This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
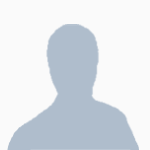
how to print document?
Started by YUGATUG, 05 April 2015 - 02:31 PMPosted 05 April 2015 - 04:31 PM
How do you print the contents of a text file?
Posted 05 April 2015 - 04:34 PM
How do you print the contents of a text file?
local f = fs.open("file", "r")
local contents = f.readAll()
f.close()
print(contents)
I hope it helps.You might want to take a look at the fs API.
Edited on 05 April 2015 - 02:42 PM
Posted 05 April 2015 - 06:56 PM
if you mean the printer block you may be wanting to look at the printer API
Posted 05 April 2015 - 07:19 PM
The edit program gives you the option to print
When editing a file, hit ctrl to open the menu, and one of the options should be print
If you want to make a program that prints a file, you'll need to use the printer API (Link in lupus's post above)
When editing a file, hit ctrl to open the menu, and one of the options should be print
If you want to make a program that prints a file, you'll need to use the printer API (Link in lupus's post above)
Posted 05 April 2015 - 07:44 PM
I meant print as in print(), i am about to test the code of your first post
Edited on 05 April 2015 - 05:44 PM
Posted 05 April 2015 - 07:56 PM
it returns an error at line 8: "attempt to call nil", help?
pastebin.com/jaYn3F0b
pastebin.com/jaYn3F0b
Edited on 05 April 2015 - 06:16 PM
Posted 05 April 2015 - 08:00 PM
The writing to the file is good, reading, not so much.
This should work:
This should work:
--# Write to file
myDocument = {"yolo"}
myFile = fs.open("myFile.txt", "w")
myFile.writeLine(myDocument[1])
myFile.close()
--# Read file
myFile = fs.open("myFile.txt", "r")
contents = myFile.readAll()
myFile.close()
--# Print file
print(contents)
Posted 05 April 2015 - 08:00 PM
You closed the file before reading it.
Posted 05 April 2015 - 08:22 PM
The writing to the file is good, reading, not so much.
This should work:--# Write to file myDocument = {"yolo"} myFile = fs.open("myFile.txt", "w") myFile.writeLine(myDocument[1]) myFile.close() --# Read file myFile = fs.open("myFile.txt", "r") contents = myFile.readAll() myFile.close() --# Print file print(contents)
That is what he has for the read already…
You closed the file before reading it.
No he didn't
Posted 05 April 2015 - 08:26 PM
Did you even look at the pastebin? :P/>The writing to the file is good, reading, not so much.
This should work:--# Write to file myDocument = {"yolo"} myFile = fs.open("myFile.txt", "w") myFile.writeLine(myDocument[1]) myFile.close() --# Read file myFile = fs.open("myFile.txt", "r") contents = myFile.readAll() myFile.close() --# Print file print(contents)
That is what he has for the read already…You closed the file before reading it.
No he didn't
Posted 05 April 2015 - 08:28 PM
That is what he has for the read already…
This was his original:
myFile, myDocument = fs.open("myDocument", "r"), {}--allows document to be written, i don't know what ", {}" does but someone on the forums said to include it
table.insert(myDocument)--not exactly sure what this does
myDocument.close()--done reading
myFile = fs.open("myDocument", "r")
contents = myDocument.readAll()
print(contents)
No, it isn't the same, notice this line (Second to last):
contents = myDocument.readAll() --# myDocument is the table from above, not the file handle
Edited on 05 April 2015 - 06:29 PM
Posted 05 April 2015 - 09:26 PM
Awesome, finally working! I made a thread that went on for two pages and couldn't get it to work, thanks a million man!
Another question: If i read from a document, then write to it before executing any other command, do i have to use fs.close()?
Another question: If i read from a document, then write to it before executing any other command, do i have to use fs.close()?
Posted 05 April 2015 - 09:32 PM
ALWAYS close your files
Posted 05 April 2015 - 10:06 PM
Yes master, please don't hurt me!
jk, thanks for tip :P/>
jk, thanks for tip :P/>
Posted 06 April 2015 - 08:33 PM
Another question: using writeLine(), myDocument gets written to the first line of myDocument.txt, but i want myDocument to be added to myDocument.txt. how would i do this?
