This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
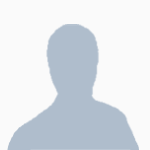
looking into using parallel
Started by kain184, 25 April 2015 - 02:01 AMPosted 25 April 2015 - 04:01 AM
hello all. i stumbled across the parallel api the other day and i just have a few questions for anyone who knows. first if you use a sleep or anything in your command does it exit automaticly or will it keep running in a true loop . and second does all of the program share data like tables and ect
Posted 25 April 2015 - 04:35 AM
If you call sleep() from within one of the functions you pass to the parallel API, then when sleep() calls os.pullEvent() (and therefore, in turn, coroutine.yield()), the API will take that opportunity to see whether the other function(s) is/are ready to run some code. When it later resumes the first function, that'll continue from where it left off. If a function finishes, it is not restarted for you.
In terms of scope, it's the same as if you weren't using the parallel API. The functions will "see" your other variables in exactly the same way as they normally would.
In terms of scope, it's the same as if you weren't using the parallel API. The functions will "see" your other variables in exactly the same way as they normally would.
Posted 25 April 2015 - 05:33 AM
say i have two functions
value=true
function go()
while value do
stuff
value=not value
end
end
function stop()
while not value do
sleep()
end
end
function other()
stuff
end
parallel(go,other)
when it hits that sleep in stop it will kill go and move to other but will not move back to go automatically
Posted 25 April 2015 - 06:57 AM
- Snip -
I don't really see a situation where this would be used, but lets discuss it anyway
This loop will only run once:
value = true
function go()
while value do
--# Do stuff
value = not value --# Stops because of this, obviously
end
end
This function, in your code, will never run - It isn't ever called
function stop()
while not value do
sleep() --# Sleeps for a very short amount of time
end
end
This function would be run after the 'Stuff' in 'go()' finished
function other()
--# More stuff
end
Of course, this is all theoretical, since this call would error (Attempt to call table):
parallel(go,other)
Here is the correct usage:
parallel.waitForAll(go,other)
--# or
parallel.waitForAny(go,other)
waitForAll runs the functions until they are all finishedwaitForAny runs the functions until ONE finishes
('Any' wouldn't be the one for this situation, it wouldn't run 'other', since 'go()' stops without calling 'os.pullEvent')
Remember, Lua doesn't support multiple functions running at once - the parallel API just makes it seem that way
When the first function you give it calls 'os.pullEvent()' in some form, the parallel API goes to the next function and lets it run until THAT function calls 'os.pullEvent()'
Hopefully this was at least a bit helpful ;)/>
Edited on 25 April 2015 - 04:57 AM
Posted 25 April 2015 - 12:26 PM
If you want to know how to use the coroutine API, I would recommend reading the source of the parallel API, as that uses the coroutine API.
However, you do not need to know how to use the coroutine API unless you need to filter the events being passed to the functions.
Edited on 25 April 2015 - 10:26 AM
Posted 25 April 2015 - 12:55 PM
The first thing to know about co-routines is that you probably don't need to be using them. If you want to, then fine; but if you want to step outside of having the parallel API handle them for you (said API basically offers "baby's first co-routine manager" on a platter), then I suggest gaining a strong grounding in tables and functions before you begin.
That is to say, every time you read a co-routine article that doesn't make sense to you, go back and read another tutorial about pointers or somesuch. Figure out the key words or phrases you're hazy on and go find whole documents explaining them. You won't understand the high-level stuff until you understand the low-level stuff, and there's a LOT of low-level concepts to absorb before co-routines will fully make sense to you.
If you simply have a certain goal in mind and don't much care about how you get there, then perhaps if you explain what that goal is someone might offer simpler solution.
Just to elaborate on this; ComputerCraft's specific implementation revolves around actively executing one co-routine at a time. You can have multiple "alive" co-routines, and you can pause them and resume them from where they left off, but while one's actually doing something none of the others are.
This rule applies world-wide - every computer / turtle has its own co-routine, and while one's doing something none of the others are. If one computer starts executing code that doesn't yield very often, all of the others will slow down as a result of that.
That is to say, every time you read a co-routine article that doesn't make sense to you, go back and read another tutorial about pointers or somesuch. Figure out the key words or phrases you're hazy on and go find whole documents explaining them. You won't understand the high-level stuff until you understand the low-level stuff, and there's a LOT of low-level concepts to absorb before co-routines will fully make sense to you.
If you simply have a certain goal in mind and don't much care about how you get there, then perhaps if you explain what that goal is someone might offer simpler solution.
Remember, Lua doesn't support multiple functions running at once - the parallel API just makes it seem that way
Just to elaborate on this; ComputerCraft's specific implementation revolves around actively executing one co-routine at a time. You can have multiple "alive" co-routines, and you can pause them and resume them from where they left off, but while one's actually doing something none of the others are.
This rule applies world-wide - every computer / turtle has its own co-routine, and while one's doing something none of the others are. If one computer starts executing code that doesn't yield very often, all of the others will slow down as a result of that.
Posted 25 April 2015 - 04:28 PM
i was generalizing the functions there i know that it was not the full name of the parallel functions the reason i am asking is im trying to make a game program that right now will have to be managed by three computers i am trying to see if it would be more efficient to use parrelel or three networked by rednet.
but for the basic question i was asking with my code there is if the first exits it does not return to the first and they are not running at the exact same time. is that correct.
but for the basic question i was asking with my code there is if the first exits it does not return to the first and they are not running at the exact same time. is that correct.
Posted 25 April 2015 - 05:37 PM
It's definitely more efficient if you use a single computer.i was generalizing the functions there i know that it was not the full name of the parallel functions the reason i am asking is im trying to make a game program that right now will have to be managed by three computers i am trying to see if it would be more efficient to use parrelel or three networked by rednet.
but for the basic question i was asking with my code there is if the first exits it does not return to the first and they are not running at the exact same time. is that correct.
If I understand you right, you're asking how coroutines work with parallel…
They are not running at the same time, ever. However, each time you do something that yields (os.pullEvent, sleep, turtle.forward, etc.) it switches to the next function you gave it until that one yields, and so on. If a function returns (or finishes), it'll be removed from the list of 'running' functions. Then, depending on how many are left and which function from parallel you were using, it may continue to resume the remaining functions or cancel them entirely and return.
Posted 25 April 2015 - 05:58 PM
i will have to look into this more and ask some more questions i may have to stick with the three computers as i cant see how i can resume a function from where it yeilded unless i write a ton of logic to do it and right now im trying to have a simple program handle a complex task and it is working so long as i have it seperated the problem i am running into is data sharing and thats what im trying to overcome.
Posted 25 April 2015 - 06:32 PM
We can be a lot more helpful if you post your current code and provide a complete explanation of what you're trying to do. You don't need three computers to accomplish it, most likely.