http://pastebin.com/msVDnu6e
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
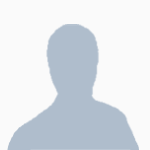
loop getting skipped?
Started by RoyalKingZB, 14 October 2014 - 09:18 PMPosted 14 October 2014 - 11:18 PM
One of my loops in my program is getting skipped. Its moving on to the next thing in the program and completely skipping the "all" loop in this program:
http://pastebin.com/msVDnu6e
http://pastebin.com/msVDnu6e
Posted 14 October 2014 - 11:41 PM
I assume you are talking about this:
I see no reason for it to do so within the code. Although I do see a *lot* of useless code that could fail and does not have failure prevention…
EG:
And should be this:
Edit: Fixed mispelling of "turlte"
for all = 1, y do
smartForward()
torch()
dig()
torch()
end
Your saying, the turtle turns when it starts, and continues in that direction for 1/2 of what you entered?I see no reason for it to do so within the code. Although I do see a *lot* of useless code that could fail and does not have failure prevention…
EG:
local function dig()
turtle.dig() --1
turtle.digUp()
turtle.placeDown()
turtle.forward()
turtle.digUp()
turtle.placeDown()
turtle.dig() --2
turtle.digUp()
turtle.placeDown()
turtle.forward()
turtle.digUp()
turtle.placeDown()
turtle.dig() --3
turtle.digUp()
turtle.placeDown()
turtle.forward()
turtle.digUp()
turtle.placeDown()
turtle.dig() --4
turtle.digUp()
turtle.placeDown()
turtle.forward()
turtle.digUp()
turtle.placeDown()
turtle.dig() --5
turtle.digUp()
turtle.placeDown()
turtle.forward()
turtle.digUp()
turtle.placeDown()
turtle.dig() --6
turtle.digUp()
turtle.placeDown()
turtle.forward()
turtle.digUp()
turtle.placeDown()
turtle.dig() --7
turtle.digUp()
turtle.placeDown()
turtle.forward()
turtle.digUp()
turtle.placeDown()
turtle.dig() --8
turtle.digUp()
turtle.placeDown()
turtle.forward()
turtle.digUp()
turtle.placeDown()
end
Is literally this:
local function dig()
for i = 1, 8 do
turtle.dig()
turtle.digUp()
turtle.placeDown()
turtle.forward()
--#why do you even have the rest of this?? it does nothing!
digUp()
turtle.placeDown()
forward()
end
end
And should be this:
local function dig()
for i = 1, 8 do
repeat until not turtle.digUp() --#dig up until nothing above
turtle.placeDown() --#attempt to place a block below
while not turtle.forward() do --#while it cant move, dig the block in front (fixes gravel/sand issue)
turtle.dig()
end
end
end
Edit: Fixed mispelling of "turlte"
Edited on 14 October 2014 - 09:42 PM
Posted 15 October 2014 - 12:05 AM
Yeah I didnt realize I could loop it until I already posted this Lol will that fix the turning around at the start?
And im just starting programming in computercraft so dont give me such a hard time :/
And im just starting programming in computercraft so dont give me such a hard time :/
Posted 15 October 2014 - 12:07 AM
It's worth noting that line 92:
… will set y to a number from 0 to 7 (whatever the remainder of x divided by 8 is). If you wanted to set y to x divided by 8, you'd do:
math.floor() rounds the result down; you could alternatively use math.ceiling() to round up.
If a "for" loop's target is less than its starting point, it won't run at all. Say you have:
… and y is 0; in such a case, "all" will start at 1, will already exceed y, and so the loop's content outright isn't executed.
y = x % 8
… will set y to a number from 0 to 7 (whatever the remainder of x divided by 8 is). If you wanted to set y to x divided by 8, you'd do:
y = math.floor(x / 8)
math.floor() rounds the result down; you could alternatively use math.ceiling() to round up.
If a "for" loop's target is less than its starting point, it won't run at all. Say you have:
for all = 1, y do
… and y is 0; in such a case, "all" will start at 1, will already exceed y, and so the loop's content outright isn't executed.