Posted 06 April 2015 - 08:38 PM
You might want to look into the append mode for fs.open. This allows for you to add things onto the end of the file instead of the file being erased when it is opened.
temp = fs.open("example","a")
temp.writeLine("hello")
temp.close()
[code]
This would write to the end of the file.
Posted 06 April 2015 - 08:52 PM
you want the file name at the top of the file?
http://computercraft.info/wiki/Fs.getName
http://computercraft.info/wiki/Fs.getName
Posted 06 April 2015 - 09:08 PM
not at all… when i apply this to program, i'm going to have this syntax: "Enderchest add <string>", and i want all strings added using that command to be stored, but using the method i currently am, if i type "Enderchest add yolo" then "Enderchest add gg" yolo will not be stored, but i want it to be.
Posted 06 April 2015 - 09:11 PM
can you post the code?
Posted 06 April 2015 - 09:16 PM
pastebin.com/Hm8YAJtw
Getting an error on line 10, don't know why. help? fixed the error, put 3 dots instead of 2 to combine strings.
Edited on 06 April 2015 - 07:28 PM
Posted 06 April 2015 - 09:20 PM
It appears you want to open the file in append mode, this allows you to add lines into the end of the file instead of erasing the file when writing.
Posted 06 April 2015 - 09:27 PM
the three dots that you are using to concatenate the string should be two
change … to ..
the fixed line is
three dots are used to fetch parameters, two for combining strings
change … to ..
the fixed line is
print(args[2] .. " is already on the list")
three dots are used to fetch parameters, two for combining strings
Edited on 06 April 2015 - 07:29 PM
Posted 07 April 2015 - 12:15 AM
how do i open the file in append mode? do i use an "a" instead of a "w" or a "r"?
Edited on 06 April 2015 - 10:16 PM
Posted 07 April 2015 - 12:46 AM
Yes.how do i open the file in append mode? do i use an "a" instead of a "w" or a "r"?
Posted 07 April 2015 - 07:27 AM
a - append, w - write and r - read.
Posted 08 April 2015 - 12:11 AM
pastebin.com/PACL177r
Tried to make the string store on top of eachother, but the i variable is lost at the end of the program, rendering it useless. Help?
Tried to make the string store on top of eachother, but the i variable is lost at the end of the program, rendering it useless. Help?
Posted 08 April 2015 - 12:29 AM
What, exactly, are you trying to accomplish with that program?
I've commented what it is doing to help you out
I've commented what it is doing to help you out
i = 1 --#set i to 1 immediately, overwriting any previous declaration of i
contents = " " --#make a global variable (FYI this overwrites any previous vars too) (why?)
myFile = " " --# ^
myDocument = " " --# ^^
argstringUse = {" "} --#define a new table, overwriting any previous tables
args = {...} --#globally declare a table of arguments
if args[1] == "add" then --#if they said add then
myFile = fs.open("myFile.txt", "r") --#open your file to a global variable
contents = myFile.readAll() --#read the contents, putting them in another global variable
myFile.close() --#close it
argstringUse[i] = args[2] --#set the first key in your fresh table to the second argument
if contents == argstringUse[1] then --#if the entire previous document is equal to what they entered
print(args[2] .. " is already on the list") --#tell them it's on a list
else
myFile = fs.open("myFile.txt", "w") --#open the document in write mode
myFile.write(args[2]) --#overwrite everything with the second argument
myFile.close() --#close the file
i = i + 1 --#uselessly add 1 to i, since we never loop again
end
end
Posted 08 April 2015 - 01:06 AM
My end goal is to have a program that will have this syntax: Enderchest add <string>/Enderchest search <string>/Enderchest list/Enderchest delete <string> . Enderchest add <string> will add a string to a document of strings (for storage after the program ends). Enderchest search <string> will take <string> and compare it to the strings in the document. If the program finds that <string> and one of the strings in the document match, it will print(<string>.." is in use."). else, it will print(<string>.." is available."). Enderchest list will list out all of the strings in the document (if there are too many to display, it will allow you to hit up and down to go up and down the list). Enderchest delete <string> will delete <string> from the document. if <string> was never in the document, it will print(<string>.." was not on the list."). else, it will print(<string>.."has been deleted.")
right now, i'm coding a prototype, and im trying to make the stage where i make list of strings. to do this, i am using .writeLine(). the problem with .writeLine() is that when it is used like so: 'document.writeLine(array[1])', whatever is stored in the first spot of array will become the first line of document. In effect, that means document.writeLine(array[2]) makes the second line of document whatever is stored in the first spot of array. that means this means that i can't use .writeLine(array[1]) everytime, because then the previous <string>'s get lost.
to counteract that, i tried to count the number of times the user adds strings (hence the i = i + 1), so that the line <string> gets written to doesn't overwrite previous strings. but, once all is said and done, the program ends, and i gets reset to one.
