This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
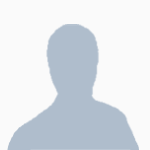
Using HTTP to gather a variable in php to process in lua
Started by Bubbycolditz, 08 July 2017 - 11:14 PMPosted 09 July 2017 - 01:14 AM
I need help to when I connect to a http website, i can gather a variable in the php code and then use it in lua. How can I do this?
Posted 09 July 2017 - 01:36 AM
Lua side:
PHP:
local h = http.get( "your link here" )
print( h.readAll() )
h.close()
PHP:
echo "Hello World";
Posted 09 July 2017 - 02:17 AM
Lua side:local h = http.get( "your link here" ) print( h.readAll() ) h.close()
PHP:echo "Hello World";
No such as if i had a variable named updatedVersion and currentVersion. Then i say if currentVersion is less than updatedVersion it does so and so. How do i do that?
Posted 09 July 2017 - 02:56 AM
Lua side:
PHP:
local h = http.get( "your link here" )
print( h.readAll() == "1.0" )
h.close()
PHP:
echo "1.1";
Posted 09 July 2017 - 03:01 AM
Lua side:local h = http.get( "your link here" ) print( h.readAll() == "1.0" ) h.close()
PHP:echo "1.1";
EDIT: This code does not work. All it says is False all the time when I test this and I have no clue on where i can insert code to where a pastebin can run when there is a update.
Edited on 09 July 2017 - 04:48 PM
Posted 09 July 2017 - 07:58 PM
Your PHP page needs to echo the variable's value, so that it's part of the page content when you fetch it from ComputerCraft. You then make your comparison on the Lua end.
Posted 10 July 2017 - 12:10 AM
The code sample provided by KingofGamesYami should work fine. The one issue with that one is that it'll, in addition to returning false if the current version number is below the latest version, it'll also say if the current version number is above the latest version. This, in many cases, won't be an issue, but if you do push the program version above the one that's being returned by the website, it will in fact appear to be out of date. A way to prevent this is by using tonumber() to turn the version into a number before comparison and using >=/<=, though note this obviously won't work correctly if your version is laid out like 1.0.1.
(Note: Even in that case, you could remove all of the '.' in lua; though this would mean you'd have to keep the format consistent. Ie, you couldn't move from 1.0 to 1.0.1 or 1.0.0 to 1.0.01 without potentially causing issues. (like from going from 1.0.11 from 1.0.2, you'd have to put 1.0.20 as it'd be comparing 1011 to 102)
I've set up these two samples to hopefully demonstrate this & help your understanding:
First webpage
https://cc.tylertwin...var/1point0.php
Second webpage
https://cc.tylertwin...var/1point1.php
Lua:
pastebin run 1VZga5iN
This will check both versions and tell if they're up to date with the default version (1.0). You can additionally run `pastebin run 1VZga5iN 1.1` and you'll see the issue that the 1.0 webpage will return false, as if it's out of date when the client would technically be ahead.
The "Content-Type" headers are not very necessary. It's more used to make it more viewable in a webpage (and technically more correct), because it sees it as a text document instead of an HTML page.
(Note: Even in that case, you could remove all of the '.' in lua; though this would mean you'd have to keep the format consistent. Ie, you couldn't move from 1.0 to 1.0.1 or 1.0.0 to 1.0.01 without potentially causing issues. (like from going from 1.0.11 from 1.0.2, you'd have to put 1.0.20 as it'd be comparing 1011 to 102)
I've set up these two samples to hopefully demonstrate this & help your understanding:
First webpage
https://cc.tylertwin...var/1point0.php
<?php
header("Content-Type: text/plain");
echo "1.0";
?>
Second webpage
https://cc.tylertwin...var/1point1.php
<?php
header("Content-Type: text/plain");
echo "1.1";
?>
Lua:
pastebin run 1VZga5iN
This will check both versions and tell if they're up to date with the default version (1.0). You can additionally run `pastebin run 1VZga5iN 1.1` and you'll see the issue that the 1.0 webpage will return false, as if it's out of date when the client would technically be ahead.
The "Content-Type" headers are not very necessary. It's more used to make it more viewable in a webpage (and technically more correct), because it sees it as a text document instead of an HTML page.
