Second, I been working on a little project on my own server. This project was going to use CC and AE ME system. Basically, program will ask the user for their username/password to gain access to their ME Drive (it will have their own ME storage disks that they will need to make themselves). The login computer will then send a message to the computer that will then run the program to send a redstone signal to the dark ME cable to allow that ME Drive to be apart of the ME system.
Here is the picture of a test setup:
Spoiler
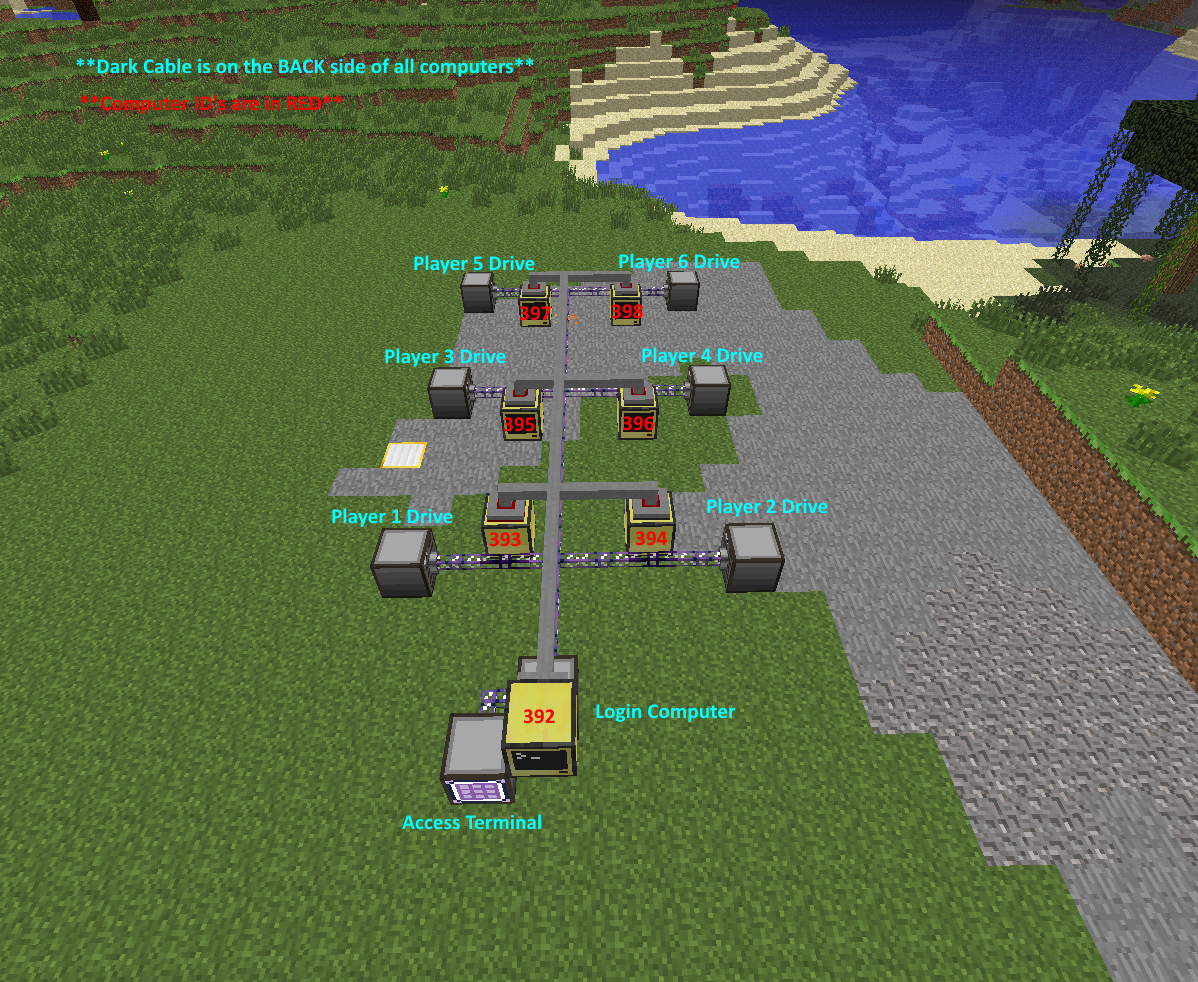
How this (should) work:
The player will need to login (of course!) and the program on the Login Computer will then check the table for the verification. If true, it will then send a message to the computer with the ID (username) the player logged in as. First, the message will send a command to run the "On" program to turn on the redstone output to turn the dark cable on (duh!) The program will then wait for a set time (default of 60 seconds) then will automatically send the command to run the "Off" program to the same computer to turn off the dark cable.