That is currently what i'm trying to solve.
Thanks for commenting my horrendous code by the way! it cleared up the purpose to myself :D/>
right now, i'm coding a prototype, and im trying to make the stage where i make list of strings. to do this, i am using .writeLine(). the problem with .writeLine() is that when it is used like so: 'document.writeLine(array[1])', whatever is stored in the first spot of array will become the first line of document. In effect, that means document.writeLine(array[2]) makes the second line of document whatever is stored in the first spot of array. that means this means that i can't use .writeLine(array[1]) everytime, because then the previous <string>'s get lost.
to counteract that, i tried to count the number of times the user adds strings (hence the i = i + 1), so that the line <string> gets written to doesn't overwrite previous strings. but, once all is said and done, the program ends, and i gets reset to one.
That is currently what i'm trying to solve.
Thanks for commenting my horrendous code by the way! it cleared up the purpose to myself :D/>
Posted 08 April 2015 - 01:14 AM
For what you want to achieve, I'd do something like this:
local contents, tArgs = {}, {...}
if fs.exists( "myFile" ) then
local file = fs.open( "myFile", "r" )
for line in file.readLine do
contents[ line ] = true
end
end
if tArgs[ 1 ] == "add" then
if contents[ tArgs[ 2 ] ] then
print( tArgs[ 2 ] .. " is already on the list" )
else
local file = fs.open( "myFile", "a" )
file.writeLine( tArgs[ 2 ] )
file.close()
end
end
Posted 08 April 2015 - 08:36 PM
Wow dude, thanks for the help!
Do you know how i'd add in the list feature? I don't really understand you're code so i can't edit it…
Do you know how i'd add in the list feature? I don't really understand you're code so i can't edit it…
Edited on 08 April 2015 - 06:37 PM
Posted 08 April 2015 - 10:01 PM
local contents, tArgs = {}, {...} --#contents is a new table, tArgs is the arguments
if fs.exists( "myFile" ) then --#if the file exists
local file = fs.open( "myFile", "r" ) --#open it
for line in file.readLine do --#iterate through the lines of the file
contents[ line ] = true --#say the line was "Hello World", this would make contents[ "Hello World" ] = true
end
end
if tArgs[ 1 ] == "add" then --#if they said add
if contents[ tArgs[ 2 ] ] then --#if the contents table contains the line
print( tArgs[ 2 ] .. " is already on the list" ) --#print it's already on the list
else
local file = fs.open( "myFile", "a" ) --#open in append mode (doesn't overwrite)
file.writeLine( tArgs[ 2 ] ) --#write the new line at the end of the file
file.close() --#close it
end
elseif tArgs[ 1 ] == "list" then
for k, v in pairs( contents ) do --#iterate through the contents
print( k ) --#print the key (note: line 5 adds them as keys, not values)
end
end
Posted 08 April 2015 - 10:14 PM
You are honestly amazing bro, I am speechless. Thank you so much for the help!
So far you've pretty much written my code for me, which I'm not opposed to if you're not, so if you'd make the program for me that'd be AMAZING, but i totally understand if not.
If not, do you know how i'd be able to add the delete feature?
also, problem: if there are too many strings, the program will not display all of the strings. I wanted to have the user be able to use the up and down arrows to scroll up and down the list, then enter or space or some other special key to exit scroll mode, with text at the bottom of the screen constantly displaying "Hit [UP] and [DOWN] to scroll up and down the list, and hit space/enter to exit scroll mode." until the user exits scroll mode. I have no idea how i'd even begin to write that, can you help me out?
So far you've pretty much written my code for me, which I'm not opposed to if you're not, so if you'd make the program for me that'd be AMAZING, but i totally understand if not.
If not, do you know how i'd be able to add the delete feature?
also, problem: if there are too many strings, the program will not display all of the strings. I wanted to have the user be able to use the up and down arrows to scroll up and down the list, then enter or space or some other special key to exit scroll mode, with text at the bottom of the screen constantly displaying "Hit [UP] and [DOWN] to scroll up and down the list, and hit space/enter to exit scroll mode." until the user exits scroll mode. I have no idea how i'd even begin to write that, can you help me out?
