I'm having a real hard time with understand ccsensors. This is the code from the aforementioned website,
os.unloadAPI("/rom/apis/sensors")
os.loadAPI("/rom/apis/sensors")
local controllerSide = sensors.getController()
local sensorsList = sensors.getSensors( controllerSide )
for _,sensor in pairs( sensors.getSensors( controllerSide ) ) do
for _,probe in pairs( sensors.getProbes( controllerSide, sensor ) ) do
for _, target in pairs( sensors.getAvailableTargetsforProbe( controllerSide, sensor, probe ) ) do
for k, data in pairs( sensors.getSensorReadingAsDict( controllerSide, sensor, target, probe ) ) do
print( k.." : "..tostring( data ) )
end
end
end
end
This is the output.
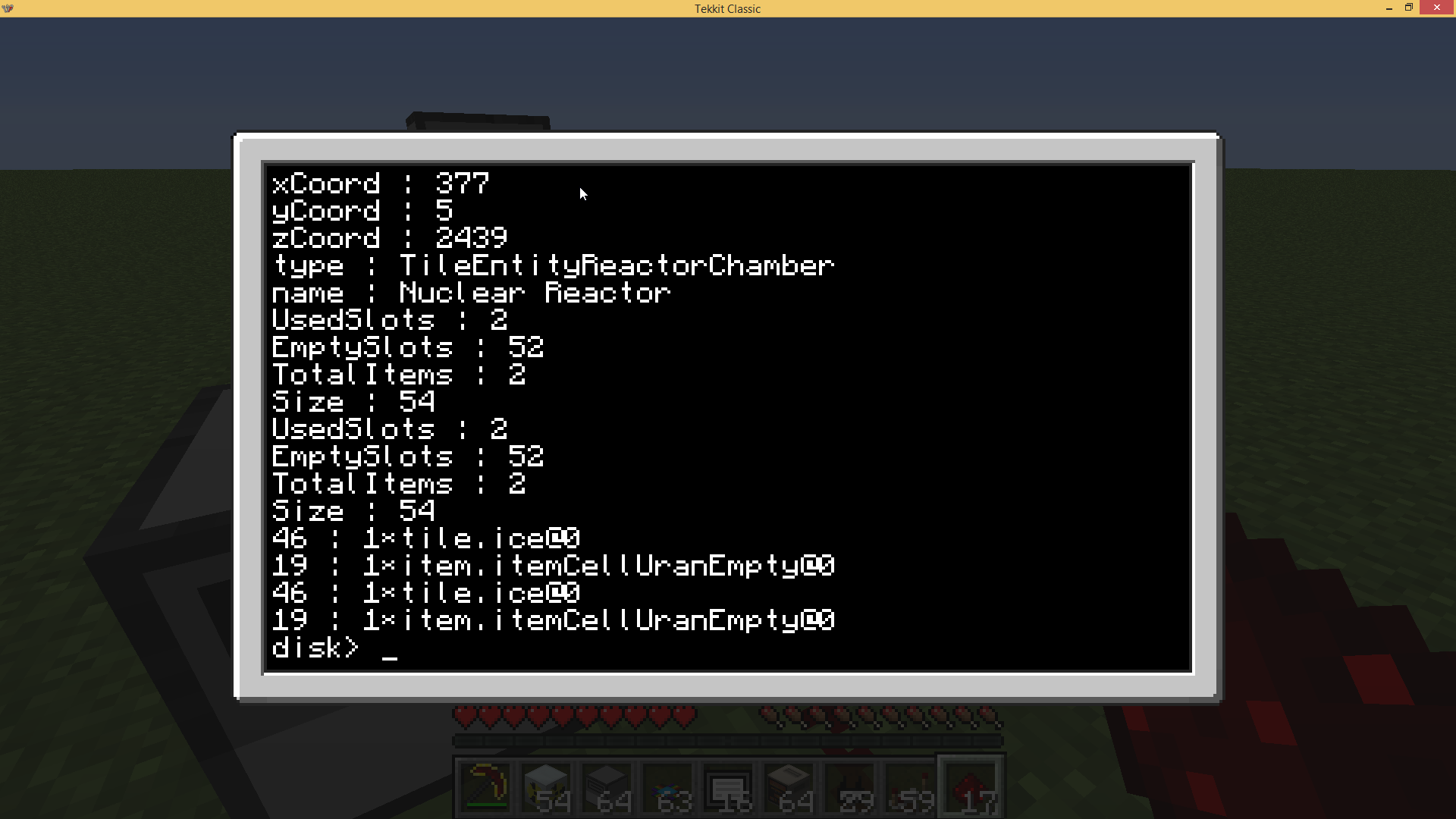
I don't understand how to output just the data for the inventory as it's giving me a whole slew of other information.
edit: I've narrowed down the code a bit and put in solid variables for the first two for loops. It's now only giving me inventory information.
os.unloadAPI("/rom/apis/sensors")
os.loadAPI("/rom/apis/sensors")
local controllerSide = sensors.getController()
local sensor = "Sensor"
local probes = "InventoryContent"
for _, target in pairs( sensors.getAvailableTargetsforProbe( controllerSide, sensor, probes ) ) do
for k, data in pairs( sensors.getSensorReadingAsDict( controllerSide, sensor, target, probes ) ) do
print( k.." : "..tostring( data ) )
end
end
The last issue to tackle is I don't understand the for loops. I tried setting a variable equal to
sensors.getAvailableTargetsforProbe( controllerSide, sensor, probes )
and then replacing that variable with "target" in
sensors.getSensorReadingAsDict( controllerSide, sensor, **INSERT VARIABLE**, probes )
and it outputs nothing.'
Edit:
I tried just outputting the value of the target function to see what it outputs and it just gives me "table: *insert random alphanumeric stuff, 7-8 characters long.*"
os.unloadAPI("/rom/apis/sensors")
os.loadAPI("/rom/apis/sensors")
local controllerSide = sensors.getController()
local sensor = "Sensor"
local probes = "InventoryContent"
local target = sensors.getAvailableTargetsforProbe( controllerSide, sensor, probes )
print( target )