Posted 17 January 2015 - 02:01 PM
Hey there.
Because it seems like i cant get any existing essentia refill system working, i decided to try to build it.
I'm new to all that lua stuff and i'm still learning so please be kind with me and dont judge me for my chaotic code. (Maybe someone can give me some tipps instead?)
This is what my project currently looks like:
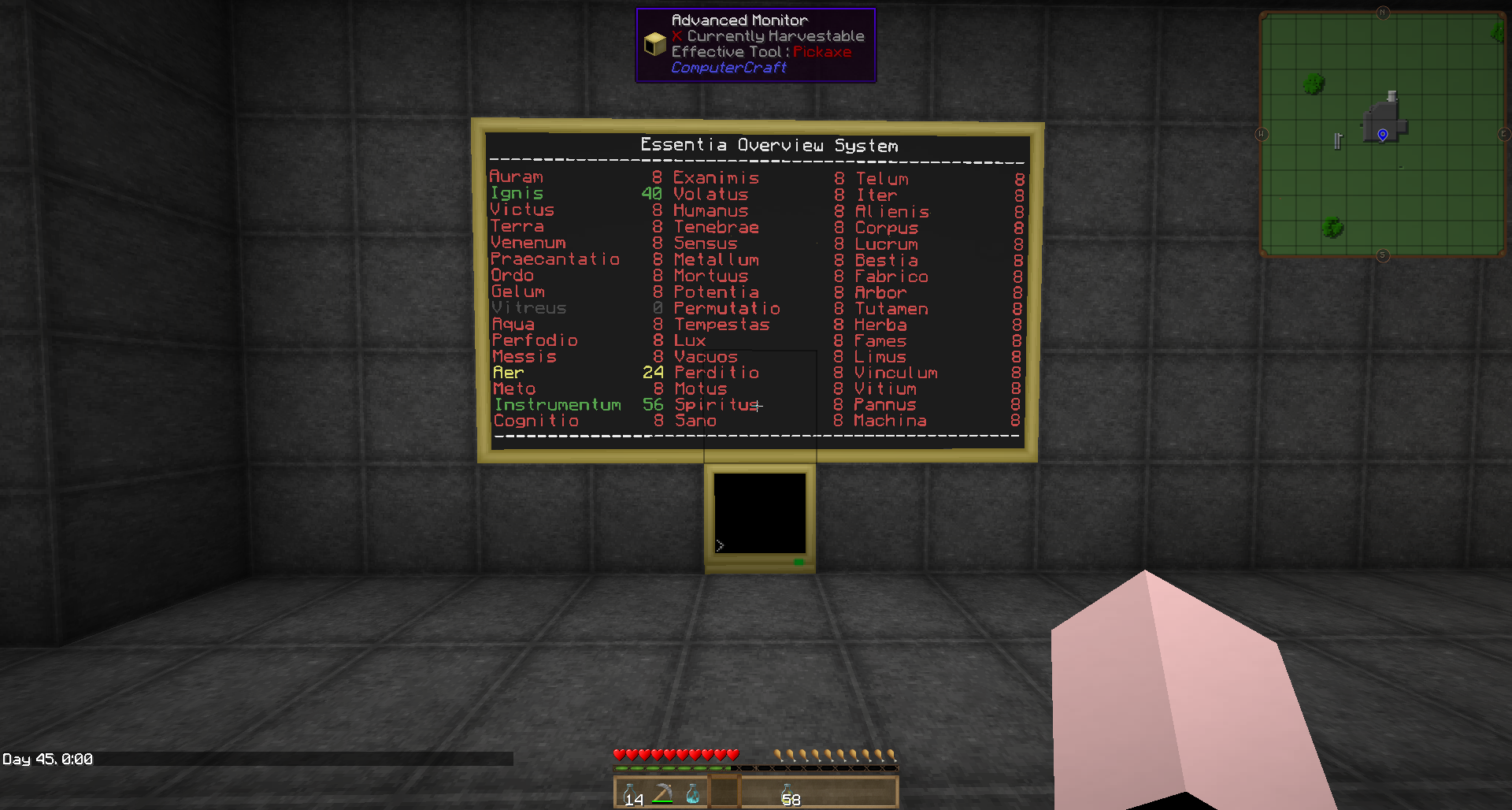
This is my current script:
For now it only reads all the jars and prints the output on the monitor.
Everytime i start the script it sorts all the essentia in a different order.
I think it would be much easier to find the correct essentia when everything would be alphabetically.
Is there any way to sort all the essentia based on my code alphabetically?
If not, what would i have to change to do it?
Sorry for my bad english, i hope its not to hard to understand.
Because it seems like i cant get any existing essentia refill system working, i decided to try to build it.
I'm new to all that lua stuff and i'm still learning so please be kind with me and dont judge me for my chaotic code. (Maybe someone can give me some tipps instead?)
