Posted 17 July 2015 - 01:05 PM
I am trying to make a turtle go forward and make a bridge, when it gets to the other side it comes back making the 2nd side of the bridge so it is a 2*2 bridge. Unfortunetly this is not working, I have tried debuging this and solved quite a few issues but I cant seem to find the current issue.
Here is an gif of what it is doing. I have realised it reacts to the stone even though I have tried telling it to ignore the stone.
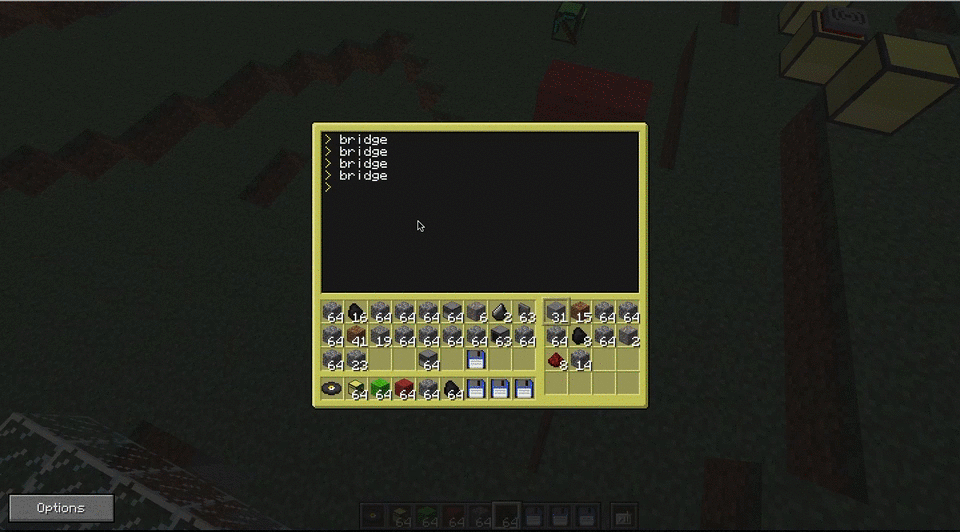
if turtle.detect() ~= "minecraft:air" then
turtle.dig()
turtle.forward()
turtle.placeDown()
else
turtle.forward()
turtle.placeDown()
end
X1 = false
while true do
if turtle.detectDown() ~= "minecraft:air" and turtle.detectDown() ~= "minecraft:stone" and X1 == false then
turtle.turnRight()
if turtle.detect() ~= "minecraft:air" then
turtle.dig()
turtle.forward()
else
turtle.forward()
end
if turtle.detect() ~= "minecraft:air" then
turtle.dig()
turtle.forward()
turtle.placeDown()
else
turtle.forward()
turtle.placeDown()
end
turtle.turnRight()
X1 = true
end
if turtle.detect() ~= "minecraft:air" then
turtle.dig()
turtle.forward()
else
turtle.forward()
end
if turtle.detectDown() ~= "minecraft:air" and turtle.detectDown() ~= "minecraft:stone" and X1 == true then
turtle.turnRight()
turtle.turnRight()
return
end
end
Here is an gif of what it is doing. I have realised it reacts to the stone even though I have tried telling it to ignore the stone.
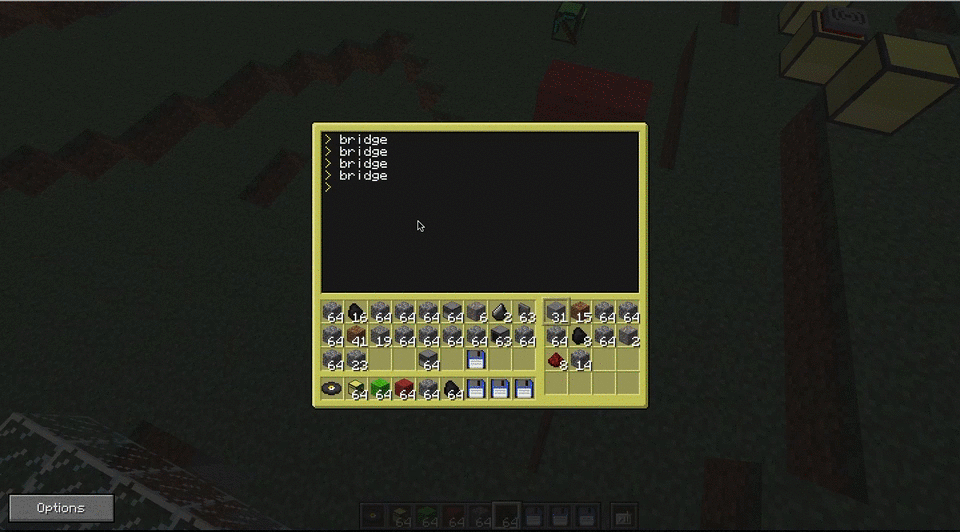
Edited on 17 July 2015 - 11:12 AM