2 posts
Posted 29 July 2016 - 07:39 AM
I'm trying to create an automatic strip mining turtle that returns to home when it runs out of fuel.
It determines how many strips to make and for what length each via tArgs
As soon as I run the program with any arguments, it gives me the java.lang.ArrayIndexOutOfBoundsException error. To the best of my knowledge, this is commonly caused by recursive functions that call upon itself or incorrectly utilized infinite loops. However my program does not use either of these so I am left scratching my head.
The code can be located here:
http://pastebin.com/N5TQnAshHere is a screenshot of the error itself in minecraft:
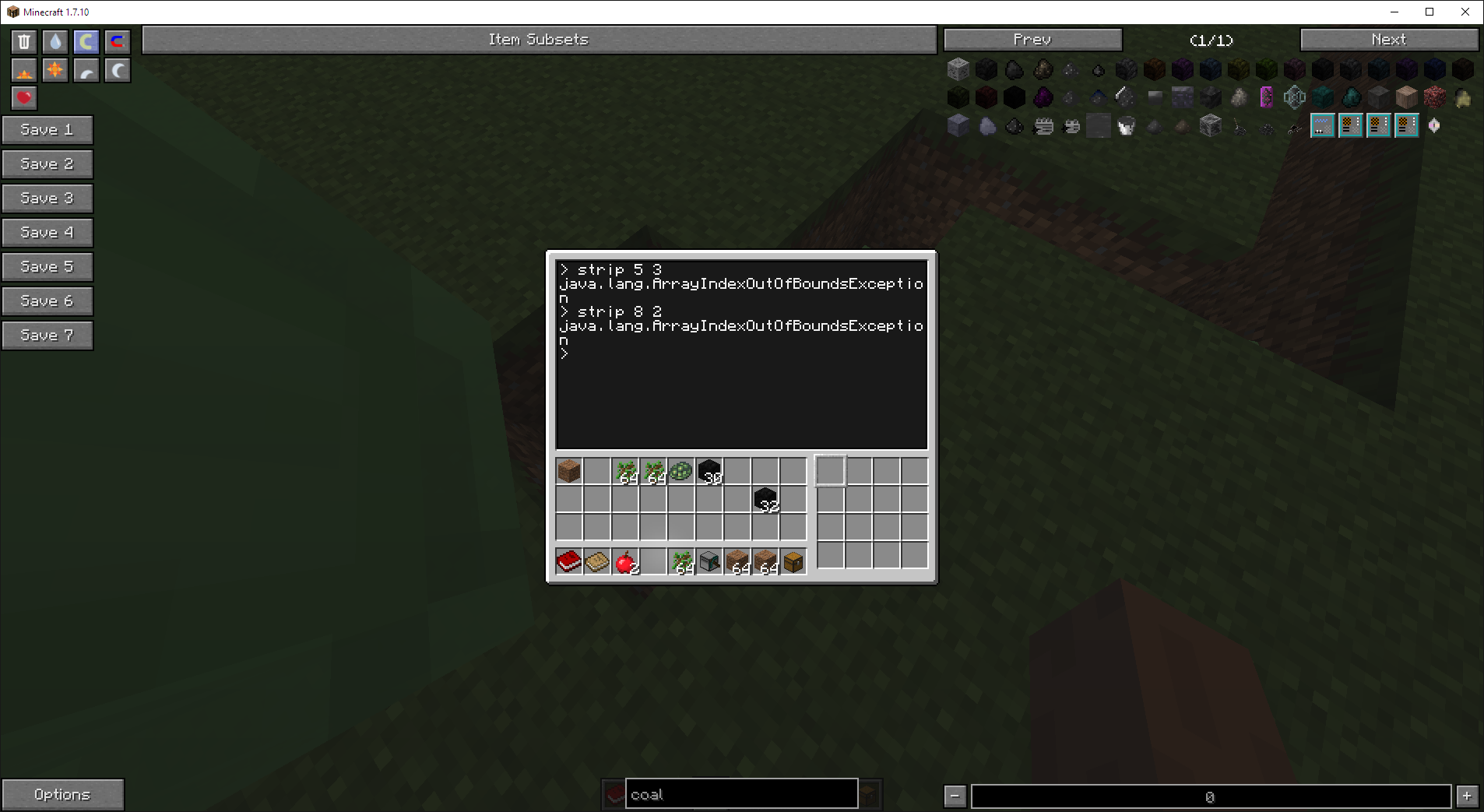
Any other suggestions to my code would be cool as well
3057 posts
Location
United States of America
Posted 29 July 2016 - 08:29 PM
The problem is in your goTo function, as it calls itself.
function goTo(x,z)
local distanceToTarget = xPos-x+zPos-z
if canRTH() and distanceToTarget+2<turtle.getFuelLevel() then
if z>zPos then
faceForward()
for i=1,z-zPos do
if turtle.detect() then
turtle.dig()
end
moveForward()
if turtle.detectUp() then
turtle.digUp()
end
turtle.suck()
zPos=zPos+1
print("forwards1")
end
end
if z<zPos then
faceBackward()
for i=1,zPos-z do
if turtle.detect() then
turtle.dig()
end
moveForward()
if turtle.detectUp() then
turtle.digUp()
end
turtle.suck()
zPos=zPos-1
print("backwards1")
end
end
if x>xPos then
faceRight()
for i=1,x-xPos do
if turtle.detect() then
turtle.dig()
end
moveForward()
if turtle.detectUp() then
turtle.digUp()
end
turtle.suck()
xPos=xPos+1
print("right1")
end
end
if x<xPos then
faceLeft()
for i=1,xPos-x do
if turtle.detect() then
turtle.dig()
end
moveForward()
if turtle.detectUp() then
turtle.digUp()
end
turtle.suck()
xPos=xPos-1
print("left1")
end
end
else
local lastX,lastZ=xPos,zPos
returnToHome() --# << returnToHome calls goTo, RECURSIVE FUNCTION CALL
print("I need fuel in slot 1, then press enter")
io.read()
turtle.select(1)
turtle.refuel()
print("Resuming..")
goTo(lastX,lastZ) --# <<<< RECURSIVE FUNCTION CALL
end
end
In fact, what I think happens is the turtle has no fuel, so the goTo function calls returnToHome, which calls goTo, which (since you still have no fuel) calls returnToHome… etc.
Edited on 29 July 2016 - 06:32 PM
2 posts
Posted 30 July 2016 - 05:03 PM
Thank you very much, I didn't catch that!
I made a boolean for it to be able to tell if it needs to returnToHome so that way the goTo function doesn't force itself to returnToHome when called by the returnToHome function…if that makes sense
Here are the updated parts:
local tArgs = {...}
if #tArgs ~= 2 then
print("Usage: strip <# of strips> <strip depth>")
return
end
local stripCount=tonumber(tArgs[1])
local stripDepth=tonumber(tArgs[2])
local heading="forward"
local xPos,zPos=0,0
local returningToHome=false -- added a boolean to keep it from asking for more fuel while returning home
...
function returnToHome()
returningToHome=true --new line
goTo(0,zPos)
goTo(0,0)
faceLeft()
for i=1,16 do
turtle.select(i)
turtle.drop()
end
turtle.select(1)
faceForward()
returningToHome=false --new line
end
It now works exactly as intended, thank you for the help!