Posted 23 January 2015 - 10:23 PM
Cant figure out how to change the color of table text, i have if statements turning on and off lights, i want those to change the value of the relating number so 1 is the number 1=Entrance lights for example. so i want the menu item to be displayed in green or red depending on if its lit up or not. anyone know how to do this? i want the text in the table to change to red og green depending on the if statement.
Here is an example i made just using photoshop of how i want it, either the text to be green and red or a box behinde it. thanks :)/>
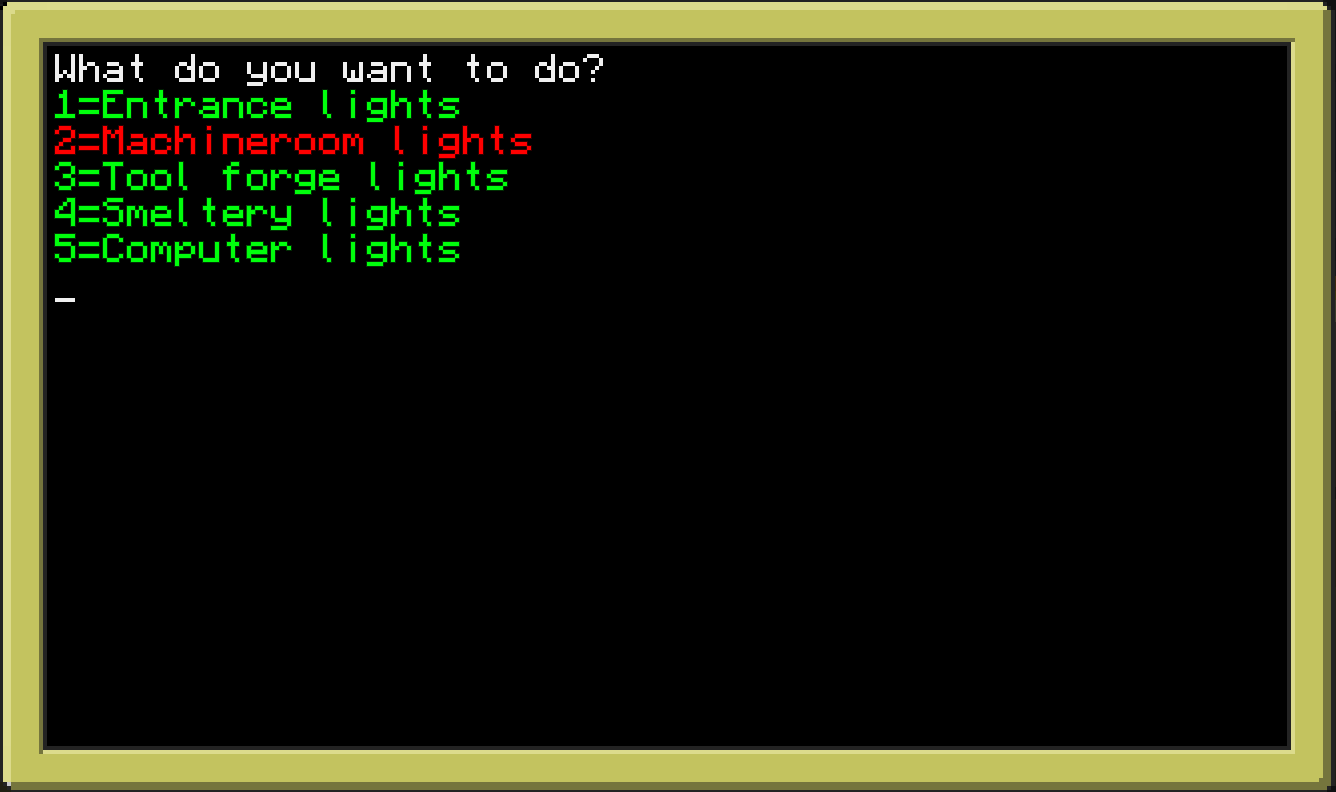
local function addcol(col, side)
rs.setBundledOutput(side, colors.combine(rs.getBundledOutput(side),col))
end
local function remcol(col,side)
rs.setBundledOutput(side, colors.subtract(rs.getBundledOutput(side),col))
end
while true do
term.clear()
term.setCursorPos(1,1)
local menu1 = {}
menu1[1] = "What do you want to do?"
