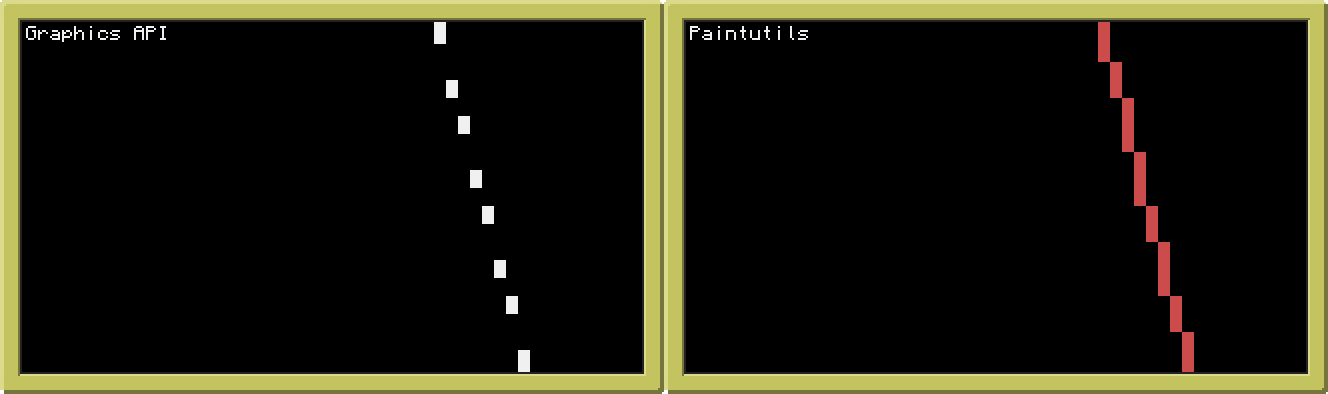
See the difference? I don't really know what's wrong, I've looked into paintutils function but that didn't get me anywhere.
Some help would be much appreciated.
Here's the code for the Graphics API
Graphics API
--[[
[Library] Graphics
@version 1.0, 2015-02-23
@author TheOddByte
--]]
local Graphics = {}
Graphics.setColor = function( col )
assert( type( col ) == "number" or type( col ) == "string", "string/number expected, got " .. type( col ), 2 )
term.setTextColor( type( col ) == "number" and col or colors[col] )
end
Graphics.setBackgroundColor = function( col )
assert( type( col ) == "number" or type( col ) == "string", "string/number expected, got " .. type( col ), 2 )
term.setBackgroundColor( type( col ) == "number" and col or colors[col] )
end
Graphics.writeAt = function( x, y, text )
term.setCursorPos( x, y )
term.write( text )
end
Graphics.line = function( x1, y1, x2, y2, col )
local dx, dy = x2-x1, y2-y1
if col then
Graphics.setBackgroundColor( col )
end
Graphics.writeAt( x1, y1, " " )
if (dx ~= 0) then
local m = dy / dx;
local b = y1 - m*x1;
dx = x2 > x1 and 1 or -1
while x1 ~= x2 do
x1 = x1 + dx
y1 = math.floor( m*x1 + b + 0.5);
Graphics.writeAt( x1, y1, " " )
end
end
end
Graphics.rectangle = function( mode, x, y, width, height, col )
assert( mode == "fill" or mode == "line", "invalid mode", 2 )
Graphics.setBackgroundColor( col )
local line = string.rep( " ", width )
if mode == "fill" then
for i = 1, height do
Graphics.writeAt( x, (y-1)+i, line )
end
else
for i = 1, height do
if i == 1 or i == height then
Graphics.writeAt( x, (y-1)+i, line )
else
Graphics.writeAt( x, (y-1)+i, " " )
Graphics.writeAt( x + width - 1, (y-1)+i, " " )
end
end
end
end
return Graphics
Main code
local Graphics = dofile( "Graphics" )
local line = {
x1 = 1,
y1 = 3,
x2 = 51;
y2 = 19;
}
local mode = "graphics"
while true do
Graphics.setBackgroundColor( "black" )
term.clear()
Graphics.writeAt( 1, 1, mode == "graphics" and "Graphics API" or "Paintutils" )
if mode == "graphics" then
Graphics.line( line.x1, line.y1, line.x2, line.y2, "white" )
else
paintutils.drawLine( line.x1, line.y1, line.x2, line.y2, colors.red )
end
local e = { os.pullEvent() }
if e[1] == "mouse_click" or e[1] == "mouse_drag" then
if e[2] == 1 then
line.x1, line.y1 = e[3], e[4]
elseif e[2] == 2 then
line.x2, line.y2 = e[3], e[4]
end
elseif e[1] == "key" then
if e[2] == 28 then
mode = mode == "graphics" and "paintutils" or "graphics"
end
elseif e[1] == "char" then
if e[2] == "r" then
local w, h = term.getSize()
line.x1, line.y1 = math.random( 1, w ), math.random( 1, h )
line.x2, line.y2 = math.random( 1, w ), math.random( 1, h )
end
end
end