This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
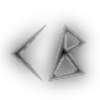
FS question
Started by TyDoesMC, 28 January 2016 - 04:28 PMPosted 28 January 2016 - 05:28 PM
Hello, I am getting back into CC and I need some help for a project I am making. I would like to have config files EG: MyProgram.cfg and then read certain lines and have then do specific functions. I would like to have strings, booleans, Int etc.
Posted 28 January 2016 - 05:51 PM
Simple enough to do, we do not normally write code for people but more point them in the right direction… So read up on the below:
FS API: http://www.computercraft.info/wiki/Fs_(API)
Textutils API: http://www.computercraft.info/wiki/Textutils_(API)
Lua Tables: http://www.lua.org/pil/2.5.html
Once you have done this, then you can understand how this would work. So I shall explain the process, in your program you can have a table for example:
Then you want to give it some data that you wish to be stored in the configuration file, so maybe the name of the program (Only an example, you can put whatever you want in this - other than functions):
Boom now that is saved to file.
To open it back up and get the data, you will technically reverse the above steps, but you will open the file in read mode, not write mode:
And so you can recap here is the code above but in functions:
To use that code above, paste it at the top of your program to make sure Lua registers the function then say your config table is called "ConfigTable" and do the following:
To load it do:
Hope that helps, any questions send me a PM! :D/>
FS API: http://www.computercraft.info/wiki/Fs_(API)
Textutils API: http://www.computercraft.info/wiki/Textutils_(API)
Lua Tables: http://www.lua.org/pil/2.5.html
Once you have done this, then you can understand how this would work. So I shall explain the process, in your program you can have a table for example:
myconfig = {}
Then you want to give it some data that you wish to be stored in the configuration file, so maybe the name of the program (Only an example, you can put whatever you want in this - other than functions):
myconfig["program_name"] = "My program"
myconfig.program_name = "My program"
Both lines above do the same thing. Once you have some data you will want to save it, so you need to open a file like so:local configFile = fs.open("MyProgram.cfg", "w")
Above we have opened a file in write mode, meaning we can write to the file, there are other modes, detailed in the FS api documentation. Now let's get our config table and we need to make a string so we can save it to a file, this we can do with the textutils api:local stringConfig = textutils.serialize(myconfig)
Now that is saved as we have serialized the data in the table to a string so now let's save it to the file, remember the file has a handle which is "configFile" so you do:configFile.write(stringConfig)
This has now written the string version of your config table into the file, now always make sure to close the file / handle:configFile.close()
Boom now that is saved to file.
To open it back up and get the data, you will technically reverse the above steps, but you will open the file in read mode, not write mode:
local configFile = fs.open("MyProgram.cfg", "r")
Now lets read all the data and unserialize (convert it from string back to a table):local fileData = configFile.readAll()
local myconfig = textutils.unserialize(fileData)
Now your table is back in the "myconfig" variable, DON'T forget to close the file again:configFile.close()
And so you can recap here is the code above but in functions:
function saveConfig(configTable, fileName)
local f = fs.open(fileName, "w")
f.write(textutils.serialize(configTable))
f.close()
return true
end
function loadConfig(fileName)
local f = fs.open(fileName, "r")
local config = f.readAll()
f.close()
return config
end
To use that code above, paste it at the top of your program to make sure Lua registers the function then say your config table is called "ConfigTable" and do the following:
local ConfigTable = {
["Name"] = "My Program";
}
if saveConfig(ConfigTable, "MyProgram.cfg") then
print("Saved")
else
print("Could not save")
end
To load it do:
local config_table = loadConfig("MyProgram.cfg")
Hope that helps, any questions send me a PM! :D/>
Posted 28 January 2016 - 06:21 PM
It may not be related to file functions, BUT it is a new way of saving settings
There is a new API in CC 1.78, the Settings API, and it has a program the user can use to set and get value's:
I am really trying to get people to use this API as its a nice way of giving users options, especially with a tool that allows to visually edit settings
There is a new API in CC 1.78, the Settings API, and it has a program the user can use to set and get value's:
settings.set("MyProgramName_SettingName", value)
local val = settings.get("MyProgramName_SettingName", default value)
you can also use a diffrent settings file using this API, wiki: http://www.computerc.../Settings_(API)I am really trying to get people to use this API as its a nice way of giving users options, especially with a tool that allows to visually edit settings
Edited on 28 January 2016 - 05:28 PM
Posted 28 January 2016 - 07:38 PM
It may not be related to file functions, BUT it is a new way of saving settings
There is a new API in CC 1.78, the Settings API, and it has a program the user can use to set and get value's:you can also use a diffrent settings file using this API, wiki: http://www.computerc.../Settings_(API)settings.set("MyProgramName_SettingName", value) local val = settings.get("MyProgramName_SettingName", default value)
I am really trying to get people to use this API as its a nice way of giving users options, especially with a tool that allows to visually edit settings
Yes but remember there are versions of CC that do not have that, and this guy might still be using. < 1.78
Posted 28 January 2016 - 10:21 PM
Ok I understand most of that. how would I go about using functions based on whats in the config?
Posted 29 January 2016 - 12:07 AM
Ok I understand most of that. how would I go about using functions based on whats in the config?
What do you mean? Can you give me an example of what you are trying to achieve?
Posted 29 January 2016 - 12:41 AM
Ok I understand most of that. how would I go about using functions based on whats in the config?
We aren't really sure to begin since we don't know how well you know Lua.
Do you have any code? Maybe we could take a peek at it and tell how you integrate this into it.
Posted 29 January 2016 - 09:11 AM
I think this has been said before on this thread, but the easiest way that I can think of this is to dump all your settings data in a table and serialise it to a file.
Loading is then reading the file and un-serialising the table.
If you need an example then (the beta version of) QuadPipe does this. http://www.computerc...ment-made-easy/
Edit: I evidently can't recall how my own code works, QuadPipe doesn't load stuff from a file like this.
Loading is then reading the file and un-serialising the table.
Edit: I evidently can't recall how my own code works, QuadPipe doesn't load stuff from a file like this.
Edited on 29 January 2016 - 08:25 AM
Posted 29 January 2016 - 10:45 AM
local function loadData(fname)
local f = fs.open(fname, "r")
local data = textutils.desrialize(f.readAll())
f.close()
return data
end
local function saveData(fname, tdata)
local f = fs.open(fname, "w")
f.write(texturils.serialize(tdata)
f.close()
end
this is just a little example of how simple saving and loading a table can be
These functions will throw errors if not used properly, handle errors yourself,