Posted 02 January 2015 - 01:10 AM
the way messages gotten from the modem(API) is confusing me. when writing it to a monitor it is a table, but when printing it in the terminal it is a random string.
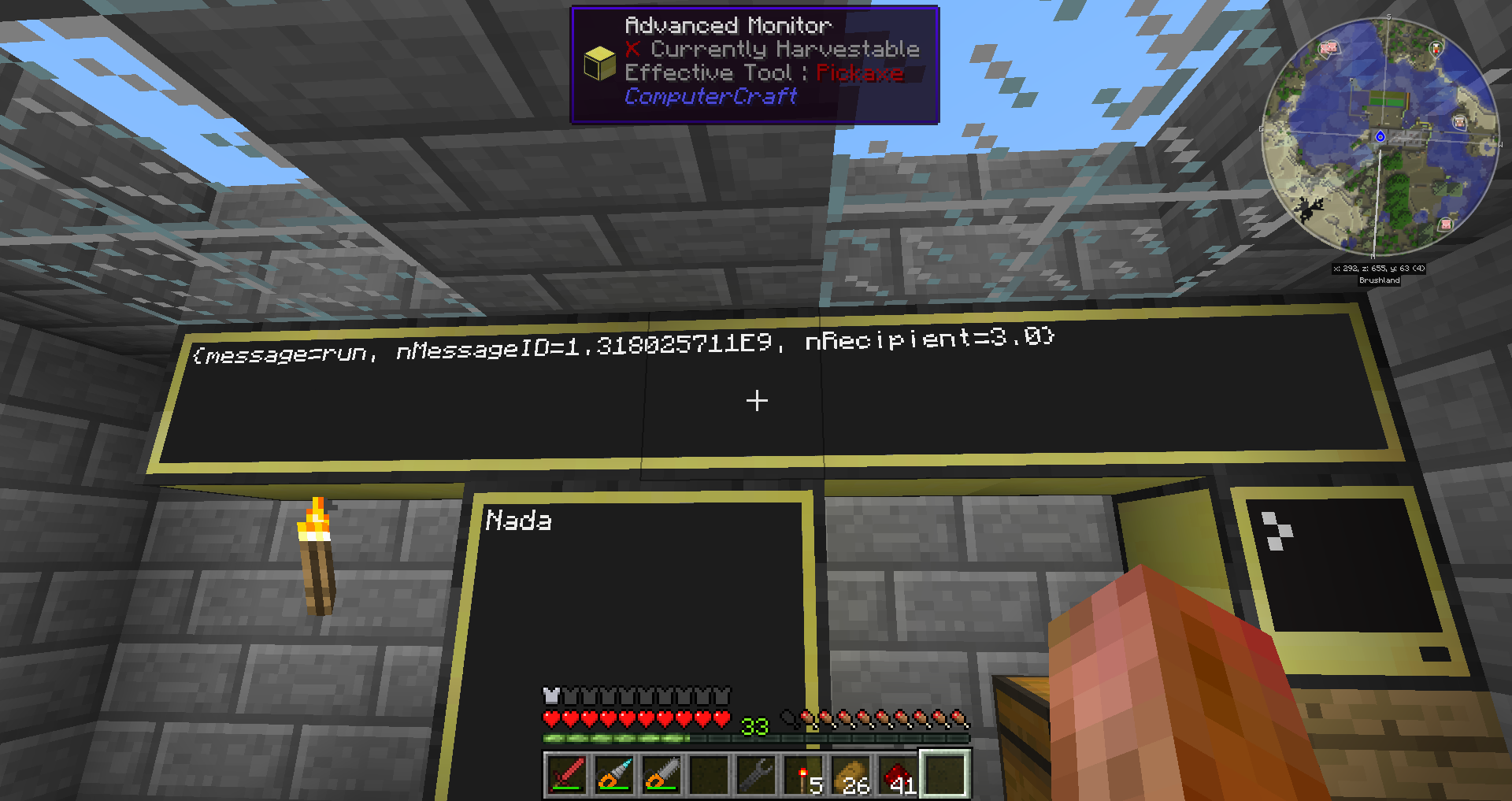
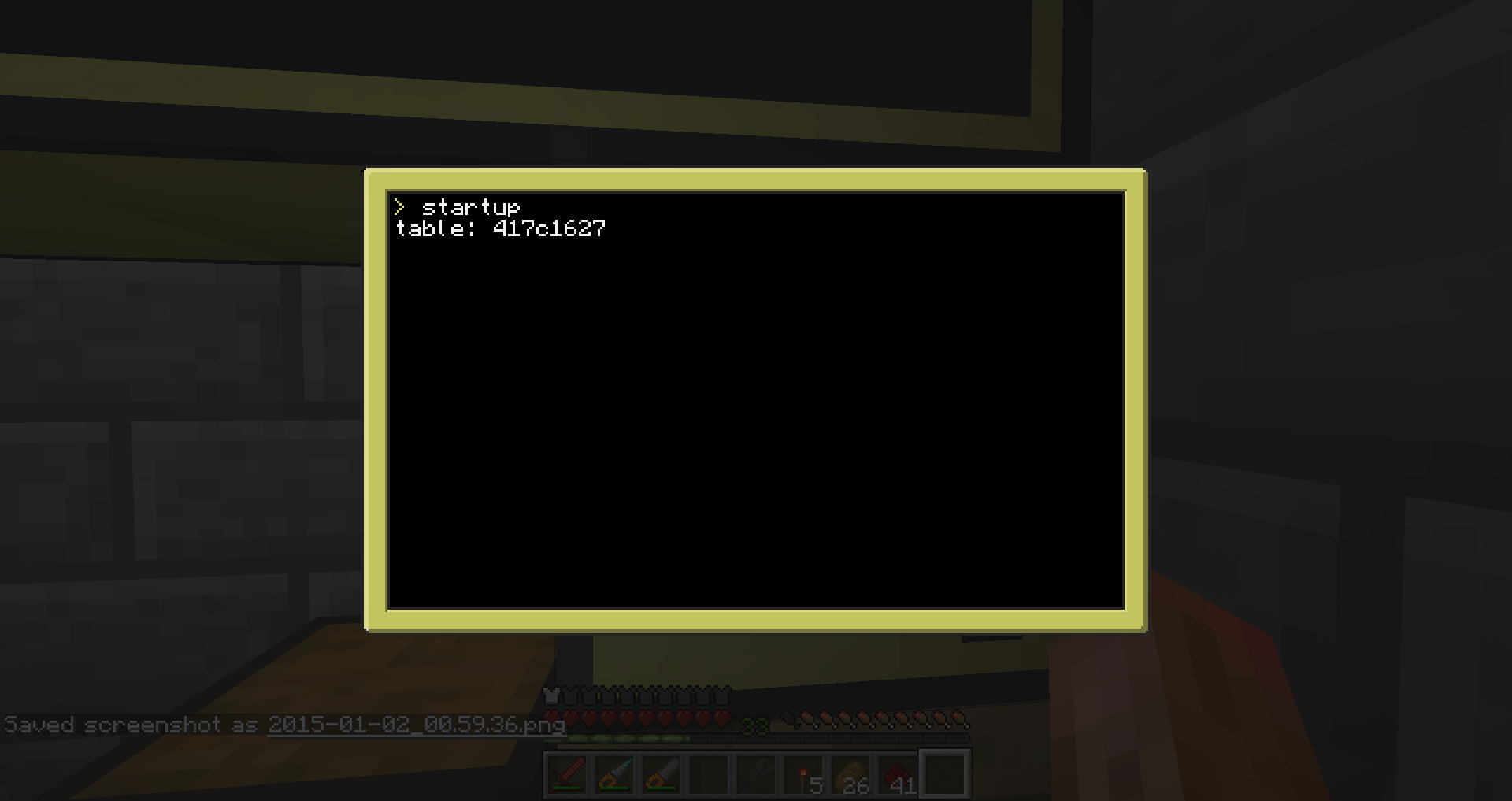
my code:
the program is meant to write all rednet communication from the network to the monitor. it kind of does so in it's current state, but i'd like it represented in a different way. could anyone explain why writing and printing the message gives two different results and how to get the message in a string?
message[0] and message[2] returns nil, which is very confusing to me. how can i get information out of "message"?
Answers and suggestions appreciated.