Edited on 09 July 2017 - 10:17 PM
Posted 17 July 2017 - 04:21 AM
You can parse queries to the http server containing what variable you want to get, example:
Lua
PHP File
Output
Lua
local protocol, domain, port, file, query = 'http://', 'localhost', ':80/', 'lua-request.php?', 'version=1.0'
local handle = http.get(protocol .. domain .. port .. file .. query);
local txt = handle.readAll();
handle.close();
print(txt);
PHP File
<?php
$versions = [
'1.0' => 'Version 1.0 is an old version',
'1.1' => 'Version 1.1 is a new version'
];
echo $versions[$_GET['version']];
Output
Version 1.0 is an old version!
Posted 07 August 2017 - 06:01 AM
EDIT: When i try using your method, it prints them at the same time for both versions being out-of-date. I copied everything that you have written down and put it in my files and they do not work. Once this does work, I'll give you full credit for this. :)/>The code sample provided by KingofGamesYami should work fine. The one issue with that one is that it'll, in addition to returning false if the current version number is below the latest version, it'll also say if the current version number is above the latest version. This, in many cases, won't be an issue, but if you do push the program version above the one that's being returned by the website, it will in fact appear to be out of date. A way to prevent this is by using tonumber() to turn the version into a number before comparison and using >=/<=, though note this obviously won't work correctly if your version is laid out like 1.0.1.
(Note: Even in that case, you could remove all of the '.' in lua; though this would mean you'd have to keep the format consistent. Ie, you couldn't move from 1.0 to 1.0.1 or 1.0.0 to 1.0.01 without potentially causing issues. (like from going from 1.0.11 from 1.0.2, you'd have to put 1.0.20 as it'd be comparing 1011 to 102)
I've set up these two samples to hopefully demonstrate this & help your understanding:
First webpage
https://cc.tylertwin...var/1point0.php<?php header("Content-Type: text/plain"); echo "1.0"; ?>
Second webpage
https://cc.tylertwin...var/1point1.php<?php header("Content-Type: text/plain"); echo "1.1"; ?>
Lua:
pastebin run 1VZga5iN
This will check both versions and tell if they're up to date with the default version (1.0). You can additionally run `pastebin run 1VZga5iN 1.1` and you'll see the issue that the 1.0 webpage will return false, as if it's out of date when the client would technically be ahead.
The "Content-Type" headers are not very necessary. It's more used to make it more viewable in a webpage (and technically more correct), because it sees it as a text document instead of an HTML page.
Edited on 07 August 2017 - 04:02 AM
Posted 07 August 2017 - 11:27 AM
It might be helpful to print out the actual result. It's possible something interesting is happening, like an extra character (a space or a return or something like that).
Posted 07 August 2017 - 07:53 PM
The code sample provided by KingofGamesYami should work fine. The one issue with that one is that it'll, in addition to returning false if the current version number is below the latest version, it'll also say if the current version number is above the latest version. This, in many cases, won't be an issue, but if you do push the program version above the one that's being returned by the website, it will in fact appear to be out of date. A way to prevent this is by using tonumber() to turn the version into a number before comparison and using >=/<=, though note this obviously won't work correctly if your version is laid out like 1.0.1.
(Note: Even in that case, you could remove all of the '.' in lua; though this would mean you'd have to keep the format consistent. Ie, you couldn't move from 1.0 to 1.0.1 or 1.0.0 to 1.0.01 without potentially causing issues. (like from going from 1.0.11 from 1.0.2, you'd have to put 1.0.20 as it'd be comparing 1011 to 102)
I've set up these two samples to hopefully demonstrate this & help your understanding:
First webpage
https://cc.tylertwin...var/1point0.php<?php header("Content-Type: text/plain"); echo "1.0"; ?>
Second webpage
https://cc.tylertwin...var/1point1.php<?php header("Content-Type: text/plain"); echo "1.1"; ?>
Lua:
pastebin run 1VZga5iN
This will check both versions and tell if they're up to date with the default version (1.0). You can additionally run `pastebin run 1VZga5iN 1.1` and you'll see the issue that the 1.0 webpage will return false, as if it's out of date when the client would technically be ahead.
The "Content-Type" headers are not very necessary. It's more used to make it more viewable in a webpage (and technically more correct), because it sees it as a text document instead of an HTML page.
Alternatively, you can use string.gmatch and compare each version number individually. This works with strings like "1.0.1".
function isOutdated(current, remote)
local cfunc = current:gmatch("%d+")
local rfunc = remote:gmatch("%d+")
local cnum = tonumber(cfunc())
local rnum = tonumber(rfunc())
while cnum and rnum do
if cnum ~= rnum then
return cnum < rnum
end
cnum = tonumber(cfunc())
rnum = tonumber(rfunc())
end
return rnum ~= nil
end