Posted 20 July 2014 - 01:13 AM
I'm trying to write a function that would use the paintutils to draw a box of a certain size at a certain position.
However this is what happens with my code:
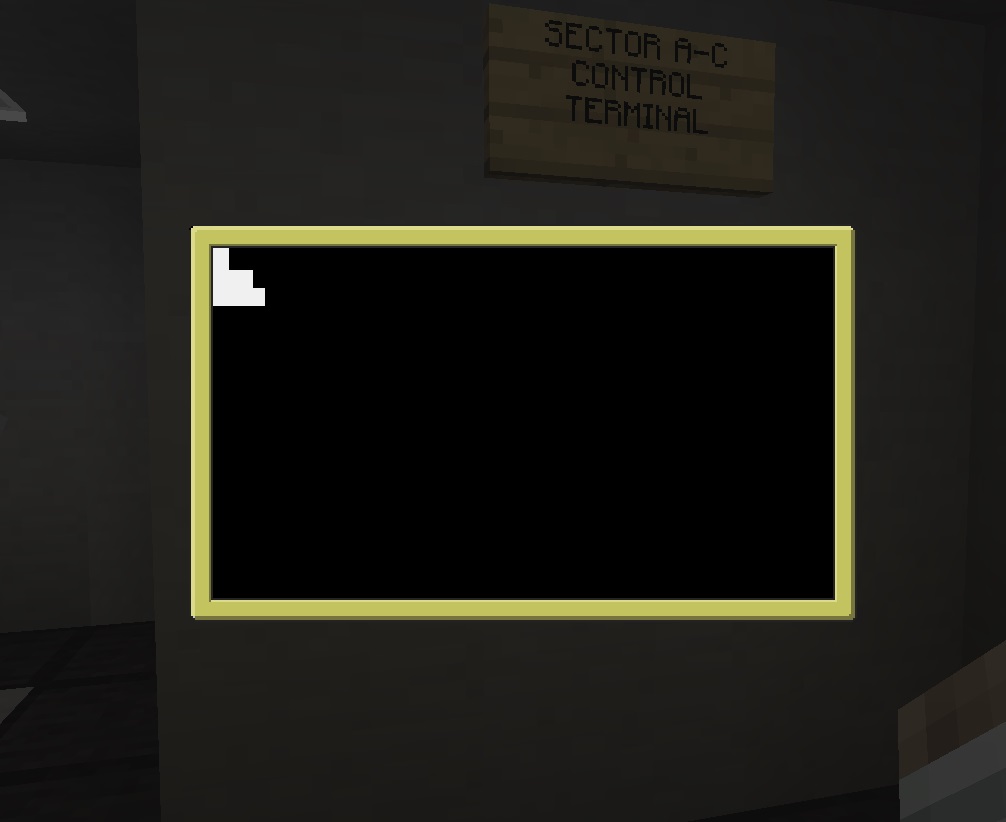
However this is what happens with my code:
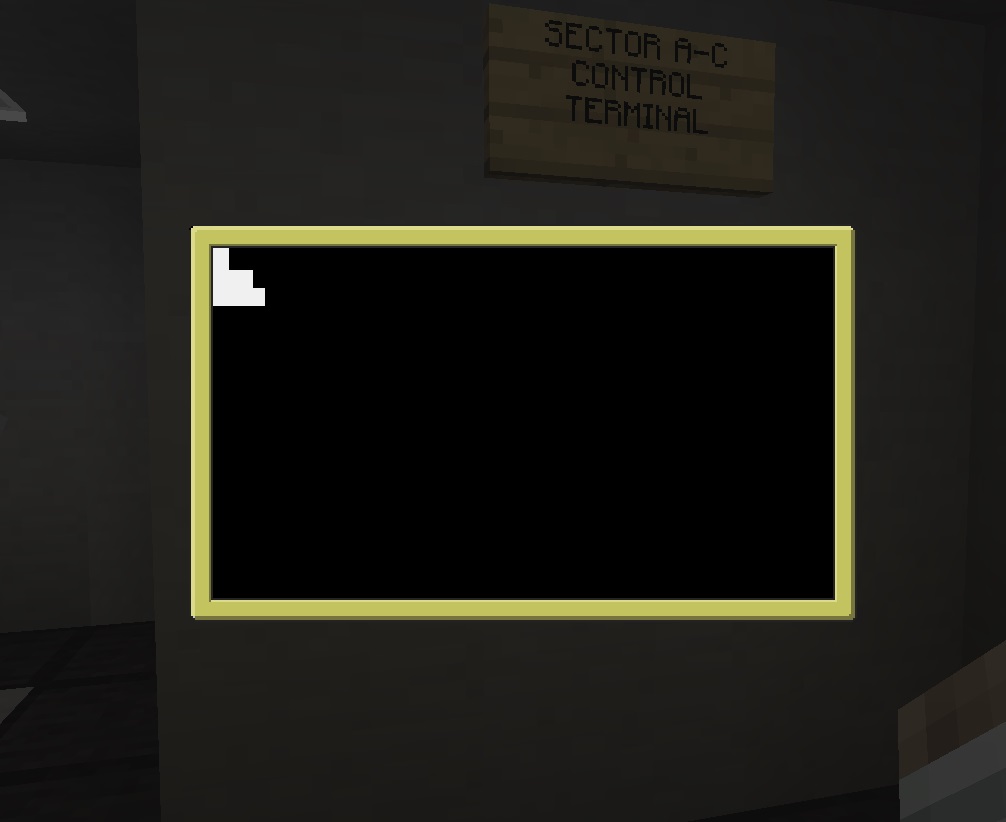
local Vector2 = {}
Vector2.new = function(xv,yv)
return {x = (xv or 0), y = (yv or 0)}
end
function win.drawBox(pos, size, c)
local xp = pos.x
local yp = pos.y
local xs = size.x
local ys = size.y
local bgc = termbgc
for currentX = xp, xs do
local currentY = yp
paintutils.drawPixel(
currentX,
currentY,
c
)
for cy = currentY, ys do
paintutils.drawPixel(
currentX,
cy,
c
)
end
end
return {
bgc,
xp,
yp,
xs,
ys,
hide = function(this)
for currentX = this.xp, this.xs do
local currentY = this.yp
paintutils.drawPixel(
currentX,
currentY,
bgc
)
for cy = currentY, this.ys do
paintutils.drawPixel(
currentX,
this.cy,
bgc
)
end
end
}
end
local bp = Vector2.new(1,1)
local bs = Vector2.new(5,5)
win.drawBox(bp, bs, colors.white)