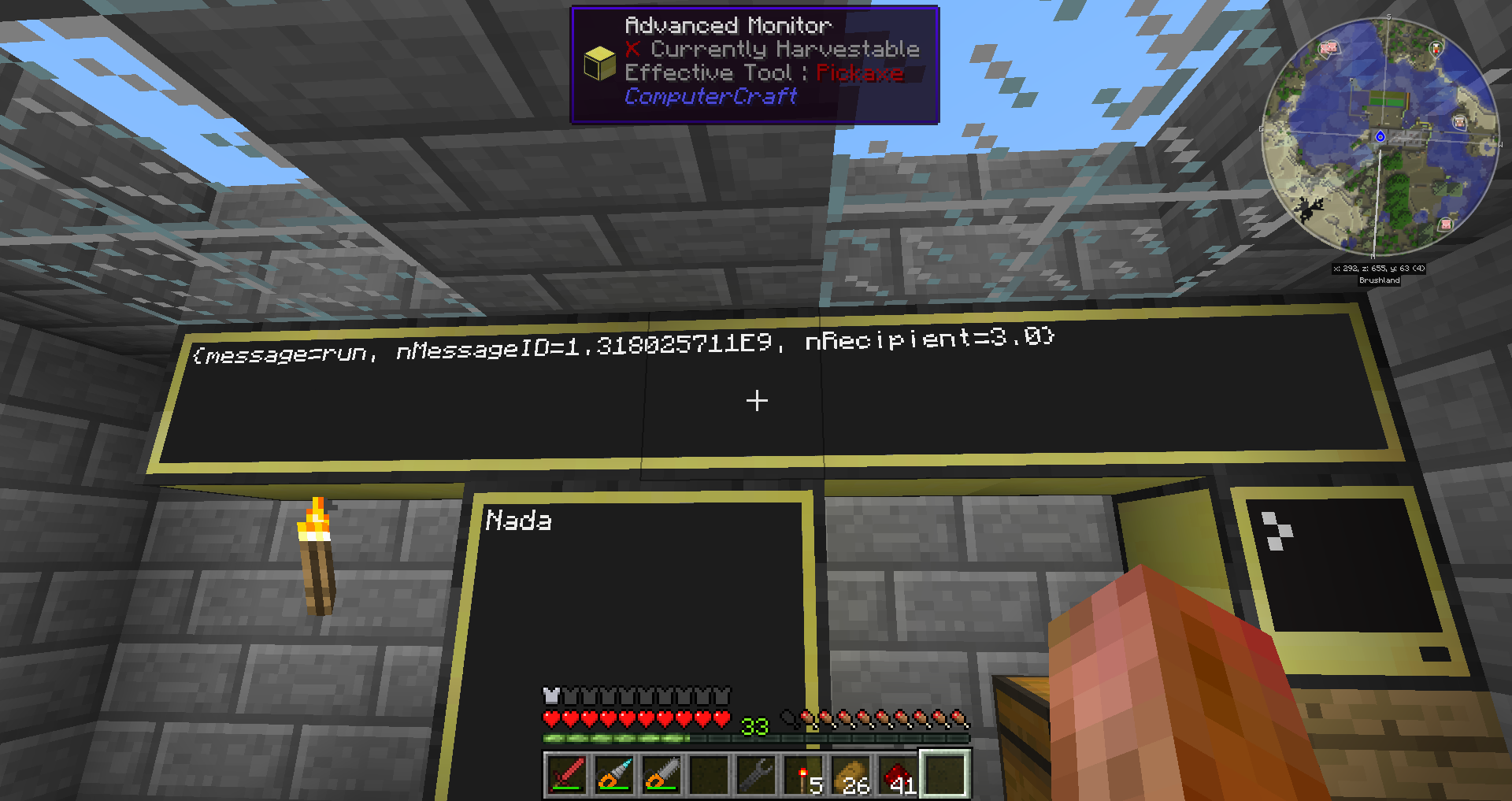
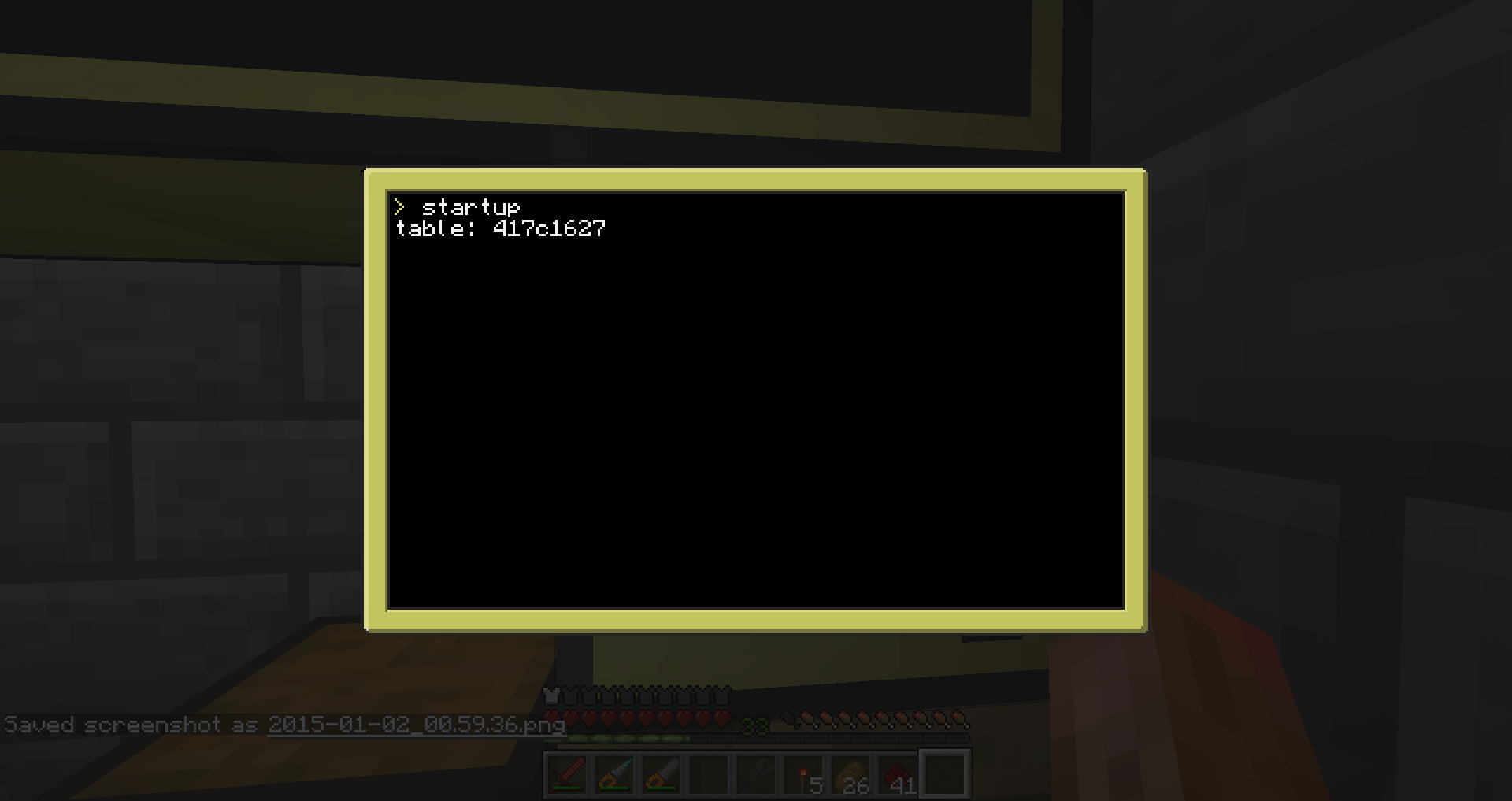
my code:
modem = peripheral.wrap("right")
modem.open(65533)
m = peripheral.wrap("top")
m.clear()
m.setCursorPos(1, 1)
function write(message)
print(message)
m.write(message)
--m.write("melding: "..message[0]..", "..
-- "mottaker:"..tostring(message[2]))
-- unmessage = textutils.unserialize(message)
-- print(unmessage)
x, y = m.getCursorPos()
if y == 1 then
m.setCursorPos(1, 2)
elseif y == 2 then
m.setCursorPos(1, 3)
elseif y == 3 then
m.setCursorPos(1, 4)
else
m.setCursorPos(1, 1)
end
end
while true do
event, modemSide, senderChannel,
senderID, message, distance = os.pullEvent("modem_message")
write(message)
end
the program is meant to write all rednet communication from the network to the monitor. it kind of does so in it's current state, but i'd like it represented in a different way. could anyone explain why writing and printing the message gives two different results and how to get the message in a string?
message[0] and message[2] returns nil, which is very confusing to me. how can i get information out of "message"?
Answers and suggestions appreciated.