This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
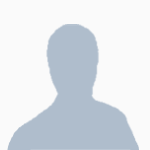
"explain it like im 5" tables
Started by hunter239833, 21 March 2014 - 09:48 PMPosted 21 March 2014 - 10:48 PM
hi, whiile i found i could grasp most of the basic concepts of programming in lua through trial and error, i am perplexed by tables, for some reason i just cannot get them, and i have read through many posts about them, but still no luck. the most i can do is to utilize arguments when i run a program, so i was hoping someone could take me through creating a table, adding to the table, getting values from a table, and also getting data out of a table containing unknown data (such as from a peripheral). im sorry if i am asking a lot, but ive realized the only way i will be able to really use them if i understand what each step is doing and why, thanks!
Edited on 21 March 2014 - 09:56 PM
Posted 21 March 2014 - 11:12 PM
Tables are something that can hold lots of different strings and numbers. Make a table like this:
This is useful for having one variable with everything instead of tons of unnecessary ones. If you do this:
You can also store these to names, rather than numbers, like this:
Tables can also hold functions, and even become function, but that's a lot more complicated, so I won't go over that for now. Check the CC wiki and the Lua 5.1 documentation for more.
table = {}
You have now made a table called 'table'! Change this to:
table = {"foo","bar"}
The table now has the string "foo" and the string "bar" inside it. You can access these strings with variables. For example:
print(table[1])
This will print the first string in the table called 'table' on the screen. Similarly, this:
print(table[2])
will print the second one. You can also store numbers and booleans in tables. For example:
table = {3,"foo",true,"bar",7,false}
will store all of these in one table as table[1], table[2], table[3] etc.This is useful for having one variable with everything instead of tons of unnecessary ones. If you do this:
for i = 1, #table do
print(table[i])
end
it will print every single thing in the table called 'table'.You can also store these to names, rather than numbers, like this:
table = {"foo" = "bar","hello" = "world"}
This means that, while our for loop to print everything won't work anymore as it uses numbers, we can do this:
print(table[foo])
and it will print "bar" or
print(table[hello])
and it will print "world".Tables can also hold functions, and even become function, but that's a lot more complicated, so I won't go over that for now. Check the CC wiki and the Lua 5.1 documentation for more.
Posted 22 March 2014 - 06:07 PM
-snip
Do note that you should never call a table 'table', because 'table' is a reserved keyword in Lua. In fact, never use any reserved keywords as an identifier (variable name).
Posted 23 March 2014 - 02:21 AM
If you haven't seen this tutorial you should check it out; it's a good place to begin - wish I had found it when I was starting with tables. Hope that helps :)/>
Edited on 23 March 2014 - 03:47 PM
Posted 23 March 2014 - 02:45 PM
You can also store these to names, rather than numbers, like this:This means that, while our for loop to print everything won't work anymore as it uses numbers, we can do this:table = {"foo" = "bar","hello" = "world"}
and it will print "bar" orprint(table[foo])
and it will print "world".print(table[hello])
Tables can also hold functions, and even become function, but that's a lot more complicated, so I won't go over that for now. Check the CC wiki and the Lua 5.1 documentation for more.
Those last two aren't quite right. You can either do:
local v = tab.key
or
local v = tab["key"]
Doing what you did would get the value of the globals foo and hello (which is nil) and throw back a nil index error.
Posted 23 March 2014 - 03:18 PM
Also for tables if you wanted to take multiple variables and wanted to line them up like doing usernames and passwords you can do this. This basically checks if user1 which is username1 matches to anything in the table of username. (Which is does)
username = {"username1", "username2", "username3"}
user1 = "username1"
for i, v in pairs(username) do
if user1 == v then
print("User exists!")
else
print("No")
end
end
Edited on 23 March 2014 - 02:22 PM
Posted 23 March 2014 - 03:51 PM
The loop isn't needed if you instead use the keyes for your values. This makes it a lot more efficent when working with large tables.
username = {username1 = true, username2 = true, username3 = true}
user1 = "username1"
if username[user1] then
print("User exists!")
else
print("No")
end
Posted 23 March 2014 - 06:38 PM
The loop isn't needed if you instead use the keyes for your values. This makes it a lot more efficent when working with large tables.username = {username1 = true, username2 = true, username3 = true} user1 = "username1" if username[user1] then print("User exists!") else print("No") end
True ,but it is a lot more effective for for loops because you can use it for other things such as using each username and putting a different value to it like a password instead of doing another if statement.
Posted 23 March 2014 - 06:53 PM
Which again would be less efficent. Just store the password in the username key. All you need is still one if statment.
if password == username[user1] then
Posted 25 March 2014 - 01:51 AM
wow, thanks guys, and sorry for getting back late, some stuff came up again . interesting, that clears it up a bit, but im still a bit confused on them, so could i perhaps throw up some snippets of code and have you tell me if im doing it right?
Posted 25 March 2014 - 04:51 AM
Go for it! Don't forget to use CODE tags :)/>
Posted 26 March 2014 - 12:45 AM
after doing some tests, i have a basic understanding of tables that i create, and how to use them. i am still having problems with tables that i dont create, such as from a peripheral, how would i get the data out of those?
Posted 26 March 2014 - 12:56 AM
(for some background, i am trying to pull info out of a table that i got from a openp scanner, the player data table in particular) everything i try just leads me to either it printing "1", "1table:*number*" just the table and number or "attempt to concate string and table, i assume this means that the table i got from the scanner contains other tables, and if so i am even more confused than before, help?
Posted 26 March 2014 - 01:03 AM
wait! i managed to figure it out by complete chance, for some reason printing the key and the value separately prints it correctly, thank you guys! one step closer to the augmented reality program i am working on!
edit: im not sure how to delete a post, so sorry for posting multiple replies, i have more questions, turns out there was a table in that table (dawg), how would i access that table? and also, is there a way to print a large table to that it doesnt go off screen? (i may be able to do this myself, im working on it as i post these)
edit: im not sure how to delete a post, so sorry for posting multiple replies, i have more questions, turns out there was a table in that table (dawg), how would i access that table? and also, is there a way to print a large table to that it doesnt go off screen? (i may be able to do this myself, im working on it as i post these)
Edited on 26 March 2014 - 12:08 AM
Posted 26 March 2014 - 01:11 AM
Let's say you did something like this:
Say one of the keys in the table, "hunter239833" for eg, was identified as another table.
To list what's in that, you could then pull:
for key,value in pairs(playerTable) do
print(key.." is of the type "..type(value))
end
Say one of the keys in the table, "hunter239833" for eg, was identified as another table.
To list what's in that, you could then pull:
for key,value in pairs(playerTable["hunter239833"]) do
print(key.." is of the type "..type(value))
end
Posted 26 March 2014 - 01:19 AM
that worked, but another sort of problem… another table! i expect i do exactly the same thing? (tableception, am i right?)
Posted 26 March 2014 - 01:25 AM
ok, it worked, im going to quit posting on this topic now