Posted 15 October 2014 - 12:27 AM
Ok so now I took out the x % 8 and the whole y value and it started working but now it goes 8x the distance I put in. How do I fix that? Updated pastebin:
http://pastebin.com/c2vq2S5p
Oh ok thank you ill see if it works.
http://pastebin.com/c2vq2S5p
Oh ok thank you ill see if it works.
Posted 15 October 2014 - 12:49 AM
In the "dig" function, you move forward 8 times. You then proceed to call dig x amount of times. This results in the turtle moving 8 times x times. It would be easily fixed by using x / 8 instead of x, OR removing the for loop inside dig().
Posted 15 October 2014 - 12:54 AM
Ok so now everything is working perfectly except when it comes back to the chest. It either doesnt go far enough or it goes past the chest. How do I make that work?
Posted 15 October 2014 - 01:06 AM
You might want to know, I'm not sure which suggestion you took, but if you used x / 8, the turtle actually moves:
math.floor( x / 8 ) * 8
Take for example 10:
math.floor( 10 / 8 ) * 8
1 * 8
8
Therefor, if the turtle used x to get back, it would overshoot the chest.
math.floor( x / 8 ) * 8
Take for example 10:
math.floor( 10 / 8 ) * 8
1 * 8
8
Therefor, if the turtle used x to get back, it would overshoot the chest.
Posted 15 October 2014 - 02:07 AM
So how do I get it to go the right amount of blocks on its way back? Im sorry im asking so many questions. Im desperate to get this working. Ive been working on it for forever >.< I also need it to go forward the right amount of blocks so how do I do that since "y = math.floor(x / 8)" doesnt work…right?
Posted 15 October 2014 - 02:30 AM
So how do I get it to go the right amount of blocks on its way back? Im sorry im asking so many questions. Im desperate to get this working. Ive been working on it for forever >.< I also need it to go forward the right amount of blocks so how do I do that since "y = math.floor(x / 8)" doesnt work…right?
If you are insisting on making it go in intervals of 8 then changing your loop to doing it once and multiplying the input by 8 would be better. If you use math.floor you lose a few blocks if you use math.ceil you gain a few so neither will be exact.
Otherwise it would be better to make it only do it once in the loop since there is no point in making it do it 8 times, and also divide by 8. Save a step and make it do it once in the function and remove the divide by 8.
If you do not want to do that you could just use the math.floor thing to figure out how far to go back. As in king's example just do x-(x%8) if I am not mistaken that will equal the same as math.floor(x)*8 which is the number of blocks the turtle travels. This is assuming of course that x is the input.
Edited on 15 October 2014 - 12:37 AM
Posted 15 October 2014 - 02:35 AM
It's math.ceil, not math.ceiling, FYI.
Posted 15 October 2014 - 04:19 AM
Can someone just tell me exactly what to put the x and y value as and where to put the two values? Trying to get this to work is seriously pissing me off. Im not understanding what you guys are saying. Or just modify this pastebin link and send the finished product back to me please.
http://pastebin.com/AXdDivPQ
http://pastebin.com/AXdDivPQ
Posted 15 October 2014 - 07:43 AM
Posted 15 October 2014 - 07:52 PM
It didnt work at all. It literally broke one block and stopped…
Posted 15 October 2014 - 08:13 PM
I really need help getting this to work :(/>
Posted 15 October 2014 - 08:19 PM
You posted your last ask literally 20 minutes before, sometimes things take time. (in other words, i'm looking at it and attempting to help you, be patient.)
Edited on 15 October 2014 - 06:20 PM
Posted 15 October 2014 - 08:22 PM
Ive been working on this program literally non stop since last saterday. I really want this to work. I just want to get done with it so I can get it working for peat sake :(/>
Posted 15 October 2014 - 08:26 PM
That doesn't mean be impatient, it makes people not want to help you. i'm currently looking at your code and trying to find the fix, and as i've not really used the turtle api that much, it's going to take me some time.
Your turtle has fuel right?
Also can you give me an in dept look as to what the turtle should be doing, every step, at what point should x happen, what point should this, what point should that.