Edited on 08 April 2015 - 08:14 PM
Posted 09 April 2015 - 02:27 AM
I'd rather not write programs for you, it's just with this sort of question it's often easier to write & comment than explain how you could do it.
A delete feature is fairly simple, I think you can handle it. First off, open the file in write mode. Next, iterate through the table like I did in list. Compare the key (k) to the second argument (tArgs[2]) using not equals to (~=). If they don't match, use .writeLine to write the line to your file. If they do, you don't have to do anything - it'll just not be written to the file.
The scrolling bit is a lot harder, an easier way of going about it is adding a slight delay after it prints each value (about 0.1 seconds), which should enable the user to read each line while keeping the code fairly simple. Adding a whole scrolling system would almost double the size of the program as it is now.
If you don't want to add a delay, you should look into key events. You'll want a variable to keep track of what you are displaying at any given time, and add/subtract to/from that variable when up/down is pressed. The keys api provides easy access to those values, keys.up is the up arrow, keys.down is the down arrow, etc. Something else you might be interested in is break, which exits a loop. Example:
A delete feature is fairly simple, I think you can handle it. First off, open the file in write mode. Next, iterate through the table like I did in list. Compare the key (k) to the second argument (tArgs[2]) using not equals to (~=). If they don't match, use .writeLine to write the line to your file. If they do, you don't have to do anything - it'll just not be written to the file.
The scrolling bit is a lot harder, an easier way of going about it is adding a slight delay after it prints each value (about 0.1 seconds), which should enable the user to read each line while keeping the code fairly simple. Adding a whole scrolling system would almost double the size of the program as it is now.
If you don't want to add a delay, you should look into key events. You'll want a variable to keep track of what you are displaying at any given time, and add/subtract to/from that variable when up/down is pressed. The keys api provides easy access to those values, keys.up is the up arrow, keys.down is the down arrow, etc. Something else you might be interested in is break, which exits a loop. Example:
local i = 1 --#this gives the same functionality as "for i = 1, 3 do print( i ) end", but shows how break works.
while true do
print( i )
if i == 3 then
break
end
i = i + 1
end
Edited on 09 April 2015 - 12:28 AM
Posted 11 April 2015 - 04:48 PM
You're not understanding what I want to do with the delete feature.
When i type 'Enderchest delete <string>' I want the program to run through the strings in the document to see if <string> matches any of the stored strings. If it does, delete the string in the list, and print(<string> .. "has been deleted.) . else, print(<string> .. "was never on the list.")
When i type 'Enderchest delete <string>' I want the program to run through the strings in the document to see if <string> matches any of the stored strings. If it does, delete the string in the list, and print(<string> .. "has been deleted.) . else, print(<string> .. "was never on the list.")
Posted 11 April 2015 - 04:53 PM
What I said will do that.
elseif tArgs[ 1 ] == "delete" then
if contents[ tArgs[ 2 ] ] then --#if it is there
local file = fs.open( "myFile", "w" ) --#open the file
for k, v in pairs( contents ) do --#iterate
if tArgs[ 2 ] ~= k then --#if it's not the line to delete
file.writeLine( k ) --#write it down
end
end
else
print( tArgs[ 2 ] .. " was never on the list." )
end
end
Posted 11 April 2015 - 04:55 PM
also, problem: if there are too many strings, the program will not display all of the strings. I wanted to have the user be able to use the up and down arrows to scroll up and down the list, then enter or space or some other special key to exit scroll mode, with text at the bottom of the screen constantly displaying "Hit [UP] and [DOWN] to scroll up and down the list, and hit space/enter to exit scroll mode." until the user exits scroll mode. I have no idea how i'd even begin to write that, can you help me out?
My getMethods program is designed to show you the functions a peripheral has, but behind the scenes, it really is just displaying a table that spans several pages. The table is created with 'peripheral.getMethods'
If you want, you can try to modify it to work with your program, it shouldn't be too hard
Posted 11 April 2015 - 05:42 PM
Or just textutils.pagedTabulate(peripheral.getMethods("side")).
Posted 11 April 2015 - 06:18 PM
Or just textutils.pagedTabulate(peripheral.getMethods("side")).
