int, rest = restDivision(50, 8)
-- int --> 6
-- rest -- > 0.25
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
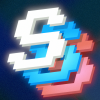
How can I get the decimal place after a divison
Started by Sewbacca, 04 April 2016 - 04:42 PMPosted 04 April 2016 - 06:42 PM
For example:
Edited on 04 April 2016 - 05:14 PM
Posted 04 April 2016 - 06:49 PM
local function intDiv(a, B)/>
local div = a / b
local round = div < 0 and math.ceil(div) or math.floor(div)
return round, div - round
end
intDiv(50, 8) -- 6, 0.25
intDiv(-50, 8) -- -6, -0.25 -- You can always do math.abs on the rest to get the positive version
The div < 0 and math.ceil(div) or math.floor(div) gets the integer part always rounded towards 0 (as Lua as no function to do this).
Edited on 04 April 2016 - 04:50 PM
Posted 04 April 2016 - 06:57 PM
thanks
Posted 05 April 2016 - 01:03 AM
Modulus works just as well.
local function restDivision(numerator,divisor)
--#Grab just the pure division, since that's going to be int plus rest
local int = numerator/divisor
--#Grab our decimal, this is your rest
local decimal = int % 1
--#Remove the decimal from int
int = int - decimal
--#return
return int,decimal
end
Posted 05 April 2016 - 01:15 AM
You'd think so, but that code fails for negative numerators.
Posted 05 April 2016 - 01:44 AM
True. I'm assuming you're meaning that the -decimal part would subtract on a negative and not work correctly?
local function restDivision(numerator,divisor)
--#Grab just the pure division, since that's going to be int plus rest
local int = numerator/divisor
--#Grab our decimal, this is your rest
local decimal = int % 1
--#Remove the decimal from int
--#the and or parts is lua ternary.
--#Basically if int > 0 then int = int - decimal
--#else int = int + decimal
int = int > 0 and int - decimal or int + decimal
--#return
return int,decimal
end
Posted 05 April 2016 - 01:56 AM
Give it a test and see. :P/>
print(restDivision(-50, 8)) --> ???
Posted 05 April 2016 - 03:28 AM
*sigh* but then I have to load up minecraft again.
Why, oh why, would this do THAT.
Thanks for the test case BB, that's an annoying as hell way to do that.
Edit: For those wondering ( -6.25 % 1 == 0.75)
Why, oh why, would this do THAT.
Thanks for the test case BB, that's an annoying as hell way to do that.
Edit: For those wondering ( -6.25 % 1 == 0.75)
local function restDivision(numerator,divisor)
--#Grab just the pure division, since that's going to be int plus rest
local int = numerator/divisor
--#Grab our decimal, this is your rest
--#Woo, doing the ternary HERE
local decimal = int % (int > 0 and 1 or -1)
int = int - decimal
--#return
return int,decimal
end
Edited on 05 April 2016 - 01:30 AM