Your turtle has fuel right?
Also can you give me an in dept look as to what the turtle should be doing, every step, at what point should x happen, what point should this, what point should that.
Edited on 15 October 2014 - 06:48 PM
Posted 15 October 2014 - 09:11 PM
Ok so I want it to start out placing a torch then after every 8 blocks, I want it to place a torch.. I also want it to not have trouble with sand or gravel. I also want it to place blocks underneith it as it goes. And finally, I want it to come back to the chest and drop off its goodies and then move on to the next tunnel with a 2 block space inbetween each tunnel. Im really having trouble getting it to come back to the chest. It either doesnt go far enough to get to the chest or it overshoots it and I dont know why it does that.
Thank you for trying to help.
Oh and yes it has fuel. I use like a full stack of coal on it Lol
Thank you for trying to help.
Oh and yes it has fuel. I use like a full stack of coal on it Lol
Posted 16 October 2014 - 10:36 AM
Sorry for not getting back to you, I sadly didn't get enough sleep the night before and fell asleep waiting for your reply. As for the last part of your troubles
All right so i believe i got the code working for you, it does not go back to the chest after the first one, i'll leave that up to you, however it should place a torch after every 8 blocks, independently of what number you start on, and it goes back the same amount it went in, mind you it does however mine an additional block at the end if the final number is a multiple of 8.
The torches are placed above the turtle on the wall, rather than in sockets in the wall. (mostly because the light doesn't reach as far with blocks obstructing it.)
How the torch placing after 8 blocks moved is simple, I checked to see if the amount of blocks we moved divided by eight had no remainder, and if it didn't, then we place a torch.
That is caused by you using math.floor (which is a bad idea in this case)Im really having trouble getting it to come back to the chest. It either doesnt go far enough to get to the chest or it overshoots it and I dont know why it does that.
All right so i believe i got the code working for you, it does not go back to the chest after the first one, i'll leave that up to you, however it should place a torch after every 8 blocks, independently of what number you start on, and it goes back the same amount it went in, mind you it does however mine an additional block at the end if the final number is a multiple of 8.
The torches are placed above the turtle on the wall, rather than in sockets in the wall. (mostly because the light doesn't reach as far with blocks obstructing it.)
How the torch placing after 8 blocks moved is simple, I checked to see if the amount of blocks we moved divided by eight had no remainder, and if it didn't, then we place a torch.
if z % 8 == 0 then
if z (in this case the amount of blocks moved) divided by eight has no remainder. In other words, if z is a multiple of 8 and a whole number.Spoiler
term.clear()
term.setCursorPos(1,1)
local z = 0
local function smartForward(v)
local c = turtle.forward()
while not c do
if turtle.detect() then
turtle.dig()
c = turtle.forward()
elseif turtle.attack() then
print("Mob detected, attacking")
c = turtle.forward()
end
end
if not v then
turtle.digUp()
z = z + 1
end
end
local function smartTorch()
while not turtle.placeUp() do
if turtle.detectUp() then
turtle.digUp()
end
end
end
local function torch()
if z % 8 == 0 then
smartForward(1)
turtle.digUp()
turtle.back()
turtle.turnLeft()
turtle.placeDown()
turtle.select(16)
turtle.digUp()
smartTorch()
turtle.turnRight()
turtle.select(1)
end
end
print("How Far?")
x = read()
y = tonumber(x)
while true do
for all = 1, y do
smartForward()
torch()
end
turtle.turnLeft()
turtle.turnLeft()
for i = 1, y do
smartForward(1)
end
turtle.turnRight()
turtle.drop()
turtle.turnLeft()
turtle.turnLeft()
smartForward()
smartForward()
smartForward()
turtle.turnLeft()
z = 0
end
Edited on 16 October 2014 - 09:40 AM
Posted 16 October 2014 - 08:00 PM
Ok Ill try it out and get back to you. Sorry it took soxlong to write this. Im in 10th grade so I had school :P/> Thats why im not that good at computercraft stuff Lol
Posted 16 October 2014 - 09:57 PM
It gave me an error saying:
bios:339: [string "miner1"]:26: '<eof>' expected
Hey, text me so we can talk a lot faster: [Removed. -L]
bios:339: [string "miner1"]:26: '<eof>' expected
Hey, text me so we can talk a lot faster: [Removed. -L]
Edited on 16 October 2014 - 08:14 PM
Posted 16 October 2014 - 10:09 PM
The code i put would not error, you need to make sure it's the same as what i put.
