This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
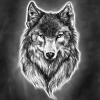
Lua 5.2 bit rotate
Started by toxicwolf, 18 June 2012 - 09:27 AMPosted 18 June 2012 - 11:27 AM
Hi, I've come across a need for the bit rotate functions from Lua 5.2's bit32 library. I've noticed that the ComputerCraft API bit already adds most of the bit32 functions, but I was wondering if there was anywhere where I could get hold of a rrotate function same as in the bit32 library.
Posted 18 June 2012 - 02:18 PM
Well you could try writing your own
something like
(probably horribly inefficient :(/>/>) )(and lrotate, not rrotate xD)
or using shift + or
now sure if these entirely work though :)/>/>
so something like that as rrotate?
something like
(probably horribly inefficient :(/>/>) )(and lrotate, not rrotate xD)
function bit32.lrotate(x,disp)
disp = disp % 32
local xArr = bit.tobits(x)
local nArr = bit.tobits(bit.blshift(x,disp))
for i=1,disp,1 do
nArr[i] = xArr[#xArr-disp+i]
end
return bit.tonumb(nArr)
end
or using shift + or
function bit32.lrotate2(x,disp)
disp = disp % 32
local mask = bit.blshift( bit.blshift(1,disp) - 1, 32-disp)
local bitex = bit.band(mask,x)
bitex = bit.brshift(bitex,32-disp)
return bit.bor(bit.blshift(x,disp),bitex)
end
now sure if these entirely work though :)/>/>
so something like that as rrotate?
function bit32.rrotate(x,disp)
disp = disp % 32
local mask = bit.blshift(1,disp) - 1
local bitex = bit.band(mask,x)
bitex = bit.blshift(bitex,32-disp)
return bit.bor(bit.brshift(x,disp),bitex)
end
Posted 18 June 2012 - 04:10 PM
One of these days I will get around to making a crypto peripheral, with native bit operations and stuff. But right now, I have lower hanging fruit to pick and such.
Posted 18 June 2012 - 04:22 PM
Well, to be honest I'm not entirely sure what it is meant to do, other than I need it :(/>/>Well you could try writing your own
I'm also not that good a programmer :)/>/>
The reason is that I found a SHA224/SHA256 implementation in Lua 5.2, however the only function that it requires that isn't available is the rrotate.
Posted 18 June 2012 - 04:37 PM
Heh, I was trying to implement the SAME THING the other day. A lshift moves every bit over to the left by one, and an rshift moves them all over to the right. These are easy to implement, since this just means dividing or multiplying by 2. The problem is, you "lose" 1 bit at the end. So, if I have a 8 bit number, and I shift it 8 times, I get all zeroes. But if I rotate it the same amount of times, I get back what I started. This is important to cryptography because, at an intuitive level, shifts make you lose data, so they cant be used for encryption, and they give you too many zeroes, so they are often unsuitable for hashing, because they make every result too similar.
Posted 18 June 2012 - 04:47 PM
I see, thanks :(/>/> I know how l/rshift works, cause that's what I used in my symmetric key encryption in WolfOS. I'm just not quite sure what rotating bits actually does compared to shifting the bits.Heh, I was trying to implement the SAME THING the other day. A lshift moves every bit over to the left by one, and an rshift moves them all over to the right. These are easy to implement, since this just means dividing or multiplying by 2. The problem is, you "lose" 1 bit at the end. So, if I have a 8 bit number, and I shift it 8 times, I get all zeroes. But if I rotate it the same amount of times, I get back what I started. This is important to cryptography because, at an intuitive level, shifts make you lose data, so they cant be used for encryption, and they give you too many zeroes, so they are often unsuitable for hashing, because they make every result too similar.
Also, ComputerCraft's native Bit API has bit shifting functions.
EDIT: After a bit of searching, I found this: https://bitbucket.or...4885805/bit.lua. Does anyone with more know-how think that it would be a suitable implementation of the bit32 library?
Just checked and the place where dan200 took the bit API from hasn't been updated since 2007, so perhaps this could be used to replace it altogether?
Posted 18 June 2012 - 07:06 PM
You can use this functions:
local function leftRotate(x, n)
return bit.bor(bit.blshift(x, n), bit.brshift(x, 32 - n))
end
local function rightRotate(x, n)
return bit.bor(bit.brshift(x, n), bit.blshift(x, 32 - n))
end
They work for 32-bit ints, but it can be changed to work with any size.I don't really like the and, or and xor functions, but it should work.EDIT: After a bit of searching, I found this: https://bitbucket.or...4885805/bit.lua. Does anyone with more know-how think that it would be a suitable implementation of the bit32 library?
Just checked and the place where dan200 took the bit API from hasn't been updated since 2007, so perhaps this could be used to replace it altogether?
Posted 18 June 2012 - 07:22 PM
Oh, okay, that's simple, thanks :(/>/> I will use the functions you suggested instead of the bit library implementation I found.You can use this functions:They work for 32-bit ints, but it can be changed to work with any size.local function leftRotate(x, n) return bit.bor(bit.blshift(x, n), bit.brshift(x, 32 - n)) end local function rightRotate(x, n) return bit.bor(bit.brshift(x, n), bit.blshift(x, 32 - n)) end
I don't really like the and, or and xor functions, but it should work.EDIT: After a bit of searching, I found this: https://bitbucket.or...4885805/bit.lua. Does anyone with more know-how think that it would be a suitable implementation of the bit32 library?
Just checked and the place where dan200 took the bit API from hasn't been updated since 2007, so perhaps this could be used to replace it altogether?
Posted 18 June 2012 - 07:47 PM
There might be some better/efficient way to do it, but it works. I actually use them for the same thing you need them, I already implemented md5, sha256 and sha224. So bad I didn't find that implementation before doing it myself :(/>/>
Posted 18 June 2012 - 08:25 PM
Well as long as it works, and it's even better that you have used it for the same thing :(/>/> I also prefer the simplicity of your functions (or at least, they look simpler -_-/>/> )There might be some better/efficient way to do it, but it works. I actually use them for the same thing you need them, I already implemented md5, sha256 and sha224. So bad I didn't find that implementation before doing it myself :)/>/>
Also, I was wondering if you could help me fix the bug in this, it is my slightly edited version of the SHA256 implementation I found, but it is causing the bit API to throw the following error:
bit:40: bad argument: double expected, got nil
If not, would you perhaps consider letting me use your version in WolfOS?Edited on 18 June 2012 - 07:05 PM
Posted 18 June 2012 - 09:20 PM
They work for 32-bit ints, but it can be changed to work with any size.You can use this functions:local function leftRotate(x, n) return bit.bor(bit.blshift(x, n), bit.brshift(x, 32 - n)) end local function rightRotate(x, n) return bit.bor(bit.brshift(x, n), bit.blshift(x, 32 - n)) end
haha right told you my way was inefficient :(/>/>