This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
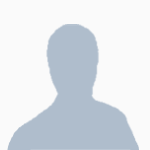
String breaking & duplicate check
Started by MrScissors, 11 November 2015 - 09:44 AMPosted 11 November 2015 - 10:44 AM
Hello. So i am writing a program that requires me to check if there are any repeating characters in string (error). Also, i need to know a way how to break string into array of characters. Anyone can help?
Posted 11 November 2015 - 10:53 AM
To break up a string you can do:
usernameLetters = {} --# Stores the individual letter for the broken up name
function breakString(username) --# This Breaks up the string
for i = 1, username:len() do --# username is a string value
table.insert(usernameLetters, username:sub(i, i))
end
end
Posted 11 November 2015 - 10:56 AM
Yep, you have to use string.sub and iterate through all the characters of the string.
Hope I helped.
Damn it, ninja'd
local array = {}
local text = "Some stuff here!"
for i=1,#text do -- the iteration
array[#array+1] = text:sub(i,i) -- take the character at the position
end
Hope I helped.
Damn it, ninja'd
Posted 11 November 2015 - 11:09 AM
Yep, you have to use string.sub and iterate through all the characters of the string.local array = {} local text = "Some stuff here!" for i=1,#text do -- the iteration array[#array+1] = text:sub(i,i) -- take the character at the position end
Hope I helped.
Damn it, ninja'd
Thanks. But i have second question. How to now check for duplicates in received array?
Posted 11 November 2015 - 11:19 AM
Well again, you should iterate (What a coincidence I am in IT class and learning about iterations in C++)
If you want to eliminate duplicates:
If you want to eliminate duplicates:
array -- the array from the prev example
local chars = {}
local newArray = {}
for i=1,#array do
local current = array[i]
if not chars[current] then
char[current] = true
newArray[#newArray+1] = current
end
end
Posted 11 November 2015 - 12:05 PM
And newArray will contain all filtered characters?Well again, you should iterate (What a coincidence I am in IT class and learning about iterations in C++)
If you want to eliminate duplicates:array -- the array from the prev example local chars = {} local newArray = {} for i=1,#array do local current = array[i] if not chars[current] then char[current] = true newArray[#newArray+1] = current end end
Posted 11 November 2015 - 12:12 PM
Yes it will
Posted 11 November 2015 - 05:56 PM
Just for completeness, here is Another way to split a string:
local array = {}
local str = "YourStringHere"
for char in str:gmatch(".") do
table.insert(array,char)
end