You can use the dot product! Dot products are awesome. It returns the cosine of the angle between two vectors. That might not mean anything on its own, but it's insanely useful because…
The angle between the vectors will be between 0 and 180 degrees. The cosine of an angle less than 90 degrees will be positive, and greater than 90 degrees will be negative.
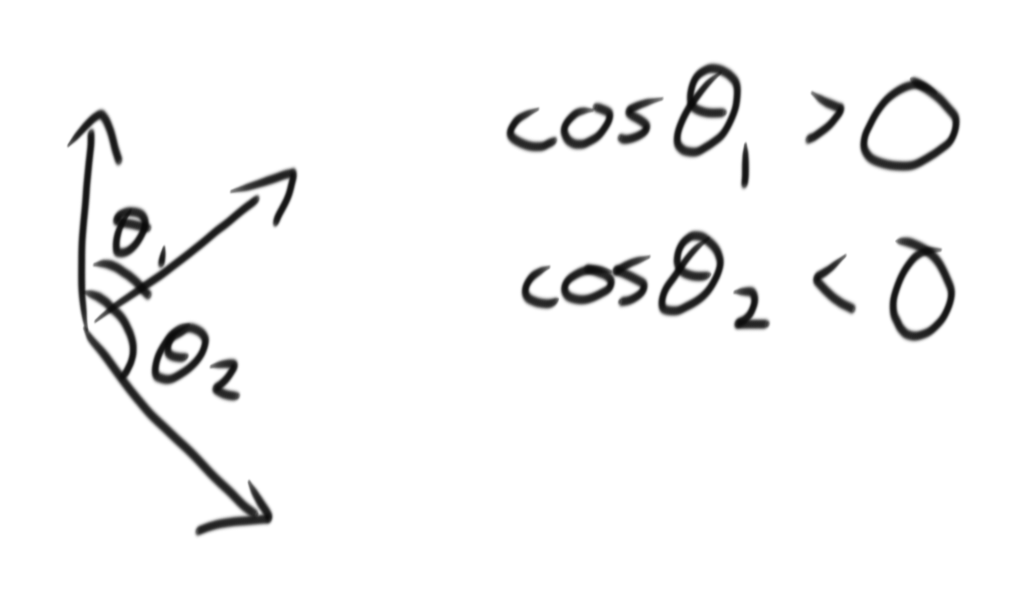
You can use this to tell if two vectors are facing the same direction. If the cosine of the angle between them (the dot product) is positive, then they are, otherwise, they aren't.
So, you can use this with the unit vector of the camera's facing direction (probably (0, 0, 1) or (0, 0, -1) for you), and the normal vector of the polygon (I'll explain how to calculate this in a bit), to work out whether the polygon is facing the camera. However, you'll want to make sure that the dot product is negative because you're checking if they're pointing towards each other, not in the same direction:
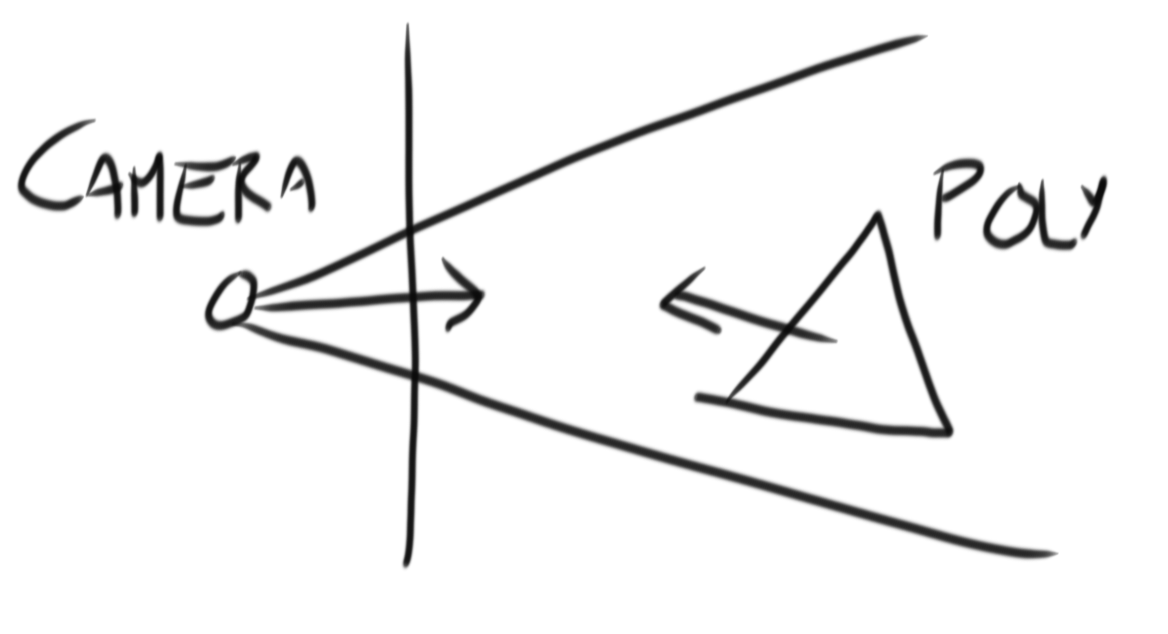
So, time for the normal vector of a polygon. The cross product this time. Basically, A x B gives you a vector perpendicular to both A and B. You can use two edges of the triangle of your polygon for A and B, just note that they both must originate from the same point.
