I have a mp world with a couple of friends where we have a big underground laboratory with different rooms and such.
At the entrance to each room I've added computers with a very simple program that shows the name of that room as text on a connected monitor.
Our doors are also opened/closed by a small cc program I wrote which just checks for a redstone input from pressure plates and then turns on/off a few drawbridges from tinker's construct.
I've now gotten that (I think at least :)/>) amazing idea to have some sort of Main Control room where I have a computer which is connected via rednet to all the other computers and which is able
to change the text on each monitor and lock the doors if need be. Think; Laboratory Lockdown - Nobody enters/leaves! (I like giving my minecraft builds some life/story behind it :D/>)
I've been trying hard to figure out how to do this best with my very limited knowledge with CC and Lua.
One thing I have a hard time figuring out is that when my doors is looking for a redstone event all the time, how will it be able to get commands from a remote computer?
So my big question is if some of you guys could help me out, and give me some pointers to what kind of stuff I need to look into to make this possible.
I'm not asking for a finished program that does exactly what I'm talking about, but maybe some links to good tutorials about this stuff, or perhaps even some finished programs some of you/others already made
that would work with a few tweaks.
Just about anything would be a huge help :)/>
Oh, and btw these a the two simple programs I made to control the doors and display the text on the monitors if you need to see those:
Pretty horrific stuff I know! :P/>
Doors
rs.setOutput("left", true)
rs.setOutput("right", true)
while true do
--wait for a redstone event.
local event = os.pullEvent("redstone")
--test for input
local BState=rs.getInput("back")
local FState=rs.getInput("front")
--if true
if BState or FState then
rs.setOutput("left", false)
rs.setOutput("right", false)
sleep(3)
rs.setOutput("left", true)
rs.setOutput("right", true)
sleep(3)
end
end
Monitors
monitors = {
peripheral.wrap("left");
peripheral.wrap("right");
--For monitors on each side of computer
}
function allMonitorsClear()
for i=1,#monitors do
monitors[i].clear()
end
end
