41 posts
Location
Everywhere
Posted 11 November 2014 - 12:08 PM
Hey,So i'm trying to send a serialized table over rednet, and write it to a file on the other end. I have this working, but what I can't figure out how to do is read from the table once unserialized. In the file, it looks like this:{1.0=Bob, 2.0=16, 3.0=Male}
I'm trying to get, say, entry 1 in the table (bob). Any ideas on how I could do this?
7083 posts
Location
Tasmania (AU)
Posted 11 November 2014 - 12:16 PM
Let's say you assigned your unserialised table to "myTable" - to get at index 1, you'd refer to myTable[1]. Eg:
print(myTable[1])
41 posts
Location
Everywhere
Posted 11 November 2014 - 12:23 PM
Let's say you assigned your unserialised table to "myTable" - to get at index 1, you'd refer to myTable[1]. Eg:
print(myTable[1])
f = fs.open("2", "r")
myTable = f.readLine()
f.close()
print(myTable) -- prints the table in 1 line
print(myTable[1]) -- prints a blak line
Just prints a blank line.
1847 posts
Location
/home/dannysmc95
Posted 11 November 2014 - 12:24 PM
Yes as said above:
You are using an array, which means to get the values in the table it's ordered by 1 and upwards:
so myTable[1] is Bob, myTable[2] is 16 and myTable[3] is male.
if you want to print all values onto a in a list going down use:
for _, v in ipairs(myTable) do
print(v)
end
Let's say you assigned your unserialised table to "myTable" - to get at index 1, you'd refer to myTable[1]. Eg:
print(myTable[1])
f = fs.open("2", "r")
myTable = f.readLine()
f.close()
print(myTable) -- prints the table in 1 line
print(myTable[1]) -- prints a blak line
Just prints a blank line.
This is because you haven't made the text a string…
This should be:
{1.0="Bob", 2.0=16, 3.0="Male"}
7083 posts
Location
Tasmania (AU)
Posted 11 November 2014 - 12:28 PM
If print(myTable) "prints the table in one line", then I'm guessing you haven't actually unserialised the table:
myTable = textutils.unserialise(myTable)
Note that unless you're using a very old version of ComputerCraft, there's no need to serialise it in the first place unless you want to write it to disk. Modem/rednet transmissions will deal with just fine in an unserialised state.
41 posts
Location
Everywhere
Posted 11 November 2014 - 12:53 PM
-snip-
-snip-
Forgive me if I am being ignorantly stupid here, but I took your advice and just straight out sent the table over rednet, then used
for _, v in ipairs(myTable) do print(v)end to print it.
It just prints:
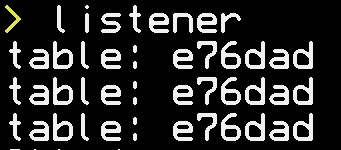
1847 posts
Location
/home/dannysmc95
Posted 11 November 2014 - 01:11 PM
-snip-
-snip-
Forgive me if I am being ignorantly stupid here, but I took your advice and just straight out sent the table over rednet, then used
for _, v in ipairs(myTable) do print(v)endto print it.
It just prints:
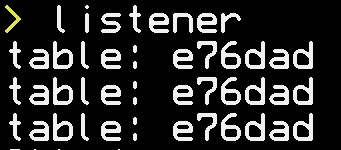
This means that you are storing it as a table inside a table, what does the table look like at the moment?
41 posts
Location
Everywhere
Posted 11 November 2014 - 01:19 PM
-snip-
{1.0=bob, 2.0=16, 3.0=male}
I stopped printing it, it now just writes it to a file as soon as it is received. That is what it looks like in the file. All I want is to be able to extract each element in the table, but I get blank when I try.
Thanks for the help, btw.
1080 posts
Location
In the Matrix
Posted 11 November 2014 - 01:27 PM
What is your current code? It might be a problem on how you're sending/receiving.
1847 posts
Location
/home/dannysmc95
Posted 11 November 2014 - 02:20 PM
-snip-
{1.0=bob, 2.0=16, 3.0=male}
I stopped printing it, it now just writes it to a file as soon as it is received. That is what it looks like in the file. All I want is to be able to extract each element in the table, but I get blank when I try.
Thanks for the help, btw.
The text is not a string?:S
41 posts
Location
Everywhere
Posted 11 November 2014 - 03:13 PM
-snip-
{1.0=bob, 2.0=16, 3.0=male}
I stopped printing it, it now just writes it to a file as soon as it is received. That is what it looks like in the file. All I want is to be able to extract each element in the table, but I get blank when I try.
Thanks for the help, btw.
The text is not a string?:S
stringTable = tostring(myTable)?
1847 posts
Location
/home/dannysmc95
Posted 11 November 2014 - 03:18 PM
-snip-
{1.0=bob, 2.0=16, 3.0=male}
I stopped printing it, it now just writes it to a file as soon as it is received. That is what it looks like in the file. All I want is to be able to extract each element in the table, but I get blank when I try.
Thanks for the help, btw.
The text is not a string?:S
stringTable = tostring(myTable)?
Nope, the actual values aren't strings? Bob and "Bob" are very different things, the Bob needs to be "Bob", same with any other values that aren't numbers.
1080 posts
Location
In the Matrix
Posted 11 November 2014 - 03:31 PM
myTable = {"hello","hi","nooo"}
stringTable = textutils.serialize(myTable)
print(stringTable)
Prints out:
{
[1] = "hello",
[2] = "hi",
[3] = "nooo"
}
However using unserialize on the file's data will give you the normal table.
local tableString = "{ [1] = \"hello\", [2] = \"hi\", [3] = \"nooo\" }" --#Backslashes for escaping the quotes
local stringTable = textutils.unserialize(tableString)
print(stringTable[1])
Prints out:
hello
Edited on 11 November 2014 - 02:32 PM
41 posts
Location
Everywhere
Posted 11 November 2014 - 03:33 PM
-snip-
EDIT:
Must be an error in my syntax along the way, retyped it using your guys' first suggestions, works fine. Thanks for all the help.
Edited on 11 November 2014 - 03:04 PM