My overall goal with this program was to make a turtle that would go through a 7x7 farm of wheat in a clockwise spiral, scanning to see if the wheat was grown before farming it, and then returning to a final position and crafting bread, then dropping its contents and refueling if need be. However, while i fixed several of the issues that arised, i still have a few.
When it does its initial movement into the field, it doesnt attempt to farm, it makes its turn, scans and farms properly. But then when it moves again it goes forward 2 blocks rather than 1. I have no idea as to why it does this because when I look at the code i see no reason it should move twice, and without farming non the less.
This is a picture of the farm, turtle placement, and path it should take.
Spoiler
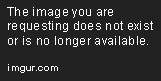
My code:
Spoiler
--[[aWheatFarm]]--
--[[By: ThePhoenixSol]]--
--[[VARIABLES]]--
local fuelLevel = turtle.getFuelLevel()
local success, data = turtle.inspectDown()
local cycle = true
local cyc = 6
local cycTwo = 4
local cycThree = 2
local incNum = 0
--[[FUNCTIONS]]--
function refuel()
if fuelLevel < 300 then
turtle.turnRight()
turtle.select(1)
turtle.suck()
turtle.refuel()
turtle.turnLeft()
end
end
function InvEmp()
for i = 1, 4 do
turtle.select(i)
turtle.dropDown()
end
for i = 8, 16 do
turtle.select(i)
turtle.dropDown()
end
turtle.select(8)
turtle.dropDown()
end
function craft()
turtle.turnLeft()
turtle.select(1)
turtle.drop()
turtle.select(2)
turtle.drop()
InvEmp()
turtle.select(16)
turtle.craft()
turtle.dropDown()
turtle.select(1)
turtle.suck()
turtle.select(2)
turtle.suck()
turtle.turnRight()
end
function replant()
turtle.select(2)
turtle.suckDown(64)
turtle.placeDown()
end
function harvest()
for i = 5, 7 do
if turtle.getItemCount(i) > 64 then
turtle.select(i)
end
if data.metadata == 7 then
turtle.digDown()
end
end
end
function forwFarm()
turtle.forward()
if data.metadata == 7 then
os.sleep(5)
harvest()
os.sleep(5)
replant()
end
end
function rightForwLayOne()
turtle.turnRight()
while cyc > incNum do
forwFarm()
os.sleep(5)
incNum = incNum + 1
end
incNum = 0
end
function rightForwLayTwo()
turtle.turnRight()
while cycTwo > incNum do
forwFarm()
os.sleep(5)
incNum = incNum + 1
end
incNum = 0
end
function rightForwLayThree()
turtle.turnRight()
while cycThree > incNum do
forwFarm()
os.sleep(5)
incNum = incNum + 1
end
incNum = 0
end
function returnHome()
turtle.turnRight()
turtle.forward()
turtle.turnLeft()
turtle.forward()
turtle.forward()
turtle.forward()
turtle.down()
turtle.turnLeft()
turtle.turnLeft()
end
function status()
turtle.up()
forwFarm()
turtle.turnLeft()
forwFarm()
forwFarm()
forwFarm()
rightForwLayOne()
rightForwLayOne()
rightForwLayOne()
turtle.turnRight()
forwFarm()
forwFarm()
forwFarm()
turtle.turnRight()
forwFarm()
turtle.turnLeft()
forwFarm()
forwFarm()
rightForwLayTwo()
rightForwLayTwo()
rightForwLayTwo()
turtle.turnRight()
forwFarm()
forwFarm()
turtle.turnRight()
forwFarm()
turtle.turnLeft()
forwFarm()
rightForwLayThree()
rightForwLayThree()
rightForwLayThree()
returnHome()
end
--[[MAIN]]--
while cycle do
refuel()
status()
craft()
end
If you need any other information to assist me, merely ask and I will do what I can to help.