This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
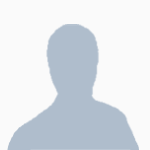
Iterations?
Started by lordofttude, 21 December 2014 - 08:58 PMPosted 21 December 2014 - 09:58 PM
If I had a program say "mine", how would I make it so that I could say "mine 10" to make that program iterate 10x, or "mine 2" to make the program iterate 2x and so on and so forth? Also How would I then make it display what iteration it was on? Thanks.
Posted 21 December 2014 - 10:09 PM
You could do this( I believe you meant getting arguments when running the program right?
If you want todo it by a read input or something you could do this
You should read about for loops here to get a better understanding.
Displaying what iteration it's on is simple
local args = { ... }
if #args < 1 then
error( "Usage: mine <n>", 0 )
end
local n = tonumber( args[1] )
for i = 1, n do
-- do stuff
end
by this example you could do "mine 10"If you want todo it by a read input or something you could do this
local function getArguments( str )
local arguments = {}
for word, _ in str:gmatch( "%S+" ) do
table.insert( arguments, word )
end
return arguments
end
local input = read()
local args = getArguments( input )
if #args < 2 then
error( "Usage: mine <n>", 0 )
end
if args[1] == "mine" then
local n = tonumber( args[2] )
for i = 1, n do
-- do stuff
end
end
You should read about for loops here to get a better understanding.
Displaying what iteration it's on is simple
--# The loop variable's name is 'i', which we can simply print
for i = 1, 10 do
print( i )
end
Edited on 21 December 2014 - 09:21 PM
Posted 21 December 2014 - 10:19 PM
Yes that is what I meant, thanks.You could do this( I believe you meant getting arguments when running the program right?by this example you could do "mine 10"local args = { ... } if #args < 1 then error( "Usage: mine <n>", 0 ) end local n = tonumber( args[1] ) for i = 1, n do -- do stuff end
If you want todo it by a read input or something you could do thislocal function getArguments( str ) local arguments = {} for word, _ in str:gmatch( "%S+" ) do table.insert( arguments, word ) end return arguments end local input = read() local args = getArguments( input ) if #args < 2 then error( "Usage: mine <n>", 0 ) end if args[1] == "mine" then local n = tonumber( args[2] ) for i = 1, n do -- do stuff end end
Posted 22 December 2014 - 11:12 PM
dont sapose u could pastebin the compleated code to save others some time who are trying t od this for the first time ever using computercraft:)
Posted 22 December 2014 - 11:14 PM
Do at least try to spell check what comes from your keyboard…dont sapose u could pastebin the compleated code to save others some time who are trying t od this for the first time ever using computercraft:)
Posted 22 December 2014 - 11:17 PM
There's so many spelling errors there O.ODo at least try to spell check what comes from your keyboard…
And for the one who asked for a pastebin link you can simply do it yourself.
Copy the code > Paste it onto pastebin
Posted 24 December 2014 - 09:30 PM
Hey, I was wondering how I could make it so if a computer receives a redstone signal on the bottom it would pause the program that is running until the signal turns off… I tried chucking an if not statement in but that isn't enough. Clearly I am very new to Lua and just programming as a whole, but can someone suggest something? Thanks.
Here is the code, pastebin get jjgfhxkT
Here is the code, pastebin get jjgfhxkT
local args = { .. }
if #args < 1 then
error( "Usage: frame <n>" , 0)
end
function moveFrame()
rs.setOutput("top", true)
sleep(0.5)
rs.setOutput("top, false)
end
function mine()
rs.setOutput("left", true)
sleep(6.5)
rs.setOutput("left", false)
end
local n = tonumber ( args[1] )
for i = 1, n do
move frame()
mine()
end
if not (rs.getInput("bottom")) then
moveFrame()
mine()
end
Edited on 24 December 2014 - 10:43 PM
Posted 24 December 2014 - 09:49 PM
You've more or less got the idea, but you need to put that check into your mining loop. Eg:
local args = { ... }
local n = tonumber ( args[1] )
if not n then error( "Usage: frame <n>" , 0) end
for i = 1, n do
while rs.getInput("bottom") do -- So long as redstone is on...
os.pullEvent("redstone") -- ... wait for redstone state changes.
end
rs.setOutput("top", true)
sleep(0.5)
rs.setOutput("top", false)
rs.setOutput("left", true)
sleep(6.5)
rs.setOutput("left", false)
end
Edited on 24 December 2014 - 08:52 PM
Posted 24 December 2014 - 10:01 PM
Thank you very much…..that makes sense.You've more or less got the idea, but you need to put that check into your mining loop. Eg:local args = { ... } local n = tonumber ( args[1] ) if not n then error( "Usage: frame <n>" , 0) end for i = 1, n do while rs.getInput("bottom") do -- So long as redstone is on... os.pullEvent("redstone") -- ... wait for redstone state changes. end rs.setOutput("top", true) sleep(0.5) rs.setOutput("top", false) rs.setOutput("left", true) sleep(6.5) rs.setOutput("left", false) end
Posted 24 December 2014 - 10:42 PM
Also, how would I make it print what iteration the program is on? As in if I said "frame 3" (frame being the name of the program) it would print 1 for the first iteration. Then as soon as the second iteration starts it would print 2, so on and so forth.You've more or less got the idea, but you need to put that check into your mining loop. Eg:local args = { ... } local n = tonumber ( args[1] ) if not n then error( "Usage: frame <n>" , 0) end for i = 1, n do while rs.getInput("bottom") do -- So long as redstone is on... os.pullEvent("redstone") -- ... wait for redstone state changes. end rs.setOutput("top", true) sleep(0.5) rs.setOutput("top", false) rs.setOutput("left", true) sleep(6.5) rs.setOutput("left", false) end
Someone told me it would be as simple as printing the variable "i" but that doesn't seem to work… it just lists 1 through 10.