This is what my project currently looks like:
Spoiler
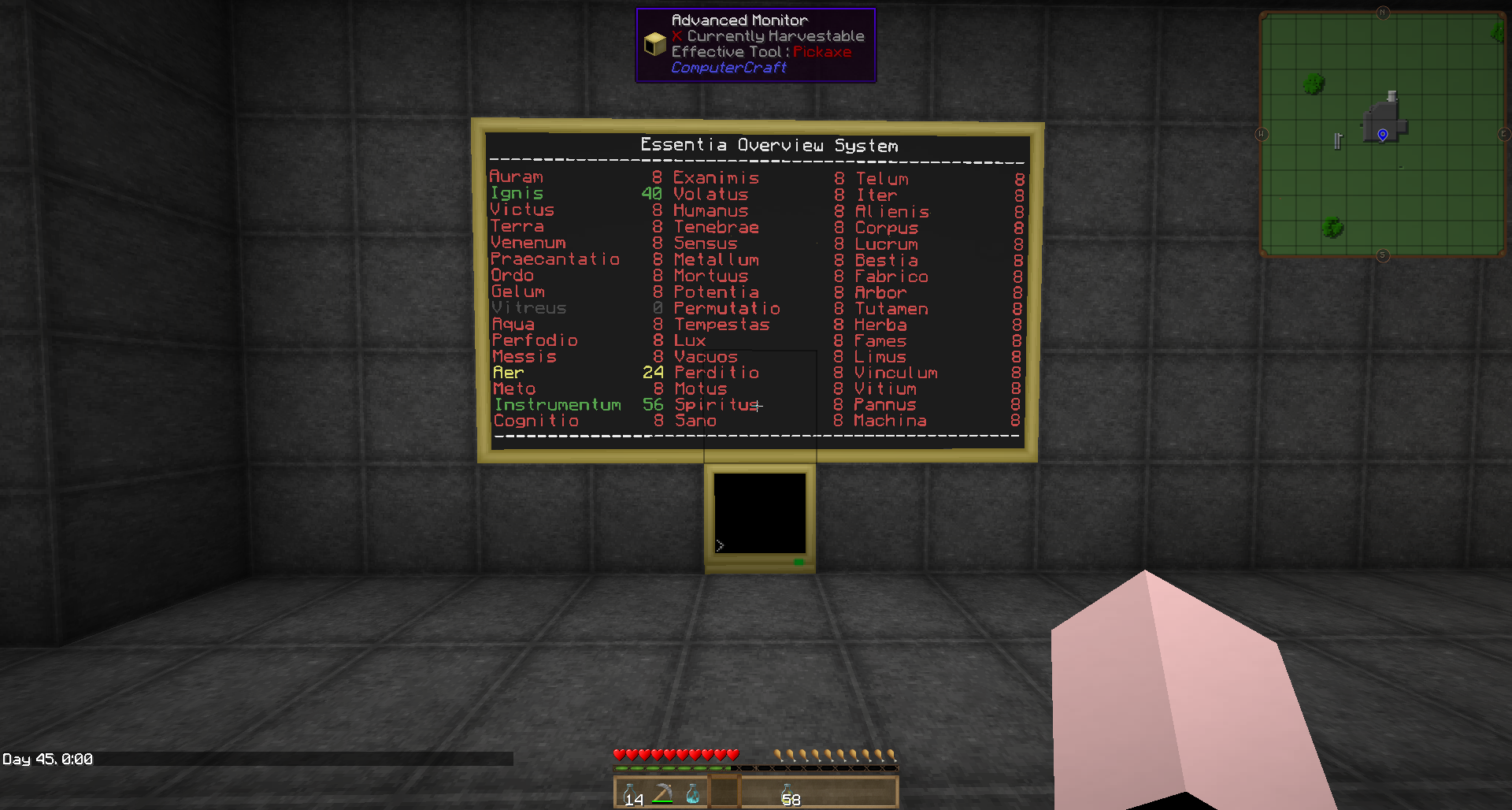
This is my current script:
Spoiler
local essentia = {}
local jars = peripheral.getNames()
local m = peripheral.find("monitor")
function firstToUpper(str)
return (str:gsub("^%l", string.upper))
end
function scanEssentia()
local x = 1
local y = 3
m.clear()
m.setCursorPos(15,1)
m.setTextColor(colors.white)
m.write("Essentia Overview System")
m.setCursorPos(1,2)
m.write("--------------------------------------------------")
m.setCursorPos(1,19)
m.write("--------------------------------------------------")
m.setCursorPos(x,y)
for i,j in ipairs(jars) do
if peripheral.getType(j) == "tt_aspectContainer" then
asp = peripheral.call(j, "getAspects")
if (asp[1] ~= nill) then
for k,v in pairs(asp) do
m.setCursorPos(x,y)
asp = string.lower(asp[1])
countasp = peripheral.call(j, "getAspectCount", asp)
if countasp == 0 then m.setTextColor(colors.gray) end
if countasp > 0 and countasp <= 20 then m.setTextColor(colors.red) end
if countasp < 40 and countasp > 20 then m.setTextColor(colors.yellow) end
if countasp >= 40 then m.setTextColor(colors.green) end
m.write(firstToUpper(asp))
if countasp >= 10 then m.setCursorPos(x+14,y)
else m.setCursorPos(x+15,y) end
m.write(tostring(countasp).." ")
if y < 18 then
y = y+1
else
y = 3
x = x+17
end
end
end
end
end
end
function monitorEssentia()
while true do
scanEssentia()
sleep(5)
end
end
monitorEssentia()
For now it only reads all the jars and prints the output on the monitor.
Everytime i start the script it sorts all the essentia in a different order.
I think it would be much easier to find the correct essentia when everything would be alphabetically.
Is there any way to sort all the essentia based on my code alphabetically?
If not, what would i have to change to do it?
Sorry for my bad english, i hope its not to hard to understand.
Edited on 17 January 2015 - 01:02 PM