please note, English is not my first language.
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
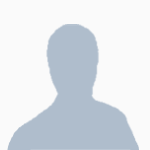
not (yet) real object oriented stuff
Started by blipman17, 04 May 2014 - 02:20 PMPosted 04 May 2014 - 04:20 PM
hello, I am currently breaking up my programs/API's into separate files to make it easyer to update to cc 1.6.x. if it is stable and wide supported. But now I need something to do which is near object oriented stuff, which I am not verry good at (read: trying to understand.) If I want to call a function TWICE, how do I make sure that it is executed as a new instance in LUA? (Hope that this is a good terminology.)
please note, English is not my first language.
please note, English is not my first language.
Posted 04 May 2014 - 06:21 PM
You could use coroutine to run in a "separate" thread.
Also try looking at http://pastebin.com/t0Nb6pbS, it might help you understand OOP in Lua a bit
Also try looking at http://pastebin.com/t0Nb6pbS, it might help you understand OOP in Lua a bit
Edited on 04 May 2014 - 04:21 PM
Posted 04 May 2014 - 07:24 PM
coroutine wouldn't store values in the scope of that routine as far as I know. (tell me if I'm wrong.)
and I know I can make my own functions and use them multiple times, but I want to make whole API's which I want to be able to trash, rewrite and replace as a whole. (like an object.) I just don't know how.
and I know I can make my own functions and use them multiple times, but I want to make whole API's which I want to be able to trash, rewrite and replace as a whole. (like an object.) I just don't know how.
Posted 04 May 2014 - 07:41 PM
Take a look at the vector API included with ComputerCraft, it may be similar to what you're looking for.
Posted 04 May 2014 - 07:46 PM
I'm sorry, I think I stated my question wrong.
How do you bind a value to the scope of a program, and after executing be able to get the value back once you execute the program another time.
And how could you make that last impossible for other programs?
or am I asking something impossible?
edit:
I know what the Vector API does and don't want to use it because I don't need to.
It doesn't have anything in common with my problem.
How do you bind a value to the scope of a program, and after executing be able to get the value back once you execute the program another time.
And how could you make that last impossible for other programs?
or am I asking something impossible?
edit:
I know what the Vector API does and don't want to use it because I don't need to.
It doesn't have anything in common with my problem.
Edited on 04 May 2014 - 05:48 PM
Posted 04 May 2014 - 07:59 PM
I have no idea what you are talking about with next run of that program (still cant figure out what you want) but you may want to use this:
file:myprogram
file:myprogram
dofile("myapi")
test=api:new()
test.hello()
file:myapiapi={
new=function(this)
object={}
setmetatable(object,this)
this.__index=this
return object
end,
hello=function()
print("hello world!")
end,
}
Edited on 04 May 2014 - 06:00 PM
Posted 04 May 2014 - 08:14 PM
I believe Lyqyd was referring to its approach at object orientation, not the actual functionality itself. So you could either have a look at the vector API's source or do what viluon said.I know what the Vector API does and don't want to use it because I don't need to.
It doesn't have anything in common with my problem.
Posted 04 May 2014 - 08:47 PM
starting to read a bit about chunks. see you in a bit. ;)/>
Posted 04 May 2014 - 11:40 PM
Thank you, I think that I am starting to understand what I am able to do. The method to tackle this kind of things is in metatables? declaring a metatable redefines an operand of everything in that table? If so, you can let a program write its own metatable and re-use it?
Edited on 04 May 2014 - 09:41 PM
Posted 05 May 2014 - 12:04 AM
any local variable is bound to the scope of the program, however once that scope ends, that is the end of the variable.How do you bind a value to the scope of a program, and after executing be able to get the value back once you execute the program another time.
Impossible, anything and everything is accessible in Lua, and even if you encrypt files, due to the open source nature of ComputerCraft they'd just be able to look up your encryption details and reverse it; if they really wanted that data!And how could you make that last impossible for other programs?
or am I asking something impossible?
well only when you implement a metamethod, for example if you define __add then every time a + is used with your object it will call your method.declaring a metatable redefines an operand of everything in that table?
I have a feeling that you're getting persistence and Object-Oriented confused here; although I don't know how!you can let a program write its own metatable and re-use it?
Object-Oriented programming is aimed at being able to make 'objects' which to put it simply allow you to do two things. The first things is it allows you to hide implementation details, for example you could have a method that calls various other methods during its process, and accesses some hidden variables, and the person invoking the original method could be none the wiser of its implementation, just the result (this is called encapsulation). The second thing it allows is for you to be able to make instances (objects) of your code, each having their own variable scope meaning any variable you change in an Object only changes for that object, not any other instance.
Now in terms of persistence… In ComputerCraft the only data that is persistent across program restarts is any variable in the global scope, however do note the global scope isn't persistent across computer restarts. Your best bet for persistence is to write the information you need out to file.
Assuming that you do actually want Objects, and are not after persistence then the Vector API is definitely a good example of how you can implement an object class. In your API you would have a single function — new — which you would invoke each time you want a new instance (object) of said API.I know what the Vector API does and don't want to use it because I don't need to.
It doesn't have anything in common with my problem.
Posted 05 May 2014 - 09:07 AM
I think i got it, thank you all for your effort. I'm off to a few hours of trail and error.
Posted 05 May 2014 - 02:10 PM
There is a really good Source for learning OOP for Lua:
http://www.lua.org/pil/16.html
For me it was amazing what Lua has all "undercover" to surprise.
The most tricky thing (what I've never seen bevore in any other Language) is the . and : Notation. Please note that or you will struggle about Lua's OOP.
http://www.lua.org/pil/16.html
For me it was amazing what Lua has all "undercover" to surprise.
The most tricky thing (what I've never seen bevore in any other Language) is the . and : Notation. Please note that or you will struggle about Lua's OOP.
Posted 06 May 2014 - 12:39 AM
Not overly… You can do OO without using the colon notation exampleThe most tricky thing (what I've never seen bevore in any other Language) is the . and : Notation. Please note that or you will struggle about Lua's OOP.
local vec = Vector.new(1,1,1)
--# colon notation
print(vec:round())
--# dot notation
print(vec.round(vec)