As an example, this is a room digger code. It's basically designed such that I pick a length, and a width, and it digs out a 3-high room with these traits. Anyhow, it ran successfully down 4 rows, before suddenly breaking on the back() step in the recursion, and opting to do the turning code (for the next length to hollow out).
I took a screen to help show what I'm getting at as far as what "error" means:
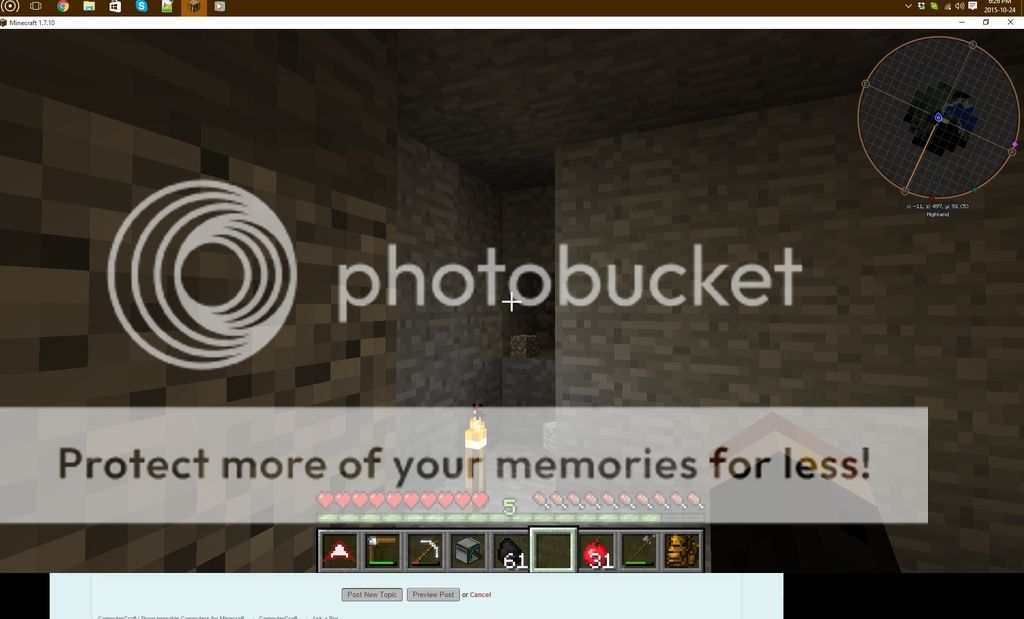
Note: far more readable version on pastebin: http://pastebin.com/vrf6S6rs
args = { ... }
-- A[1] == LENGTH, A[2] == X
function refuel()
turtle.select(1)
if turtle.refuel(0) and turtle.getFuelLevel() == 0 then
turtle.refuel(1)
return true
elseif turtle.getFuelLevel() > 0 then
return true
else
return false
end
end
function dig(counter, x)
-- dig until at length value
if counter < x then
repeat
until turtle.dig() == false and turtle.digUp() == false and turtle.digDown() == false -- in theory, deal with recurrent blocks like gravel
turtle.forward() -- move forward into the hole
dig(counter + 1, x) -- call again, pass new counter value to recursive function
turtle.back() -- as recursion resolves, move back to compensate for forwards and return to start pos
elseif x == counter then -- clear last segment before returning
turtle.digUp()
turtle.digDown()
return false
end
end
-- get turtle ready to dig another row
function prep()
turtle.turnLeft()
turtle.dig()
turtle.forward()
turtle.turnRight()
turtle.digUp()
turtle.digDown()
end
function move()
local initial = true
local length = tonumber(args[1])
local x = tonumber(args[2])
-- a note to the person kind enough to help with this: I sort of messed up the names. X is how long the room is, length is how many rows.
print("Length: ", length, " X: ", x)
turtle.up()
for i = 1, length, 1 do
if refuel() == false then
print("ERR: No fuel!")
break
end
if initial == true then
print("dig started")
dig(0, x)
initial = false
else
print("prep started")
prep()
dig(0, x)
end
end
end
move()