Posted 22 May 2012 - 02:41 AM
I started working with CC and Lua a few days ago, and I've been working on this ATM for three. I am definitely stuck. I'm using Industrial Credits as currency, but my setup should work just as easily with any item used as currency, or even a combination of currencies.
The ATM:
There is a chest for depositing ICs into, and a computer next to it. The computer asks how many credits to deposit (amount), then asks to deposit those credits and waits for the user to press enter. The computer sends a pulse to the orange wire (amount) times.
Each pulse causes a retriever (orange) to suck one credit out of a different box and into another retriever (purple). This is so that if the purple retriever has more credits in it than are in the deposit box, it will not suck any of the credits through. It will also not suck anything through that is not a credit.
Say the amount is five, so the orange retriever sucks five credits out and into the purple retriever. Then the program sends one pulse to the purple retriever. If there are five credits or more, the retriever will suck five credits out of the deposit box. If not, then it won't suck any.
Here's the problem. I have an item detector behind the purple retriever that is hooked up to white wire. It sends a pulse when any items are detected, which won't happen if there were less than five credits in the box. The idea is that if after a certain time, no pulse is detected from the white wire, it will go to the deficiterror() function and tell the user to deposit more credits. If a pulse is detected, the deposit() function should run, which thanks the user for their deposit, runs the reset() function (it should send five pulses to a blue retriever, which sucks the credits back out of the purple retriever), and restarts the ATM program (currently through use of a function).
In earlier versions of the program, it would either always run the reset() function, even if there weren't enough credits in the deposit box (and in some versions wouldn't even suck the credits from the box), or it would always run the deficiterror() function, even if an appropriate amount of credits were in the box and had been sucked up by the purple retriever.
In the current version of the program, the orange and purple retrievers work fine, and five credits are sucked through, which trips the item detector as it should. However, the computer only displays:
Here's my code. I'm pretty sure the main problem is in the check() function because I honestly have no idea how to detect the item detector pulse correctly and at the right time. Besides that, please let me know if there's any way I can organize my code better. I've never done any real coding before a few days ago.
Here is a picture of the setup. All the wireless transmitters are for indicator lights, and the receiver hooked up to the white wire is so I could turn the white wire on and off manually to test the computer's response with testing programs.
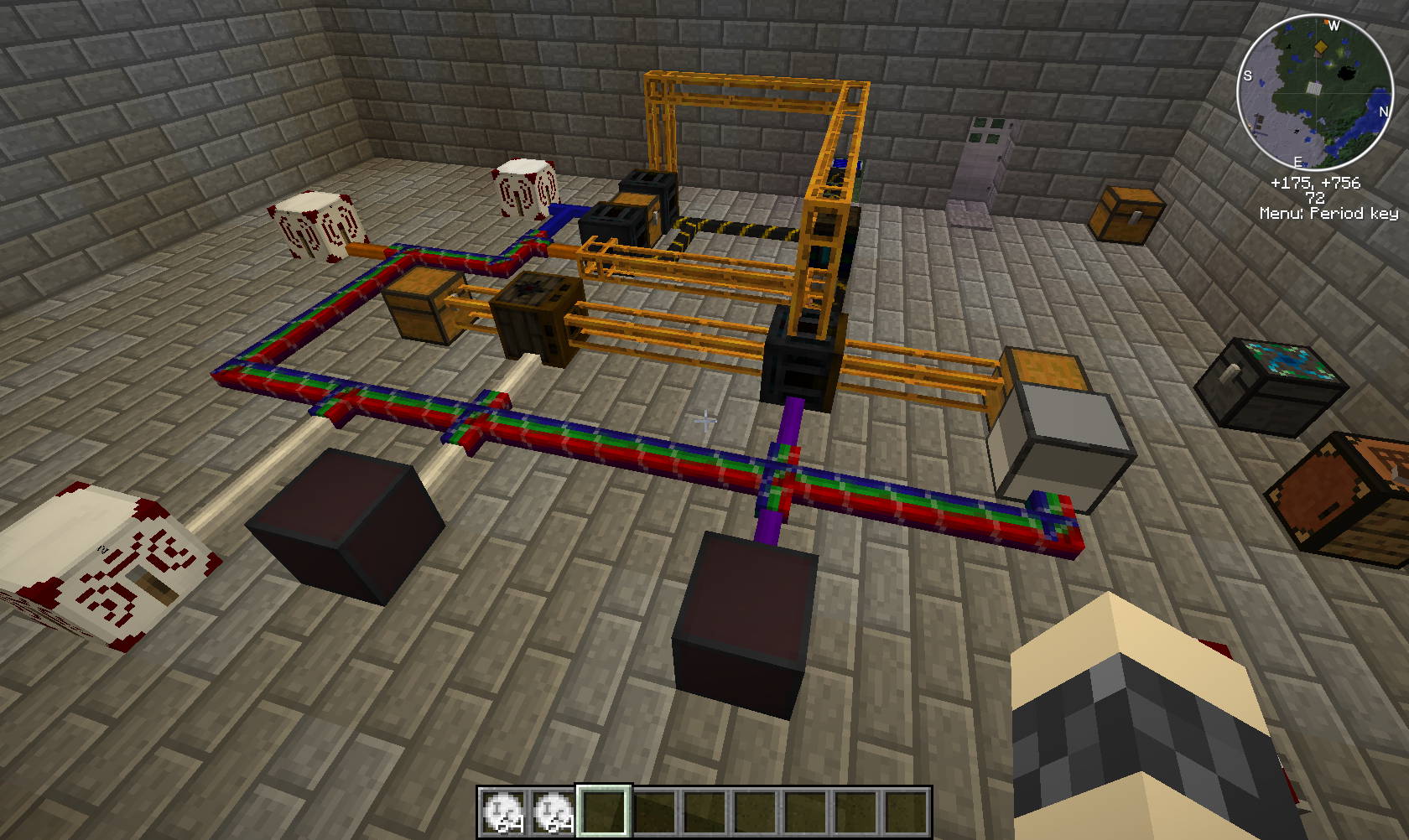
(Oh my god this picture is huge)
The ATM:
There is a chest for depositing ICs into, and a computer next to it. The computer asks how many credits to deposit (amount), then asks to deposit those credits and waits for the user to press enter. The computer sends a pulse to the orange wire (amount) times.
