Posted 28 July 2016 - 12:35 AM
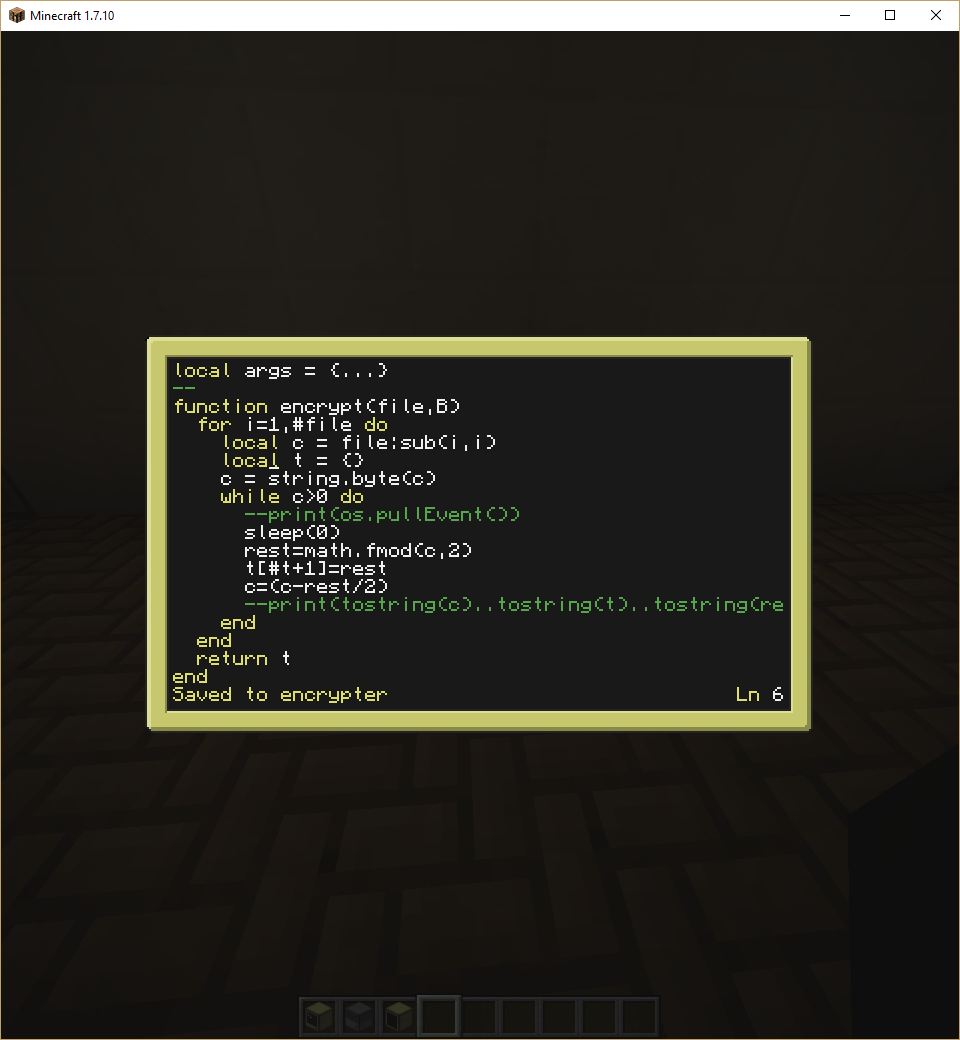
file is the input, B does nothing ignore B, file is put it then we iterate and get the decimal, and the problem is that i cant get the binary of the decimal
send help SOS
local highestNumIntoX = math.floor(math.log(x) / math.log(2))
function toBits(n)
local o = {}
repeat
o[#o+1] = n%2
n = bit32.rshift(n, 1)
until n == 0
return o
end
a = toBits(32) will return {0,0,0,0,0,1}, basically a[ j ] == 1 if the bit of value 2^j is 1.