This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
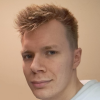
Event replacement for read()
Started by BowWhalley, 10 August 2015 - 08:57 AMPosted 10 August 2015 - 10:57 AM
Hello, I am making an OS for myself that is touchscreen and obviously uses events. I want to add a text input though with 'read()', it cant test for an event while im typing. I was wondering if someone knows of how I could use a events to simulate 'read()' without pausing the code.
Posted 10 August 2015 - 11:06 AM
Have a look at the parallel API. You can run two functions at one using it:
local result
parallel.waitForAny(
function() result = read() end,
function()
while true do
os.pullEvent("foo")
end
end
)
Posted 10 August 2015 - 09:02 PM
Have a look at the parallel API. You can run two functions at one using it:local result parallel.waitForAny( function() result = read() end, function() while true do os.pullEvent("foo") end end )
Have a look at the parallel API. You can run two functions at one using it:local result parallel.waitForAny( function() result = read() end, function() while true do os.pullEvent("foo") end end )
May you please give me more information on how I could use it in my case as I dont know if it would work with read and event listening.
Posted 10 August 2015 - 10:19 PM
The Parallel API lets you run 2 or more functions 'simultaneously'
They don't actually run together, but it makes it seem like they run 'in parallel'
It uses coroutines, which you can read up on if you'd like.
For now, just know that the Parallel API lets you run 2 functions at the same time.
Note: They both must 'yield' at some point, that is, call os.pullEvent somewhere
We are passing the VALUE of the variables (which is a function)
parallel.waitForAny runs the functions you give it until one of them finishes.
You can use 'parallel.waitForAll()' to run the functions until they all finish.
They don't actually run together, but it makes it seem like they run 'in parallel'
It uses coroutines, which you can read up on if you'd like.
For now, just know that the Parallel API lets you run 2 functions at the same time.
Note: They both must 'yield' at some point, that is, call os.pullEvent somewhere
local input
function func1() --# Doesn't really matter what they're called
input = read()
end
function func2()
while true do
local event = { os.pullEvent() }
if event[1] == "mouse_click" then
...
end
end
end
parallel.waitForAny( func1 , func2 )
Notice how there aren't parenthases when we pass 'func1' and 'func2' to the parallel api.We are passing the VALUE of the variables (which is a function)
parallel.waitForAny runs the functions you give it until one of them finishes.
You can use 'parallel.waitForAll()' to run the functions until they all finish.
Posted 11 August 2015 - 11:57 AM
This is a very basic API (thingy) which "kinda" works like the parallel API for example. You could (IMO should) look at this if you want to know how it works instead of the parallel API because parallel is more "complicated". The downside of my script is that you are not able to stop the "tasks" when one of them "dies" and then stop the others. you would have to implement that yourself.
Edited on 11 August 2015 - 06:08 PM
Posted 11 August 2015 - 04:45 PM
The downside of my script is, that you are not able to the "tasks" until only one "dies" and then stop the others…
I assume you missed a 'stop' in there…
The downside of my script is, that you are not able to stop the "tasks" until only one "dies" and then stop the others…
Posted 11 August 2015 - 07:28 PM
I've come up with using events using key and making a custom keyboard however I need to know how to test for a specific key in the 'char' event
Posted 11 August 2015 - 08:07 PM
The downside of my script is, that you are not able to the "tasks" until only one "dies" and then stop the others…
I assume you missed a 'stop' in there…The downside of my script is, that you are not able to stop the "tasks" until only one "dies" and then stop the others…
Yep, kinda. Going to rewrite that sentence now.
Posted 12 August 2015 - 02:28 AM
I realised that char is for writing a string, and only checks for certain keys. So I implemented the key char aswell.
Code used: pastebin.com/vdsJvsW9
Code used: pastebin.com/vdsJvsW9