Each pulse causes a retriever (orange) to suck one credit out of a different box and into another retriever (purple). This is so that if the purple retriever has more credits in it than are in the deposit box, it will not suck any of the credits through. It will also not suck anything through that is not a credit.
Say the amount is five, so the orange retriever sucks five credits out and into the purple retriever. Then the program sends one pulse to the purple retriever. If there are five credits or more, the retriever will suck five credits out of the deposit box. If not, then it won't suck any.
Here's the problem. I have an item detector behind the purple retriever that is hooked up to white wire. It sends a pulse when any items are detected, which won't happen if there were less than five credits in the box. The idea is that if after a certain time, no pulse is detected from the white wire, it will go to the deficiterror() function and tell the user to deposit more credits. If a pulse is detected, the deposit() function should run, which thanks the user for their deposit, runs the reset() function (it should send five pulses to a blue retriever, which sucks the credits back out of the purple retriever), and restarts the ATM program (currently through use of a function).
In earlier versions of the program, it would either always run the reset() function, even if there weren't enough credits in the deposit box (and in some versions wouldn't even suck the credits from the box), or it would always run the deficiterror() function, even if an appropriate amount of credits were in the box and had been sucked up by the purple retriever.
In the current version of the program, the orange and purple retrievers work fine, and five credits are sucked through, which trips the item detector as it should. However, the computer only displays:
Hello, how many credits would you like to deposit today?
5 -- the amount
Please deposit your credits into the chest. Press enter when done.
until terminated. In addition, an indicator light (red lumar lamp) hooked up to white wire remains on after the items have passed through the item detector. I'm not sure if it's a bug on my end or not, though, because the light stays on but the glow around the lamp does not.Here's my code. I'm pretty sure the main problem is in the check() function because I honestly have no idea how to detect the item detector pulse correctly and at the right time. Besides that, please let me know if there's any way I can organize my code better. I've never done any real coding before a few days ago.
function ATM()
term.clear()
term.setCursorPos(1,1)
amount = 0
print("Hello, how many credits would you like to deposit today?")
amount = io.read()
print("Please deposit your credits into the chest. Press enter when done.")
enter = io.read()
amount = tonumber(amount)
end
function sort()
for count = 1, amount, 1 do
rs.setBundledOutput("left", colors.orange)
sleep(.2)
rs.setBundledOutput("left",
rs.getBundledOutput("left") - colors.orange)
sleep(.2)
end
end
function retrieve()
sleep(5)
rs.setBundledOutput("left", colors.purple)
sleep(.2)
rs.setBundledOutput("left",
rs.getBundledOutput("left") - colors.purple)
end
function check()
dd = rs.getBundledInput("left")
xx = colors.test(dd, colors.white)
for timer=0, 5, 1 do
event, param1 = os.pullEvent()
if event == "redstone" and xx == "true" then
deposit()
end
sleep(1)
end
deficiterror()
end
function deposit()
print("Thank you for depositing "..amount.." credits. Have a nice day!")