function header(height, text, tSize, color)
for i=1,#monitors do
w, h = monitors[i].getSize()
monitors[i].setTextScale(tSize)
monitors[i].setTextColor(color)
monitors[i].setCursorPos((w-string.len(text))/2+1, height)
monitors[i].write(text)
end
end
--placeholder is needed for first line (cant figure why?)
--everytime I log in or server restarts the first line is all broken without it
--Seems like it have something to do with w-string.len(text))/2+1
header(1, "ph", 2, colors.lime)
allMonitorsClear()
header(1, "AE2", 2, colors.lime)
header(2, "Control", 2, colors.lime)
Sorry about any poor grammar, English isn't my native language :)/>
Thanks,
-todo
Here is a few screenies so you have an idea of what I am talking about:
A few of the monitors I would love to be able to change the text on when in "lockdown" :D/>, including the big monitor which is just running a "screensaver" I found on these forums a while back
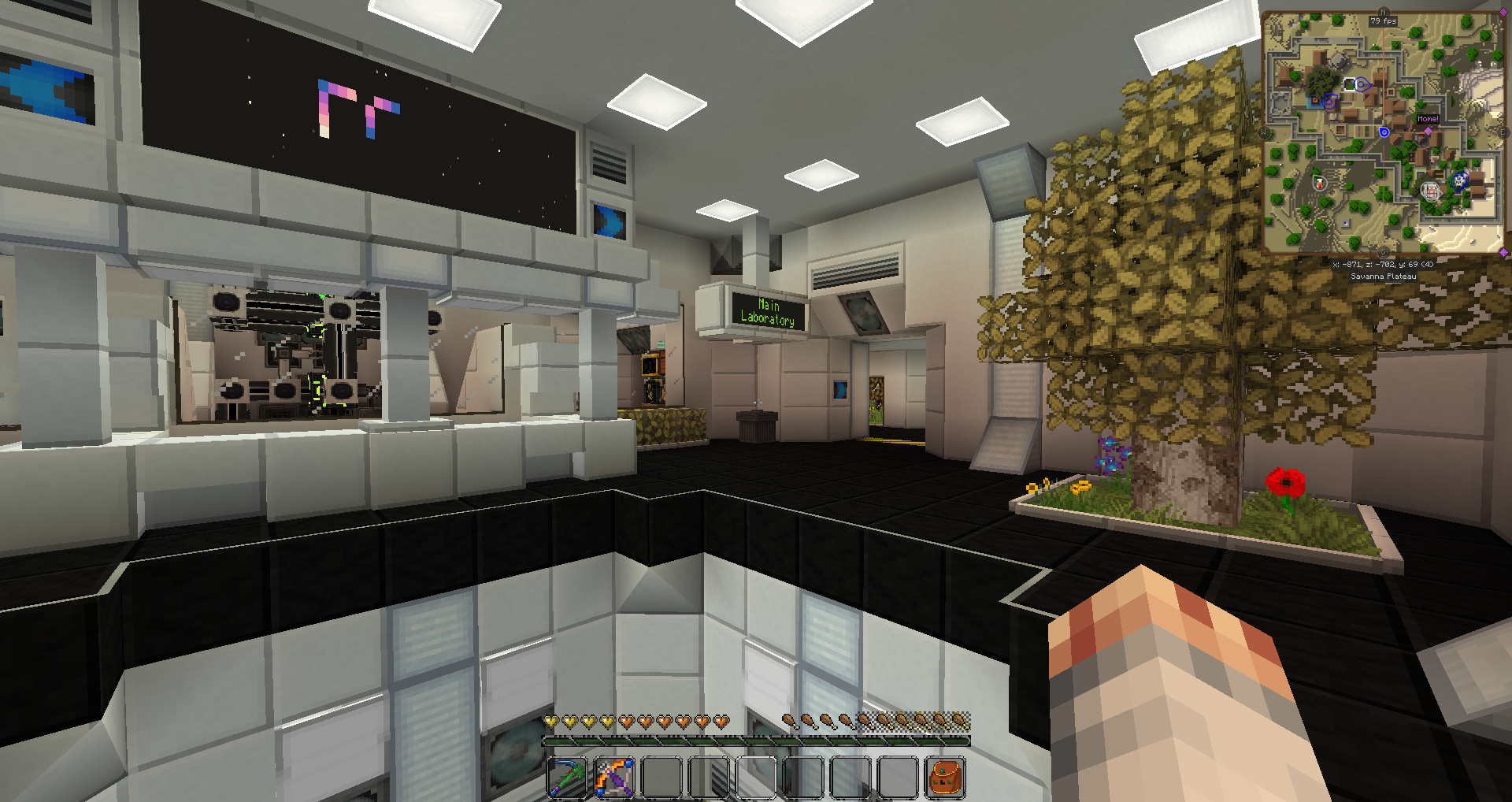
A couple of monitors down a hall
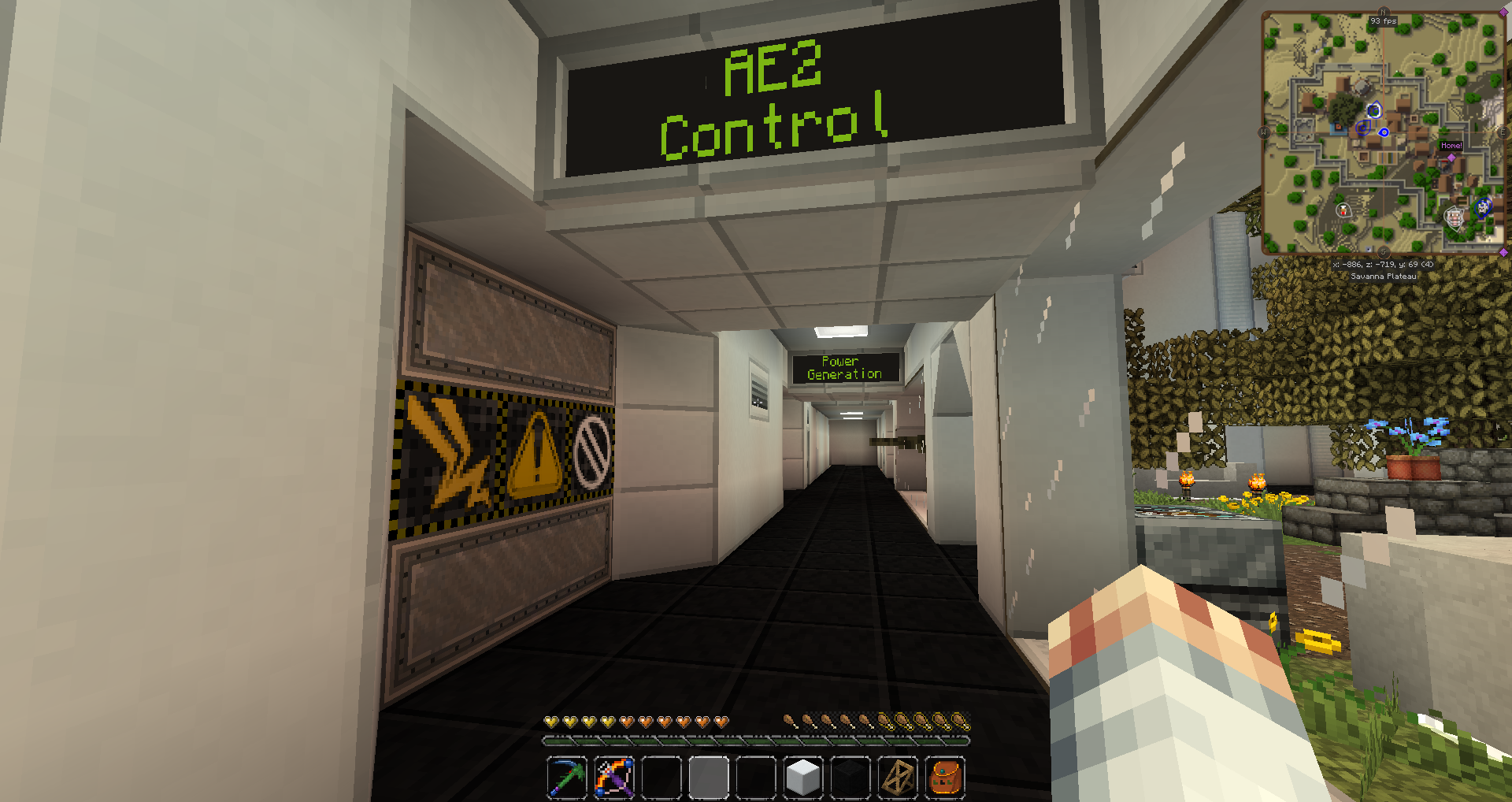
And this is the door using drawbridges which I would like to be able to lock from the remote computer
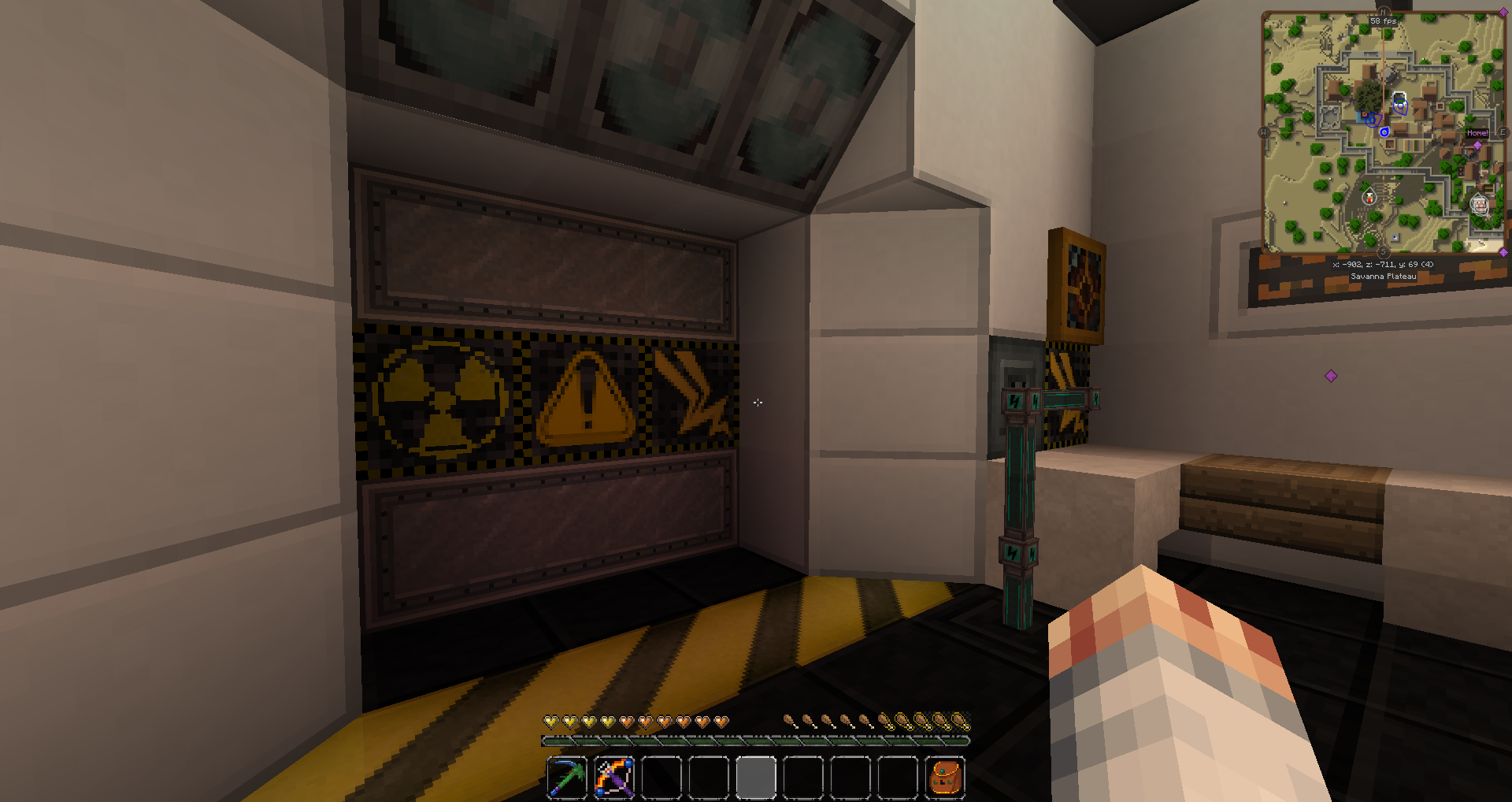