What is going wrong:
The login displays perfectly. Asks for the username (aka ID) and password. After I type in the username 393 then the password player1, the program says the welcome message and sleeps for 2 secs like i coded, but then displays the "error" message and quits the program. I'm not sure if the problem is from the table (i did a quick google on the setup) or the "if" statements (i wrote them myself, still learning about the more complex setups of theses like this one)
I thought about not doing a table setup to store the username and password so later i can add a "Change Password" option to the program in case a player gave the password out to others.
This is my code as of right now.
Spoiler
local uTable = {
393 = "player1",
394 = "player2",
395 = "player3",
396 = "player4",
397 = "player5",
398 = "player6"
}
local i = 60
write("ID: ")
ID = read()
write("Password: ")
pass = read('*')
if uTable[ID] == pass then
print("Welcome! Here is your items, "..ID.."!")
sleep(2)
else --uTable[ID] ~= pass then
print"ID/Password Combination is wrong!"
sleep(5)
--os.shutdown()
end
if ID == 393 then
shell.run"clear"
rednet.open("back")
rednet.send(393, On)
print"Connection Open."
sleep(1)
repeat
shell.run("clear")
print("Conection Will close in "..i.." secs.")
sleep(1)
i = i - 1
until i == 0
shell.run("clear")
print"Connection Closed!"
rednet.send(393, Off)
os.shutdown()
elseif ID == 394 then
shell.run"clear"
rednet.open("back")
rednet.send(394, On)
print"Connection Open."
sleep(1)
repeat
shell.run("clear")
print("Conection Will close in "..i.." secs.")
sleep(1)
i = i - 1
until i == 0
shell.run("clear")
print"Connection Closed!"
rednet.send(394, Off)
os.shutdown()
elseif ID == 395 then
shell.run"clear"
rednet.open("back")
rednet.send(395, On)
print"Connection Open."
sleep(1)
repeat
shell.run("clear")
print("Conection Will close in "..i.." secs.")
sleep(1)
i = i - 1
until i == 0
shell.run("clear")
print"Connection Closed!"
rednet.send(395, Off)
os.shutdown()
elseif ID == 396 then
shell.run"clear"
rednet.open("back")
rednet.send(396, On)
print"Connection Open."
sleep(1)
repeat
shell.run("clear")
print("Conection Will close in "..i.." secs.")
sleep(1)
i = i - 1
until i == 0
shell.run("clear")
print"Connection Closed!"
rednet.send(396, Off)
os.shutdown()
elseif ID == 397 then
shell.run"clear"
rednet.open("back")
rednet.send(397, On)
print"Connection Open."
sleep(1)
repeat
shell.run("clear")
print("Conection Will close in "..i.." secs.")
sleep(1)
i = i - 1
until i == 0
shell.run("clear")
print"Connection Closed!"
rednet.send(397, Off)
os.shutdown()
elseif ID == 398 then
shell.run"clear"
rednet.open("back")
rednet.send(398, On)
print"Connection Open."
sleep(1)
repeat
shell.run("clear")
print("Connection Will close in "..i.." secs.")
sleep(1)
i = i - 1
until i == 0
shell.run("clear")
print"Connection Closed!"
rednet.send(398, Off)
os.shutdown()
else
print"Error!"
end
FYI: i checked the message sending code with a program that will send the message with a "computerID" var equal to the network and it went to the right computer and was able to turn on the dark cabel and then 60 seconds later, turn it off. So i know that portion is good.