make your leftDig()/rightDig() functions call each other. That'll loop indefinitely.
Edited on 27 February 2014 - 01:47 AM
Posted 27 February 2014 - 03:44 AM
-snip-
the while loop I had I forgot do I was messing with functions must have forgot to re-put it down. I understand the while loops and stuff. I like what you did with the go function. I'm definitely going to use that. How can I use those left dig and right dig functions together though to create columns?
like, the computer asks how many times forward for the rows. then how many times to the left of right depending on your choice.. but how I do I get it to count that? because it'd have do a left dig. then dig the row for row 2 then right dig into a row 3. but say they only want 1 row how would I get it to not even need to run that function?
the while loop I had I forgot do I was messing with functions must have forgot to re-put it down. I understand the while loops and stuff. I like what you did with the go function. I'm definitely going to use that. How can I use those left dig and right dig functions together though to create columns?
like, the computer asks how many times forward for the rows. then how many times to the left of right depending on your choice.. but how I do I get it to count that? because it'd have do a left dig. then dig the row for row 2 then right dig into a row 3. but say they only want 1 row how would I get it to not even need to run that function?
Edited on 27 February 2014 - 03:49 AM
Posted 27 February 2014 - 06:03 AM
Down the bottom of the script, you've got:
Let's re-write just that bit, and ditch the leftDig() and rightDig() functions entirely:
if direction == "Left" then
print("Starting!")
for i=1, y do
leftDig()
rightDig()
end
else
print("Starting!")
for i=1, y do
rightDig()
leftDig()
end
end
Let's re-write just that bit, and ditch the leftDig() and rightDig() functions entirely:
print("Starting!")
for i=1,y do
forward()
if i < y then -- If we're not digging the last row, then...
if direction == "Left" then
turtle.turnLeft()
go()
turtle.turnLeft()
direction = "Right"
else
turtle.turnRight()
go()
turtle.turnRight()
direction = "Left"
end
end
end
Posted 28 February 2014 - 04:37 AM
(Improved code)
http://pastebin.com/j5bwPfHN
Ok everything works man! I didn't even think to switch the variable around like that… I hate asking for help but I've been working on this for a few hours revising my code and such. since it would mine gravel then have like a 3 space air then block on top it wouldnt mine top block so I fixed it. however it's not counting right or something? the turtle digs the flat area. unless there is a hole then it takes the depth of that hole and continues the entire dig at a new depth… so when it comes down to it's main level it's mis counting? I tried messing around with turtle.detect() functions to place the dirt down on the level it's on first before digging up but I feel the while not turtle.forward() makes that hard any ideas?
http://pastebin.com/j5bwPfHN
Ok everything works man! I didn't even think to switch the variable around like that… I hate asking for help but I've been working on this for a few hours revising my code and such. since it would mine gravel then have like a 3 space air then block on top it wouldnt mine top block so I fixed it. however it's not counting right or something? the turtle digs the flat area. unless there is a hole then it takes the depth of that hole and continues the entire dig at a new depth… so when it comes down to it's main level it's mis counting? I tried messing around with turtle.detect() functions to place the dirt down on the level it's on first before digging up but I feel the while not turtle.forward() makes that hard any ideas?
Edited on 28 February 2014 - 03:38 AM
Posted 28 February 2014 - 10:10 AM
The trick here is that you need two counters - one to keep track of how many blocks are dug out between movements (say five bits of sand were above the turtle - after digging them out, it'd need to move upwards five times before searching for another block to dig), and one to keep track of how many blocks you moved upwards in total (so that when the turtle finally hits the surface, it knows how far it needs to travel back down).
This system still won't dealing with floating islands, or even caves the turtle might break into, but I can't see those being handled unless you have the turtle dig up to the top of the world height with each column (technically doable, but the time/fuel costs would be pretty steep).
I'd have the vDig() function as something like this:
This system still won't dealing with floating islands, or even caves the turtle might break into, but I can't see those being handled unless you have the turtle dig up to the top of the world height with each column (technically doable, but the time/fuel costs would be pretty steep).
I'd have the vDig() function as something like this:
Spoiler
function vDig()
local goDown = 0 -- Declaring variables as local to this function ensures they'll
local goUp -- be effectively cleared from RAM once we're done with it.
repeat
goUp = 0
while turtle.detectUp() do -- So long as there are blocks above us,
turtle.dig() -- dig them out
goUp = goUp + 1 -- and count how many we dug.
end
for i=1,goUp do -- Then travel up that many blocks,
while not turtle.up() do turtle.attackUp() end
goDown = goDown + 1 -- while counting the total number we travelled.
end
until not turtle.detectUp() -- Escape the loop if there's nothing above us after moving up.
for i=1,goDown do -- Travel downwards the total number of blocks we went up.
while not turtle.down() do turtle.attackDown() end
end
end
Posted 28 February 2014 - 11:26 PM
hmm now the computer isn't detecting up at all. like it's not digging. it's digging forward then when it detects up it just sits there doesn't spit out any errors.
http://pastebin.com/K6pY2kDV
-edit just removed the block above it's head and it went on forever until it hit y level 256?!?! xD
http://pastebin.com/K6pY2kDV
-edit just removed the block above it's head and it went on forever until it hit y level 256?!?! xD
Edited on 28 February 2014 - 10:29 PM
Posted 01 March 2014 - 12:10 AM
That'd be my accidental use of "turtle.dig()" instead of "turtle.digUp()".
Posted 01 March 2014 - 03:36 AM
Yeah I replaced it I just don't understand why it's still not digging up now. Like it will dig up but it digs up then ends even though gravel falls on its head it just mines once up and sits still. it's like trying to loop to the sky now.
Edited on 01 March 2014 - 03:18 AM
Posted 01 March 2014 - 04:24 AM
Seems there's a slight delay between when gravel starts falling and when the turtle can detect it. Try this snippet:
while turtle.detectUp() do -- So long as there are blocks above us,
turtle.digUp() -- dig them out
sleep(0.8) -- wait a moment
goUp = goUp + 1 -- and count how many we dug.
end