function checkMail()
local option = nil
local file = nil
local temp = nil
local emailName = ""
local startIndex = nil
local endIndex = nil
local filepath = "Emails/"
if fs.exists("Emails") == false then
fs.makeDir("Emails")
end
rednet.broadcast("readmail")
a, messagep = rednet.receive(1)
if messagep ~= nil then
startIndex = messagep:find("Subject: ") + 9
endIndex = messagep:find("Message:") - 1
emailName = string.sub(messagep,startIndex,endIndex)
print(emailName)
filepath = filepath..emailName
print(filepath)
sleep(1)
file = fs.open(filepath, "w") -- offending code
-- I have already checked that the filepath is valid using print()
-- above, so I know that is not the issue. It just seems that the
-- method is not working...
if file then
print("test")
sleep(1)
file.write(messagep)
file.close()
end
end
if fs.isDir("Emails") then
emails = fs.list("Emails")
emails[#emails + 1] = "Exit"
end
option = CUI(emails)
if option ~= #emails then
file = fs.open("Emails/" ..emails[option], "r") -- Strangely enough, it works down here.
temp = file.readAll()
file:close()
print(temp.. "\n")
write("Press Enter to Continue...")
read()
os.run({}, "emailClient")
else
emailMenu()
end
end
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
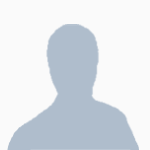
[Lua][Solved] fs.open() issue
Started by TechnoColt, 30 June 2012 - 02:41 AMPosted 30 June 2012 - 04:41 AM
Don't worry, I've read the wiki, and I actually know how to do file I/O, so there won't be any two-second searches that I could have done myself. My problem is that in the included code snippet, fs.open() simply is not working. I can't see anything wrong with the code, and it doesn't throw any sort of error, it just doesn't work. If anyone can spot the issue, I would be very grateful. I've been searching for it for a few days to no avail.
Posted 30 June 2012 - 04:57 AM
What happens? is file==nil?
Posted 30 June 2012 - 05:01 AM
I concatenated the file name onto filepath. Is there something else I need to do get it to recognize it as a file?
EDIT: I guess file is nil, although it still didn't thrown an error before I added the line "if file then". The lack of error message is what's really throwing me off. I'm not sure why fs.open() would be failing to create a file. /:
EDIT: I guess file is nil, although it still didn't thrown an error before I added the line "if file then". The lack of error message is what's really throwing me off. I'm not sure why fs.open() would be failing to create a file. /:
Posted 30 June 2012 - 05:17 AM
Try:
P.S: The same thing has happened to me before, if this doesn't work then): (oddly enough this worked for me) try reinstalling CC
if fs.isDir(filepath) then
print("Can't open "..filepath..", file is a directory!")
else
file = fs.open(filepath, "w")
if file then
print("test")
sleep(1)
file.write(messagep)
file.close()
end
end
And if it says it's a directory, well, then it's a directory and it shouldn't be.P.S: The same thing has happened to me before, if this doesn't work then): (oddly enough this worked for me) try reinstalling CC
Posted 30 June 2012 - 05:20 AM
Try:And if it says it's a directory, well, then it's a directory and it shouldn't be.if not fs.isDir(filepath) then print("Can't open "..filepath..", file is a directory!") else file = fs.open(filepath, "w") if file then print("test") sleep(1) file.write(messagep) file.close() end end
P.S: The same thing has happened to me before, if this doesn't work then): (oddly enough this worked for me) try reinstalling CC
Should that have a not? The way I'm reading it is "if fs.isDir(filepath) == false then". In any case, it doesn't work with or without the not.
Posted 30 June 2012 - 07:28 AM
i think it should be:
if fs.isDir(filepath) then
not
if not fs.isDir(filepath)
:P/>/>Posted 01 July 2012 - 02:51 AM
As I said previously, it doesn't really matter if that should have a "not" or not. I tried it both ways and it does not work. I'm really confused as to why open() would be broken like this…
Posted 01 July 2012 - 02:58 AM
Does the file exist? Cause it could be that you opened and never closed it (if there was an error or something), so now it can't be open.
Posted 01 July 2012 - 04:24 AM
The file does not exist yet. The offending line is supposed to create the file, but it just doesn't. No error is given. It just does nothing.
Posted 01 July 2012 - 11:33 AM
I really hope your username isn't indicative as to what is happening right now. Try cutting down the code to its simplest form, like so.
If that code works, then your system is fine. If not, then reinstall ComputerCraft as something is obviously wrong.
file = fs.open("Emails/test")
file:close()
If that code works, then your system is fine. If not, then reinstall ComputerCraft as something is obviously wrong.
Posted 01 July 2012 - 10:23 PM
No, no. I'm not trolling, haha. I'll try that and let you know if it works.
EDIT: Nope, that didn't work. Guess I'm deleting, doing a clean run, and re-installing.
EDIT2: Clean install didn't work. I probably should have mentioned this earlier, but I tested it on a server and it failed there as well.
Here is the full source code of the Email Client I'm working on. Let me know if you see anything that could be causing this issue:
EDIT: Nope, that didn't work. Guess I'm deleting, doing a clean run, and re-installing.
EDIT2: Clean install didn't work. I probably should have mentioned this earlier, but I tested it on a server and it failed there as well.