Posted 16 October 2014 - 10:16 PM
Don't post your phone number in a public forum, it's generally a very bad idea. Be careful who you give personal information out to, and always make sure to give it to the intended recipient in the most private way reasonable. In this case, if you really want to give him your phone number (which I am not saying is a good idea), you should use the PM system.
Posted 16 October 2014 - 10:32 PM
Did you test it?
Posted 16 October 2014 - 10:40 PM
Is the extra spaces in that one function nessecery cause I didnt put the extra spaces..
Posted 16 October 2014 - 10:43 PM
Yes i have tested it, and yes it does work. http://pastebin.com/q6Jxuy3C
Posted 16 October 2014 - 10:51 PM
Oh ok Lol I was just about to ask for the pastebin link Lol thanks
Posted 17 October 2014 - 12:36 AM
Lol I dont know why but it just breaks one block and thats it. Everytime i place one down in front of it, it breaks it but thats all it does O.o
Oh. It might be because Im using minecraft 1.6.4…
Oh. It might be because Im using minecraft 1.6.4…
Posted 17 October 2014 - 12:51 AM
I'm on minecraft 1.6.4 what are you inputting into the turtle?
Posted 17 October 2014 - 12:58 AM
8
Posted 17 October 2014 - 12:58 AM
Is it fueled?
Posted 17 October 2014 - 01:06 AM
:o/> Im so freaken stupid LMFAO I put a whole stack of coal in it so I thought I was good for a while Ugh Im stupid -.- Im scarce on coal right now so I gotta go mining >.<
Posted 17 October 2014 - 01:31 AM
It works great except you forgot to make it place blocks under it but otherwise, its perfect! Thank you so much!!!! :D/> Can you teach me how to write code like that??? :)/>
Oh wait nevermind Lol I didnt put blocks in it Lol it works great! Lol :D/>
Oh wait nevermind Lol I didnt put blocks in it Lol it works great! Lol :D/>
Posted 17 October 2014 - 01:49 AM
Oh nevermind again lol it doesnt Lol but thank you so much! Youre the best :)/>
Posted 17 October 2014 - 02:06 AM
Lol btw, you put a v when you put local function smartForward(v)
Posted 17 October 2014 - 02:22 AM
Yes i did, i used it as a check for finding if i'm just wanting to move forward and not dig up. I'm just using it as a variable that i set an arbitrary value to and if a value has been set then every time i move forward, dig up, otherwise if it doesn't have a value, don't dig up.
Posted 17 October 2014 - 08:42 PM
Oh well I kinda modified it to my liking and made it place a block before it puts a torch in case it doesnt have one but when it mines a tunnel, comes back and starts the second tunnel, it doesnt place blocks before placing the torch. Why?
http://pastebin.com/cvdwUdyb
http://pastebin.com/cvdwUdyb
Posted 17 October 2014 - 09:49 PM
All right so first thing when looking at the code, your smartforwardno function, you don't need s, i only used v so i could use it again to not break the torch on the way back, so you don't need that. (although with it, it doesn't matter), as for the not placing blocks, you're keeping the turtle.select() on slot 15 rather than resetting it back to slot 1. just put turtle.select(1) at the end of the while loop
Posted 17 October 2014 - 10:00 PM
Ohhhh Ok thank you so much.
Posted 17 October 2014 - 10:07 PM
It works perfectly now! Finally! Thank you so much!!!
Posted 17 October 2014 - 10:14 PM
Oh wait :(/> on the 3rd tunnel, it went past the chest. Can you help again? Sorry :(/>
http://pastebin.com/2vWYPhGv
http://pastebin.com/2vWYPhGv