True, that world work, mine lets you use the arrow keys to navigate back and forth
Posted 12 April 2015 - 05:35 PM
line 5 and 6:What I said will do that.elseif tArgs[ 1 ] == "delete" then if contents[ tArgs[ 2 ] ] then --#if it is there local file = fs.open( "myFile", "w" ) --#open the file for k, v in pairs( contents ) do --#iterate if tArgs[ 2 ] ~= k then --#if it's not the line to delete file.writeLine( k ) --#write it down end end else print( tArgs[ 2 ] .. " was never on the list." ) end end
–#if it's not the line to delete
–#write it down
if i understand you correctly, that's saying that if <string> does not match k, then the program will write in k (k is the current string the program is comparing against, right?). if it does, the program will print(<string> .. "was never on the list.") , which means that the <string> in the document will never be deleted. Am i understanding your code correctly?
Posted 12 April 2015 - 06:02 PM
if i understand you correctly, that's saying that if <string> does not match k, then the program will write in k (k is the current string the program is comparing against, right?). if it does, the program will print(<string> .. "was never on the list.") , which means that the <string> in the document will never be deleted. Am i understanding your code correctly?
Actually, the idea is that it will open the file that stores them, and rewrite every line, EXCEPT the one you specify to delete.
Remember that when opening files in "w" mode, it deletes the content of the file before you write anything
Posted 13 April 2015 - 01:21 AM
The
is part of this if
Notice the indentation, it makes code easier to read.
else
is part of this if
if contents[ tArgs[ 2 ] ] then --#if it is there
Notice the indentation, it makes code easier to read.
Posted 14 April 2015 - 10:54 PM
if i understand you correctly, that's saying that if <string> does not match k, then the program will write in k (k is the current string the program is comparing against, right?). if it does, the program will print(<string> .. "was never on the list.") , which means that the <string> in the document will never be deleted. Am i understanding your code correctly?
Actually, the idea is that it will open the file that stores them, and rewrite every line, EXCEPT the one you specify to delete.
Remember that when opening files in "w" mode, it deletes the content of the file before you write anything
oh, i didn't know opening a file in write mode deletes the contents of the file, that clears thing up. Thanks!
Posted 14 April 2015 - 11:17 PM
Here's the deal: I'm getting bored of this project. I have the code for a 15 dollar itunes gift card. First person to make the program (with the added scrolling feature *it must work on both pocket computers and regular ones*) as i describe gets the code. (make sure to pm your program to me, i will pm the code back.)
What's that you say? You don't believe i have the gift card? http://imgur.com/Pn4JDL5,RjpWM4C#0 and http://imgur.com/Pn4JDL5,RjpWM4C#1 . the green is to prevent you from seeing the information to cash it in without me wanting you to.
Huh? You think i've already used up the balance of the card?
Well, you might be right. I haven't, but i can't prove to you i haven't.
What? You think i won't give you the code?
You might be right again. I can't sign an internet contract because those don't exist, but i will give you the code.
Your move, internet.
What's that you say? You don't believe i have the gift card? http://imgur.com/Pn4JDL5,RjpWM4C#0 and http://imgur.com/Pn4JDL5,RjpWM4C#1 . the green is to prevent you from seeing the information to cash it in without me wanting you to.
Huh? You think i've already used up the balance of the card?
Well, you might be right. I haven't, but i can't prove to you i haven't.
What? You think i won't give you the code?
You might be right again. I can't sign an internet contract because those don't exist, but i will give you the code.
Your move, internet.
Edited on 14 April 2015 - 09:18 PM
Posted 15 April 2015 - 12:46 AM
make the program as i describe
I don't think this thread actually has a full description of the program. Maybe you should lay out how it should work in one post
You can also try posting in the General section so that more people see it, although I'm not sure if the Admins will let you do the gift card thing
Posted 15 April 2015 - 12:52 AM
We'd generally prefer that those sorts of transactions not be conducted via the forums, but we obviously can't stop people from sending whatever they want in PMs.
Posted 15 April 2015 - 01:29 AM
make the program as i describe
I don't think this thread actually has a full description of the program. Maybe you should lay out how it should work in one post
You can also try posting in the General section so that more people see it, although I'm not sure if the Admins will let you do the gift card thing
oh, i guess you're right, i thought i had typed it out already.