menu1[2] = "1=Entrance lights"
menu1[3] = "2=Machineroom lights"
menu1[4] = "3=Tool forge lights"
menu1[5] = "4=Smeltery lights"
menu1[6] = "5=Computer lights"
print(menu1[1])
print(menu1[2])
print(menu1[3])
print(menu1[4])
print(menu1[5])
print(menu1[6])
input = io.read()
state = rs.getBundledOutput("top")
if input == "1" then
test = colors.test(state, colors.purple)
if not test then
addcol(colors.purple,"top")
else
remcol(colors.purple,"top")
end
else
print("Choose from the menu")
end
if input == "2" then
test = colors.test(state, colors.red)
if not test then
addcol(colors.red,"top")
else
remcol(colors.red,"top")
end
else
print("Choose from the menu")
end
if input == "3" then
test = colors.test(state, colors.green)
if not test then
addcol(colors.green,"top")
else
remcol(colors.green,"top")
end
else
print("Choose from the menu")
end
if input == "4" then
test = colors.test(state, colors.brown)
if not test then
addcol(colors.brown,"top")
else
remcol(colors.brown,"top")
end
else
print("Choose from the menu")
end
if input == "5" then
test = colors.test(state, colors.blue)
if not test then
addcol(colors.blue,"top")
else
remcol(colors.blue,"top")
end
else
print("Choose from the menu")
end
end
Here is an example i made just using photoshop of how i want it, either the text to be green and red or a box behinde it. thanks :)/>
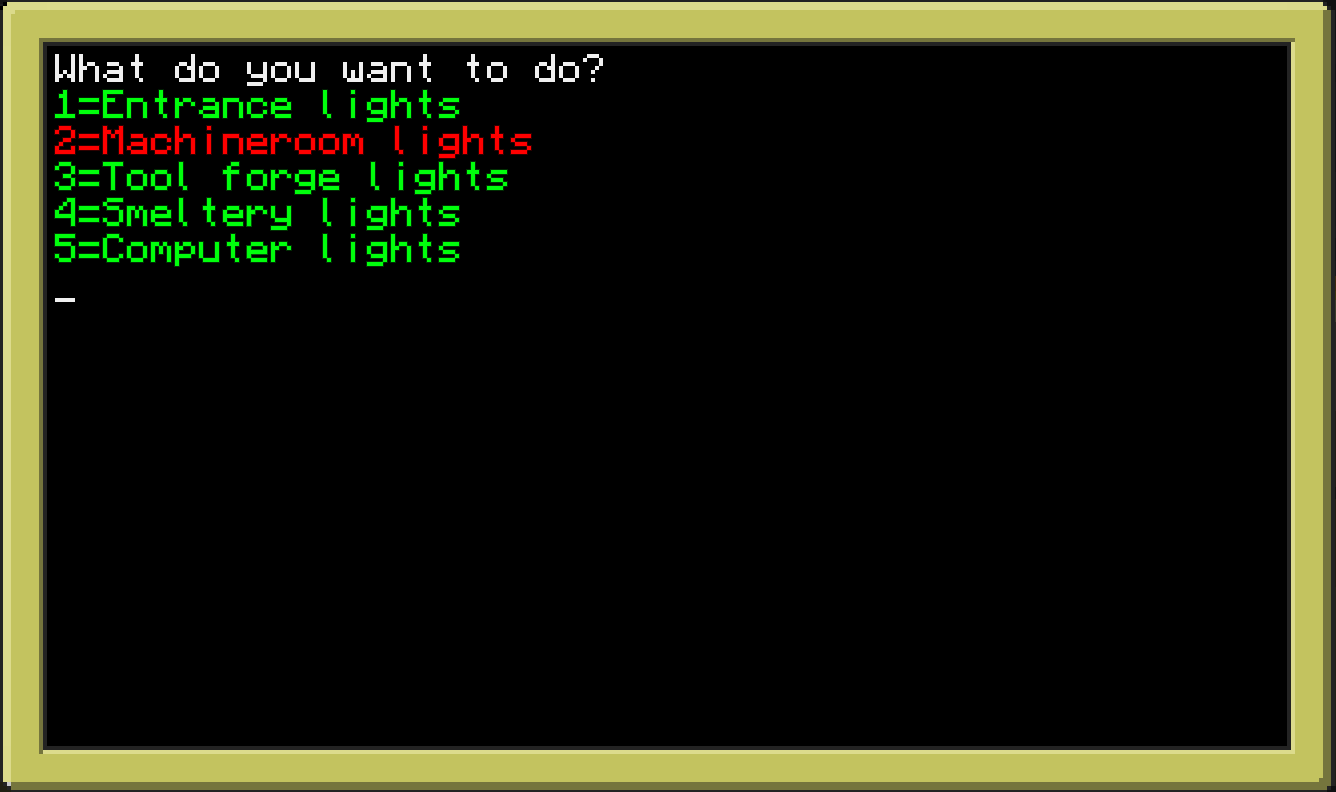