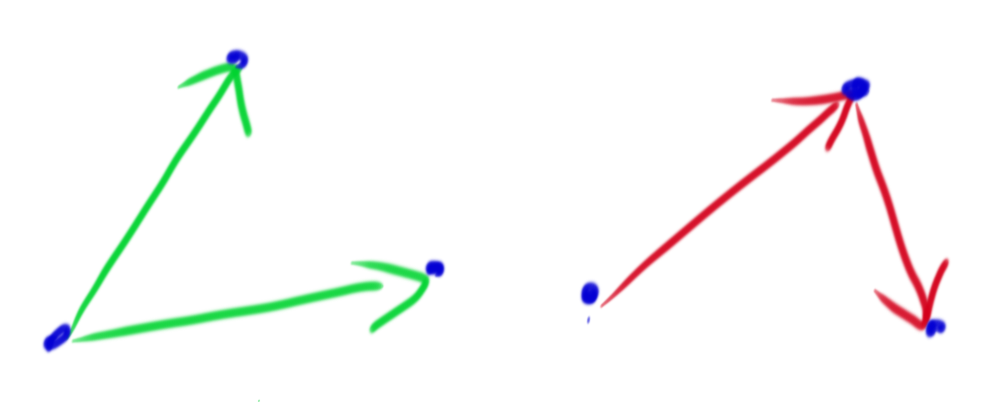
Normalise this (make it a unit vector (divide it by its length)), and you have a normal unit vector (normal vector of the polygon). However… the order matters here. A x B is different to B x A, because there will be two perpendicular vectors to the plane defined by A and B (opposite in direction).
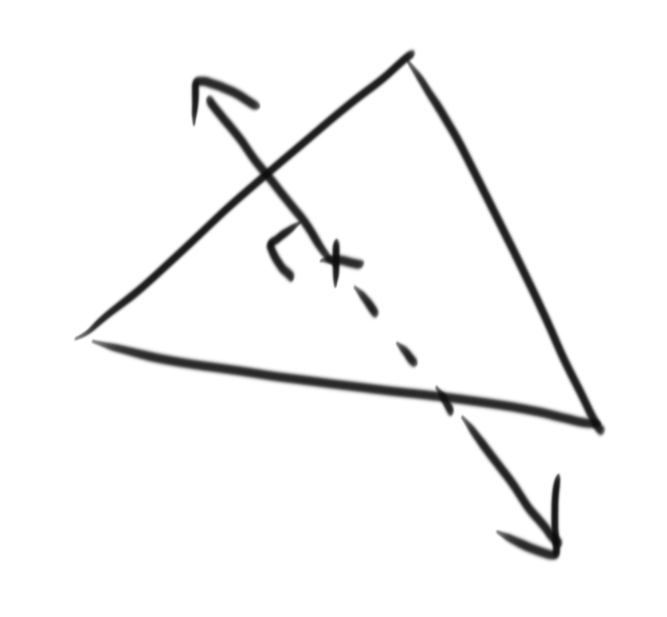
If I recall correctly, this means that you must define the points in your polygon in an anticlockwise manner, so if you had all 3 points with the same Z, they'd go anticlockwise to face the camera. It might be clockwise instead though, experiment a little and you'll find out very quickly. You can always stick a few negatives in places to fix it as well, so basically just be consistent.
The CC vector library has the dot and cross product built in, or you can write your own.
My explanations when it comes to maths are appalling, so do ask if any (or all) of this makes no sense. I've assumed a decent understanding of vectors as you're playing with 3D, but who knows, you might have no clue. I learnt all this from a
GODOT tutorial, so take a look.
Note: The dot product is actually the cosine of the angle multiplied by the magnitude of both vectors, but use unit vectors so the magnitude of both are 1 and therefore can be ignored.