reset()
sleep(5)
ATM()
end
function deficiterror()
print("Error: The number you entered and the number of credits you deposited do not match. Please deposit additional credits and press enter to continue your transaction.")
enter = io.read()
for count = 1, amount, 1 do
retrieve()
end
check()
end
function reset()
for reset = 0, amount, 1 do
rs.setBundledOutput("left", colors.blue)
sleep(.2)
rs.setBundledOutput("left",
rs.getBundledOutput("left") - colors.blue)
sleep(.2)
end
end
ATM()
sort()
retrieve()
check()
Here is a picture of the setup. All the wireless transmitters are for indicator lights, and the receiver hooked up to the white wire is so I could turn the white wire on and off manually to test the computer's response with testing programs.
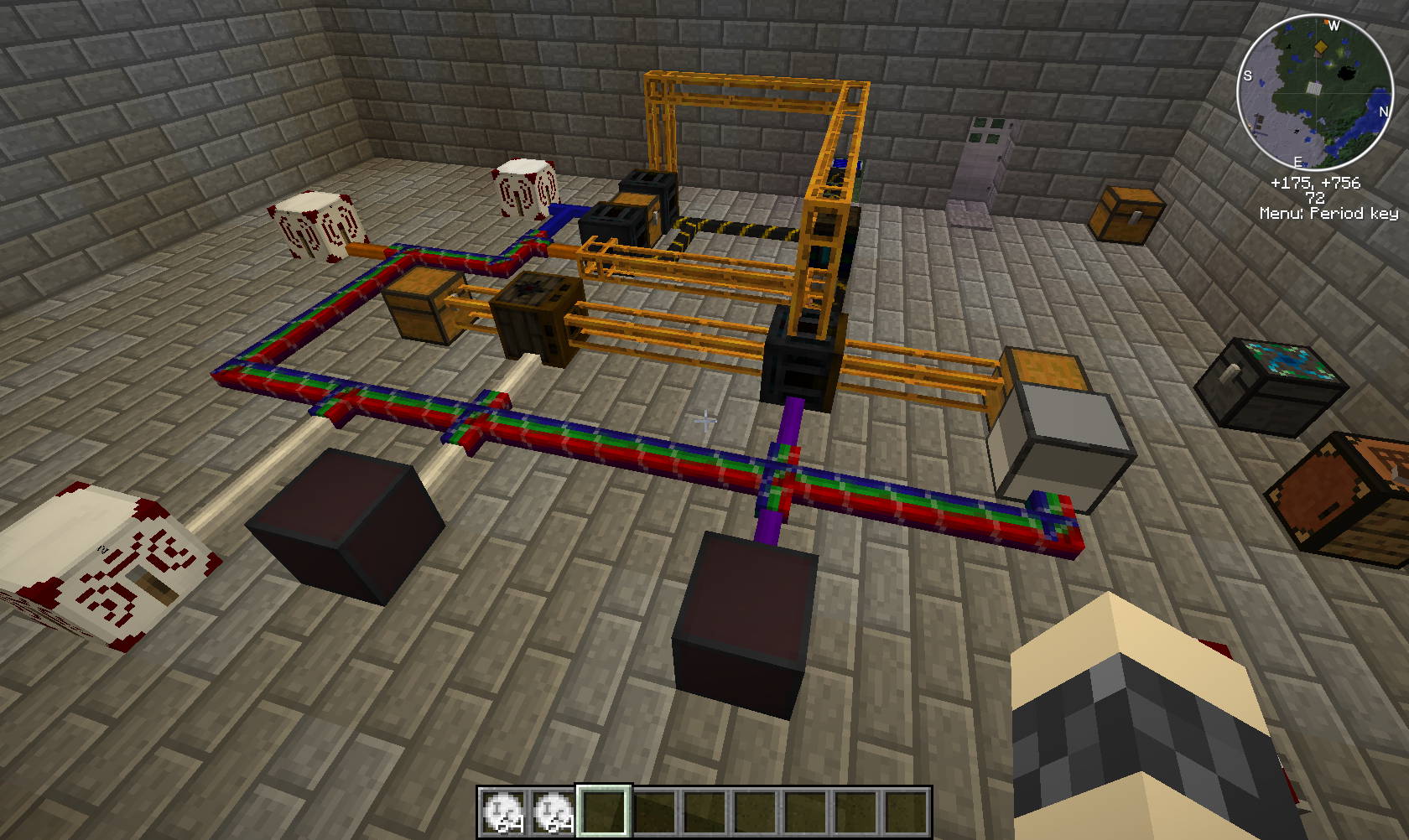
(Oh my god this picture is huge)
Edited on 22 May 2012 - 12:49 AM