Here is the full source code of the Email Client I'm working on. Let me know if you see anything that could be causing this issue:
-- This is my ComputerCraft eMail client code.
local args = {...}
local menu1 = {"Check Email","Send Email","Delete Email","Exit"}
local emails = nil
function CUI(m)
n=1
l=#m
while true do
term.clear()
term.setCursorPos(1,2)
for i=1, l, 1 do
if i==n then print(i, " ["..m[i].."]") else print(i, " ", m[i]) end
end
print("Select a number[arrow up/arrow down]")
a, b= os.pullEventRaw()
if a == "key" then
if b==200 and n>1 then
n=n-1
end
if b==208 and n<l then
n=n+1
end
if b==28 then
break
end
end
end
term.clear() term.setCursorPos(1,1)
return n
end
function emailMenu()
local option = nil
option = CUI(menu1)
if option == 1 then
checkMail()
end
if option == 2 then
sendMail()
end
if option == 4 then
os.run({},"lemonOS", true)
os.exit()
end
end
function checkMail()
local option = nil
local file = nil
local temp = nil
local emailName = ""
local startIndex = nil
local endIndex = nil
local filepath = "Emails/"
if fs.exists("Emails") == false then
fs.makeDir("Emails")
end
rednet.broadcast("readmail")
a, messagep = rednet.receive(1)
if messagep ~= nil then
startIndex = messagep:find("Subject: ") + 9
endIndex = messagep:find("Message:") - 1
emailName = string.sub(messagep,startIndex,endIndex)
print(emailName)
filepath = filepath..emailName
print(filepath)
sleep(1)
if not fs.isDir(filepath) then
print("Can't open "..filepath..", file is a directory!")
else
file = fs.open(filepath, "w")
if file then
print("test")
sleep(1)
file.write(messagep)
file.close()
end
end
end
if fs.isDir("Emails") then
emails = fs.list("Emails")
emails[#emails + 1] = "Exit"
end
option = CUI(emails)
if option ~= #emails then
file = fs.open("Emails/" ..emails[option], "r")
temp = file.readAll()
file:close()
print(temp.. "n")
write("Press Enter to Continue...")
read()
os.run({}, "emailClient")
else
emailMenu()
end
end
function sendMail()
write("Do you want to send mail? (Y/N) ")
input = read()
input = string.upper(input)
if input == "Y" then
rednet.broadcast("sendmail")
write("To: (in ID) ")
input = read()
rednet.broadcast(input)
write("Subject: ")
input = read()
rednet.broadcast(input)
print("Message body:")
input = read()
rednet.broadcast(input)
end
emailMenu()
end
emailMenu()
Posted 01 July 2012 - 10:59 PM
Try changing this line:
to:
and checking the output.
filepath = filepath..emailName
to:
filepath = fs.combine(filepath, emailName)
and checking the output.
Posted 01 July 2012 - 11:00 PM
Alright, I'll try that. Out of curiosity, why is that better than simple concatenation? The print statement works just fine, so it's clearly a valid string.
EDIT: Output's fine, just as it was with normal concatenation. The fs.open() function is still not working, unfortunately.
EDIT: Output's fine, just as it was with normal concatenation. The fs.open() function is still not working, unfortunately.
Posted 01 July 2012 - 11:00 PM
Well, the not shouldn't be in this line:
if not fs.isDir(filepath) then
since you want to check if it is a directory. Other than that, I see no error.Posted 01 July 2012 - 11:08 PM
Well, the not shouldn't be in this line:since you want to check if it is a directory. Other than that, I see no error.if not fs.isDir(filepath) then
Ah, didn't catch that. That wasn't my code anyway. Thesbros suggested it. As such, it can't be causing the issue.
Posted 01 July 2012 - 11:10 PM
Check if "Emails" is a directory, cause if it isn't that's the problem.
Posted 01 July 2012 - 11:15 PM
It's definitely a directory. It was created by the checkMail() function.
Nevertheless, I'll add an if then statement to check whether "Emails" is a dir.
EDIT: The fs.isDir() function returned true. Any other suggestions?
if fs.exists("Emails") == false then
fs.makeDir("Emails")
end
Nevertheless, I'll add an if then statement to check whether "Emails" is a dir.
EDIT: The fs.isDir() function returned true. Any other suggestions?
Posted 01 July 2012 - 11:43 PM
Ok, create a new program, containing this:
local file = fs.open("test123", "w")
if file then
file.close()
print("The file was created.")
fs.delete("test123")
else
print("Error creating file.")
end
and then run it.Posted 02 July 2012 - 12:48 AM
That code worked. What should I do now?
Posted 02 July 2012 - 01:16 AM
In ther terminal, type:
> cd /Emails
> ls
And post what you see.
> cd /Emails
> ls
And post what you see.
Posted 02 July 2012 - 01:25 AM
Oops, my bad, it shouldn't have a not. XDWell, the not shouldn't be in this line:since you want to check if it is a directory. Other than that, I see no error.if not fs.isDir(filepath) then
Ah, didn't catch that. That wasn't my code anyway. Thesbros suggested it. As such, it can't be causing the issue.
Posted 02 July 2012 - 02:32 AM
In ther terminal, type:
> cd /Emails
> ls
And post what you see.
The directory is empty.
Posted 02 July 2012 - 02:39 AM
Ok, there's no errors, it has to work. I don't see anything else, sorry.
Posted 02 July 2012 - 05:44 AM
This is why I'm so flabbergasted. There's no reason *why* it shouldn't work, it just doesn't. :P/>/>
Posted 02 July 2012 - 06:55 AM
I have solved my error. When creating the file name, I had a leftover endline character, so the file could not open properly. Thank you so much to everyone who assisted me.
Posted 02 July 2012 - 04:24 PM
Good to know you solved it.
So many troubles for a single character :P/>/>
So many troubles for a single character :P/>/>