Posted 29 November 2015 - 09:26 AM
Hello!
Overview:
I am a okay leveled programmer and have basically been writing scripts for ICBM mod.
While doing so i discovered that the actual ICBM block radar doesn't display targets well.
So i decided to come up with my own CC interface that uses radar data to plot points.
Basically its supposed to make a circle that has a rotating line within it.
The actual radar look, (kind of like a fan with a slow single blade rotating).
The screen looks like the following right now:
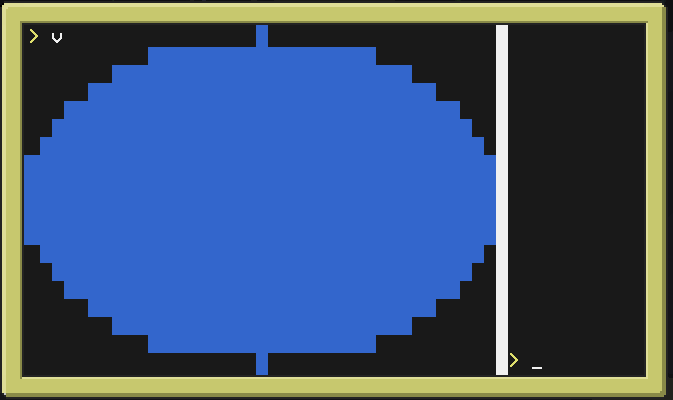
The circle is where points will be plotted and also where the line will rotate.
The white line on the right separates the radar from a list of incoming targets
My Actual Question:
I was wondering if anyone knew how to well do the following:
1. Plot points on the circle.
2. Have a white line rotate in the circle.
Program:
http://pastebin.com/TFZuGWYx
Many Thanks!!
~Andrew2060
Overview:
I am a okay leveled programmer and have basically been writing scripts for ICBM mod.
While doing so i discovered that the actual ICBM block radar doesn't display targets well.
So i decided to come up with my own CC interface that uses radar data to plot points.
Basically its supposed to make a circle that has a rotating line within it.
The actual radar look, (kind of like a fan with a slow single blade rotating).
The screen looks like the following right now:
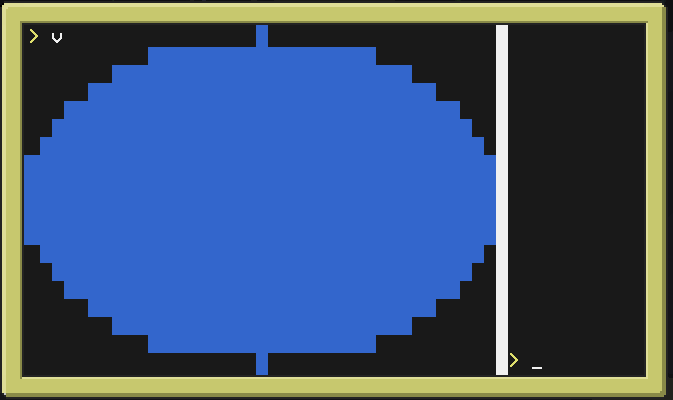
The circle is where points will be plotted and also where the line will rotate.
The white line on the right separates the radar from a list of incoming targets
My Actual Question:
I was wondering if anyone knew how to well do the following:
1. Plot points on the circle.
2. Have a white line rotate in the circle.
Program:
http://pastebin.com/TFZuGWYx
Many Thanks!!
~Andrew2060