Edit: Someone answered my question already :)/> thanks anyway.
Edited on 24 December 2014 - 10:43 PM
Posted 24 December 2014 - 10:50 PM
When I ask it to print i it just lists 1 through 10… do you know what I did wrong?You could do this( I believe you meant getting arguments when running the program right?by this example you could do "mine 10"local args = { ... } if #args < 1 then error( "Usage: mine <n>", 0 ) end local n = tonumber( args[1] ) for i = 1, n do -- do stuff end
If you want todo it by a read input or something you could do thislocal function getArguments( str ) local arguments = {} for word, _ in str:gmatch( "%S+" ) do table.insert( arguments, word ) end return arguments end local input = read() local args = getArguments( input ) if #args < 2 then error( "Usage: mine <n>", 0 ) end if args[1] == "mine" then local n = tonumber( args[2] ) for i = 1, n do -- do stuff end end
You should read about for loops here to get a better understanding.
Displaying what iteration it's on is simple--# The loop variable's name is 'i', which we can simply print for i = 1, 10 do print( i ) end
Posted 24 December 2014 - 11:16 PM
What did you expect it to do? You print the value of 'i' which is just a simple number. Also, please try to keep all problems with the same code in one thread.
Posted 24 December 2014 - 11:23 PM
I will keep all the problems with the code the same thread, thanks for telling me, but this thread wasn't originally about a problem, it was just a question on how to do something and the help I received was only used in writing a piece of code later. That said how would I print what iteration the program is on, as in iteration 1, iteration 2, iteration, 3?What did you expect it to do? You print the value of 'i' which is just a simple number. Also, please try to keep all problems with the same code in one thread.
Edited on 24 December 2014 - 10:23 PM
Posted 24 December 2014 - 11:27 PM
You could simply use the basic Lua operator '..' (concat):
print("iteration " .. i)
Posted 24 December 2014 - 11:32 PM
ThanksYou could simply use the basic Lua operator '..' (concat):print("iteration " .. i)
Posted 24 December 2014 - 11:41 PM
It sounds to me like we have a misunderstanding… What you want to do sounds like listing 1 through 10. Could you clarify what you mean?
Posted 24 December 2014 - 11:44 PM
Worth noting that the same discussion is going on in this thread.
Posted 24 December 2014 - 11:45 PM
With BB's code that would exactly be what you have to do. It would look like this:
As the 3 of 'frame 3' would get passed as a number as the end condition for the for loop, it would only iterate 3 times - not more.
local args = { ... }
local n = tonumber ( args[1] )
if not n then error( "Usage: frame <n>" , 0) end
for i = 1, n do
print( "Iteration " .. i .. "/" .. n )
while rs.getInput("bottom") do -- So long as redstone is on...
os.pullEvent("redstone") -- ... wait for redstone state changes.
end
rs.setOutput("top", true)
sleep(0.5)
rs.setOutput("top", false)
rs.setOutput("left", true)
sleep(6.5)
rs.setOutput("left", false)
end
As the 3 of 'frame 3' would get passed as a number as the end condition for the for loop, it would only iterate 3 times - not more.
Posted 24 December 2014 - 11:45 PM
Threads merged.
Posted 24 December 2014 - 11:52 PM
Yes, I thought that by printing "i" I could tell what iteration my program was on but that is not the case. Someone just clarified this for me though and this is what he said would work: print("iteration" .. i). Thanks for the help anyway :)/>.It sounds to me like we have a misunderstanding… What you want to do sounds like listing 1 through 10. Could you clarify what you mean?
Posted 25 December 2014 - 12:06 AM
ThanksWith BB's code that would exactly be what you have to do. It would look like this:local args = { ... } local n = tonumber ( args[1] ) if not n then error( "Usage: frame <n>" , 0) end for i = 1, n do print( "Iteration " .. i .. "/" .. n ) while rs.getInput("bottom") do -- So long as redstone is on... os.pullEvent("redstone") -- ... wait for redstone state changes. end rs.setOutput("top", true) sleep(0.5) rs.setOutput("top", false) rs.setOutput("left", true) sleep(6.5) rs.setOutput("left", false) end
As the 3 of 'frame 3' would get passed as a number as the end condition for the for loop, it would only iterate 3 times - not more.