This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
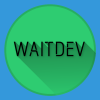
converting keycodes
Started by Waitdev_, 18 May 2015 - 09:48 AMPosted 18 May 2015 - 11:48 AM
i'm making a program, and i need it to get a user input. i don't want it to be io.read(), since that makes a new line and i need it to be only 1 letter. if i could get it to use os.pullEvent("key") and convert that to a number/letter, that would make things a lot easier. by the way, i can't do os.pullEvent("char") since i need it to do all characters.
Posted 18 May 2015 - 12:08 PM
There's no reliable solution to using "key" event to get the pressed letter, number or other character. You'll have to use the "char" event. But don't worry, to listen for more than one event at a time just don't pass an event filter. A common event-catching structure in CC is this (an event loop):
while true do
local e = { os.pullEvent() } --# catch any event and put all it's parameters into a table
if e[1] == "key" then
if e[2] == keys.enter then
elseif ...
elseif e[1] == "char" then
local pressedChar = e[2]
...
elseif ...
end
Posted 18 May 2015 - 12:30 PM
its not really detecting the key, its mainly just getting the key you press then printing that key. sorry i didn't really make it too clear :/There's no reliable solution to using "key" event to get the pressed letter, number or other character. You'll have to use the "char" event. But don't worry, to listen for more than one event at a time just don't pass an event filter. A common event-catching structure in CC is this (an event loop):while true do local e = { os.pullEvent() } --# catch any event and put all it's parameters into a table if e[1] == "key" then if e[2] == keys.enter then elseif ... elseif e[1] == "char" then local pressedChar = e[2] ... elseif ... end
Posted 18 May 2015 - 01:40 PM
There's no reliable solution to using "key" event to get the pressed letter, number or other character. You'll have to use the "char" event. But don't worry, to listen for more than one event at a time just don't pass an event filter. A common event-catching structure in CC is this (an event loop):while true do local e = { os.pullEvent() } --# catch any event and put all it's parameters into a table if e[1] == "key" then if e[2] == keys.enter then elseif ... elseif e[1] == "char" then local pressedChar = e[2] ... elseif ... end
yes there is? the keys api? keys.getName(number code)
http://computercraft.info/wiki/Keys_%28API%29
It is useful if you wish to convert it from key to character.
Posted 18 May 2015 - 01:51 PM
What if caps-lock or shift key is on? How do you know if the character should be uppercase or lowercase. Also, the keys table doesn't really have the printable characters, it's their names actually. For example there's 'numPad1' and 'one' instead of the printable character '1'.
To OP: so you don't need a printable representation of the key but rather it's name, like instead of ',' you'd get 'comma'? Then you can use the keys table. If not then please elaborate more on what you are trying to achieve so we could help as best as we can.
To OP: so you don't need a printable representation of the key but rather it's name, like instead of ',' you'd get 'comma'? Then you can use the keys table. If not then please elaborate more on what you are trying to achieve so we could help as best as we can.
Posted 19 May 2015 - 12:06 AM
i think i'm gonna have to go with converting them all in an array -_-/>
Posted 19 May 2015 - 12:48 AM
while true do local e = { os.pullEvent() } --# catch any event and put all it's parameters into a table if e[1] == "key" then if e[2] == keys.enter then elseif ... elseif e[1] == "char" then local pressedChar = e[2] ... elseif ... end
Why would this not work?
Posted 19 May 2015 - 04:30 AM
i can't do os.pullEvent("char") since i need it to do all characters.
os.pullEvent("char") does return any pressed character.
Let's assume you mean "all keys". Given that pressing a character key generates a key event followed by a char event, pressing a non-character key only generates a key event, and that you only want to get the first button pressed, I'd do something like this:
local myEvent = {os.pullEvent("key")}
os.queueEvent("dummyEvent")
local myEvent2 = {os.pullEvent()}
if myEvent2[1] == "char" then
print("Pressed character \""..myEvent2[2].."\".")
else
print("Pressed key \""..keys.getName(myEvent[2]).."\".")
end