This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
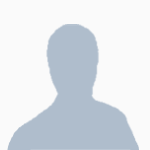
Disable error output on program execution
Started by The Penguin21, 30 September 2017 - 09:04 AMPosted 30 September 2017 - 11:04 AM
Hello. I have been programming a remote control program. The code waits for any incoming rednet message on a specific channel, writes it to a file, executes it, and deletes the file. My problem is, that if i misspell any command on the "controller" computer, it will output an error on the receiver computer. Is it possible to disable it?
Posted 30 September 2017 - 11:29 AM
Have a look at this: https://www.lua.org/pil/8.4.html
Posted 30 September 2017 - 11:53 AM
I really didn't understand that. The program got 2 functions it runs in parallel, so do I add if unexpected_condition then or if pcall(foo) then and where? If the program detects an error, it should just ignore it.
Edited on 30 September 2017 - 09:54 AM
Posted 30 September 2017 - 12:56 PM
Ok, so tried to simulate the situation, I created a file called test with the following code:
function foo ()
shell.run("file")
end
if pcall(foo) then
print("no error")
else
print("yes error")
end
and another file called file with some random text in it which cannot be executed. It doesn't seem to be working, I probably did something wrong.Edited on 30 September 2017 - 10:57 AM
Posted 30 September 2017 - 02:12 PM
Can you post your code? The code for your project.
Edited on 30 September 2017 - 12:13 PM
Posted 30 September 2017 - 02:16 PM
function makeError(n)
return 'N'+n;
end
if pcall(makeError,n) then
print("no error!")
else
print("That method is broken, fix it!")
end
This is the latest code i came across with, it actually seems to be working in some cases, but in my case, where the program has a chance of being misspelled, it won't work.Edited on 30 September 2017 - 12:16 PM
Posted 30 September 2017 - 02:27 PM
pcall only takes one argument, the function to call. If you want to pass an argument to your function you will have to wrap the function like so.
What's the advantage of using the anonymous function over giving it a name? It looks a bit cleaner in code and the function only exists for when it is needed.
--# in this example I want to pass the variable 'a' as an argument to a function which I am pcall-ing
--# I'm using the function 'print' as the function I want to call but this works the same for any function
--# what we are passing is not important for this example
local a = "abcd"
--# define a wrapper function which passes the argument for us
local function f()
print(a)
end
--# pass pcall the wrapper function
pcall(f)
--# alternatively you could use an anonymous function
--# think this as a temperary wrapper function which is discarded after use
pcall(function() print(a) end)
--# if you run this code you should find that it prints 'abcd' twice on two seperate lines
What's the advantage of using the anonymous function over giving it a name? It looks a bit cleaner in code and the function only exists for when it is needed.
Edited on 30 September 2017 - 12:37 PM
Posted 30 September 2017 - 02:54 PM
Thanks for the help, but this isn't really what I am looking for. I am trying to make a program that executes a specific file, but the file it executes contains some random non-executeable characters like "jfewfh98h8f". It should try to execute it if possible, but if not, it should just simply ignore it and NOT print any error to the screen.
Posted 30 September 2017 - 02:58 PM
Try this. It shouldn't print any sort of error to the screen.
local filename = "foo"
local file = fs.open( filename )
local data = file.readAll()
file.close()
pcall( loadstring( data, filename ) )
Posted 30 September 2017 - 03:07 PM
I get this error:
test:2: Expected string, string
When i fix that error i get another error:
test:3: attempt to call nil
test:2: Expected string, string
When i fix that error i get another error:
test:3: attempt to call nil
Edited on 30 September 2017 - 01:06 PM
Posted 30 September 2017 - 03:13 PM
Oops! That's my fault. Add "r" as a second argument on line 2.
Posting from mobile.
Posting from mobile.
Posted 30 September 2017 - 03:24 PM
Thanks! It seems to be working! :D/>
Posted 02 October 2017 - 12:17 AM
Was gonna say, if you want to completely disable errors you could just do..
local error = function() return nil end
local printError = function() return nil end
That would disable errors for that program, but would fix itself outside of it.Posted 02 October 2017 - 12:36 AM
Was gonna say, if you want to completely disable errors you could just do..That would disable errors for that program, but would fix itself outside of it.local error = function() return nil end local printError = function() return nil end
Any errors not manually raised would still occur, as the error handling mechanism wouldn't be using your copy of error or printError.
Posted 02 October 2017 - 06:37 AM
Posted 02 October 2017 - 01:24 PM
I wonder what lead me to belive otherwise, did CC not have this for a while?
Posted 04 October 2017 - 01:01 AM
I wonder what lead me to belive otherwise, did CC not have this for a while?
Well it makes sense. If you want to 'pcall' creating a new box or something with specific diameters and catch an error if something was put in wrong then you would need those extra arguments..
Posted 04 October 2017 - 01:41 AM
Well it makes sense. If you want to 'pcall' creating a new box or something with specific diameters and catch an error if something was put in wrong then you would need those extra arguments..
Not necessarily. You could always wrap it in an anonymous function. Obviously it's more convenient to pass the arguments to pcall though.
pcall( function() createNewBox( a, b, c, d ) end )
Posted 04 October 2017 - 03:10 AM
I wonder what lead me to belive otherwise, did CC not have this for a while?
You're probably thinking of the parallel API calls.
Posted 04 October 2017 - 05:13 AM
Well it makes sense. If you want to 'pcall' creating a new box or something with specific diameters and catch an error if something was put in wrong then you would need those extra arguments..
Not necessarily. You could always wrap it in an anonymous function. Obviously it's more convenient to pass the arguments to pcall though.pcall( function() createNewBox( a, b, c, d ) end )
Ah yes, I keep forgetting about anonymous functions.
With this in mind, why doesn't he just 'load()' the collected string from rednet (pretty sure we talked about this recently when I had that problem) instead of writing it to a file and then executing the file?