Posted 30 March 2015 - 05:07 PM
Hi all.
Been trying to make this turtle that goes around and around this 3x3x1 spot. You see, in the center, is a "Pure Daisy" from Botania. I want the turtle to identify the "Livingwood" and "Livingstone" generated by the daisy, dig it up, replace it with its minecraft counterpart, and move on. 1 hour of coding later, and all the experience I could call upon from my work as a Garry's Mod developer, I made this piece of crap: http://pastebin.com/7xBHcqKE
The painfully obvious problem: A stack overflow in my "Think" function. My question? How do I make (or hook into) some form of Think function that will run each tick (or at least, loop the function)?
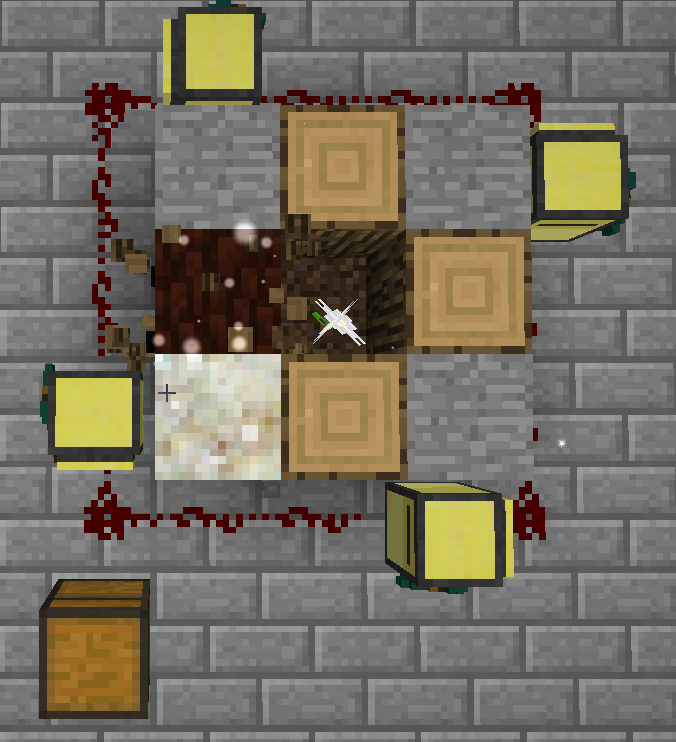
For simplicity's sake, here is a screenshot. The turtle (1 turtle when in operation, the extras are just for effect here) follows this redstone path clockwise, at each step, its supposed to turn right and inspect a block). An eventual desire of mine is to have it dump into that chest every once in a while.
Been trying to make this turtle that goes around and around this 3x3x1 spot. You see, in the center, is a "Pure Daisy" from Botania. I want the turtle to identify the "Livingwood" and "Livingstone" generated by the daisy, dig it up, replace it with its minecraft counterpart, and move on. 1 hour of coding later, and all the experience I could call upon from my work as a Garry's Mod developer, I made this piece of crap: http://pastebin.com/7xBHcqKE
Spoiler
storeSlots = {
livingwood = {1,2,3,4},
livingstone = {5,6,7,8}
}
placeSlots = {
log = {9,10},
stone = {11,12}
}
fuelSlots = {13,14,15,16}
mineThese = {"livingwood","livingstone"}
placeThese = {"log","stone"}
fuelThese = {"minecraft:coal","minecraft:charcoal"}
function refuel()
--Check all slots for fuel.
local startfuel = turtle.getFuelLevel()
if startfuel == "unlimited" or startfuel >= 10 then return true end
local fuelslots = {}
for k,v in pairs(fuelSlots) do --Reserve 13-16 for fuel to minimize calc time.
local itemDetail = turtle.getItemDetail(v)
for i = 1, #fuelThese do
if itemDetail.name == fuelThese[i] then
turtle.refuel(turtle.getItemSpace(v))
break
end
end
if turtle.getFuelLevel() >= 10 then break end
end
if turtle.getFuelLevel() < 10 then refuel() end
end
function checkBlock()
turtle.turnRight() -- Look at the block.
local success,block = turtle.inspect()
if success == true then
for i = 1, #mineThese do
if block.name == "Botania:" .. mineThese[i] then
turtle.dig()
for k,v in pairs( storeSlots[mineThese[i]] ) do
turtle.select(v)
turtle.suck()
end
for k,v in pairs( placeSlots[placeThese[i]] ) do
turtle.select(v)
local placed = nil
if not turtle.getItemSpace(v) == 0 then
placed = turtle.place("")
end
if placed then break end
end
end
end
end
turtle.turnLeft()
turtle.forward()
end
local function Think()
refuel()
checkBlock()
checkBlock()
checkblock()
turtle.turnRight()
Think() -- again please.
end
Think()
Spoiler
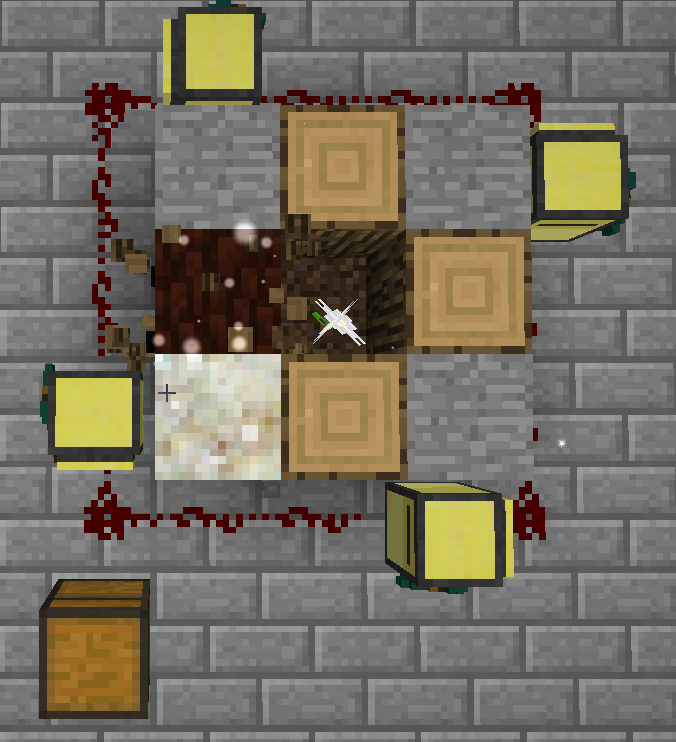
For simplicity's sake, here is a screenshot. The turtle (1 turtle when in operation, the extras are just for effect here) follows this redstone path clockwise, at each step, its supposed to turn right and inspect a block). An eventual desire of mine is to have it dump into that chest every once in a while.