local turn = 'left'
local lightBlock
function moveSpace(n)
print("Log:moveSpace: Called " .. tostring(n))
local i = 0
while i < n do
if turtle.detect() then
print("Log:moveSpace: Block In Front of Turtle")
if canForward() then
print("Log:moveSpace: Block is a Breakable")
turtle.dig()
turtle.forward()
i = i + 1
end
else
turtle.forward()
i = i + 1
end
end
print("Log:moveSpace: Done")
end
function logLightBlock()
if turtle.getItemCount(1) ~= 0 then
lightBlock = turtle.getItemDetail(1).name
elseif turtle.getItemCount(14) ~= 0 then
lightBlock = turtle.getItemDetail(14).name
elseif turtle.getItemCount(15) ~= 0 then
lightBlock = turtle.getItemDetail(15).name
elseif turtle.getItemCount(16) ~= 0 then
lightBlock = turtle.getItemDetail(16).name
else
print("------------")
print("Error: Unable to properly log the light block!! Program may not work as intended.")
print("------------")
sleep(4)
end
end
function restockMainSlot()
if turtle.getItemCount(1) == 0 and lightBlock ~= nil then
if turtle.getItemCount(14) ~= 0 then
if turtle.getItemDetail(14).name == lightBlock then
turtle.select(14)
turtle.transferTo(1)
turtle.select(1)
elseif turtle.getItemCount(15) ~= 0 then
if turtle.getItemDetail(15).name == lightBlock then
turtle.select(15)
turtle.transferTo(1)
turtle.select(1)
elseif turtle.getItemCount(16) ~= 0 then
if turtle.getItemDetail(16).name == lightBlock then
turtle.select(16)
turtle.transferTo(1)
turtle.select(1)
end
end
end
end
end
end
function canForward()
if not turtle.detect() then
return true
end
turtle.select(3)
if turtle.compare() == true then
turtle.select(1)
return true
else
turtle.select(4)
if turtle.compare() == true then
turtle.select(1)
return true
else
turtle.select(1)
return false
end
end
end
function checkStock()
if turtle.getItemCount(1) == 0 then
restockMainSlot()
while turtle.getItemCount(1) == 0 do
sleep(5)
print("Log:replace: Resources Needed")
end
end
end
function replace()
turtle.select(5)
if turtle.compareDown() or not turtle.detectDown() then
print("Log:replace: Replacing Block Below")
turtle.digDown()
turtle.select(1)
checkStock()
turtle.placeDown()
else
print("Log:replace: Unable to Replace Block Below")
checkStock()
turtle.select(1)
end
end
function checkFuel()
if turtle.getFuelLevel() < 100 then
turtle.select(2)
print("Log:checkFuel: Fuel Low, Refueling")
turtle.refuel()
turtle.select(1)
end
end
function makeTurn(dir)
if not canForward() then
if dir == 'left' then
turtle.turnLeft()
elseif dir == 'right' then
turtle.turnRight()
end
if not canForward() then
if dir == 'right' then
turtle.turnLeft()
elseif dir == 'left' then
turtle.turnRight()
end
if dir == 'right' then
turtle.turnLeft()
elseif dir == 'left' then
turtle.turnRight()
end
if not canForward() then
return '0'
else
moveSpace(5)
if dir == 'right' then
turtle.turnLeft()
elseif dir == 'left' then
turtle.turnRight()
end
replace()
return oppDir(dir)
end
else
moveSpace(5)
if dir == 'left' then
turtle.turnLeft()
elseif dir == 'right' then
turtle.turnRight()
end
replace()
return oppDir(dir)
end
else
return '0'
end
end
function oppDir(dir)
if dir == 'left' then
return 'right'
elseif dir == 'right' then
return 'left'
end
end
logLightBlock()
replace()
while true do
if canForward() == true then
moveSpace(5)
replace()
checkFuel()
else
turn = makeTurn(turn)
if turn == '0' then
return false
end
end
end
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
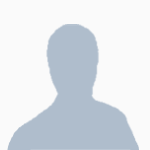
[1.12.2] Reduce Lag?
Started by Mr_Pyro, 31 December 2017 - 03:09 AMPosted 31 December 2017 - 04:09 AM
Hi, I created the program below to place glowstone blocks to light up a room. You give it [slot 1] block to place [slot 2] fuel [slot 3 & 4] blocks its allowed to break (torches or other temporary lighting solutions) [slot 14-16] extra glowstone or blocks that match what was placed in slot 1. It works well with only 1 or 2 small bugs, but it causes a lot of lag. Are there any ways to make it more efficient and cause less lag?
Posted 31 December 2017 - 03:13 PM
replace all sleeps with sleep(0) it'll make it as fast as possible. Only sleep where you're in a loop (as thats where you really need the yield)
Posted 31 December 2017 - 03:43 PM
The only sleeps in the above code occur when waiting for user input: changing that shouldn't have any effect on how fast the code runs.replace all sleeps with sleep(0) it'll make it as fast as possible. Only sleep where you're in a loop (as thats where you really need the yield)
If you're talking about in-game lag (rather than in-computer Lag), there doesn't seem to be anything in your code which should cause issues. Minecraft's lighting engine isn't super efficient, but I doubt you're placing lights fast enough for it to become an issue. If it's framerate lag, it might be worth using Shift+F3's graph to see where the issue is. If it's TPS lag (blocks take a while to respond to right clicks, etc…) I'd recommend using a profiling mod to gather more information.
Posted 01 January 2018 - 05:05 AM
Just gonna point out real quick, if you're running it on a server, it's gonna be a lot laggier than on single player.
Posted 02 January 2018 - 03:07 AM
its single player
Posted 02 January 2018 - 11:10 AM
Do you still get lag if the turtle doesn't replace the lights but otherwise is running the program?
Posted 02 January 2018 - 12:54 PM
… and what if you simply have the turtle roam around using a simple loop (forward, turn left, forward, turn left, forward, turn left…)? Odds are you can at least narrow this down to the use of a specific function.
In terms of tweaking the above script, while I doubt it'll affect your frame rate at all, a few optimisations come to mind. In particular, I don't like your canForward() function - if you have access to turtle.getItemDetail() then you have access to turtle.inspect(), so there's no need for all this slot-changing and block-comparing every single time the turtle wants to move anywhere. Let's reduce it:
In terms of tweaking the above script, while I doubt it'll affect your frame rate at all, a few optimisations come to mind. In particular, I don't like your canForward() function - if you have access to turtle.getItemDetail() then you have access to turtle.inspect(), so there's no need for all this slot-changing and block-comparing every single time the turtle wants to move anywhere. Let's reduce it:
local diggableBlocks = {["minecraft:dirt"] = true, ["minecraft:stone"] = true, ["minecraft:gravel"] = true} -- Add whatever blocks you like to the list.
function canForward()
local found, block = turtle.inspect()
if not found then return true end
return diggableBlocks[block.name] -- Returns either true or nil, depending on whether the name exists in the table or not.
end
Posted 11 January 2018 - 11:11 PM
Do you still get lag if the turtle doesn't replace the lights but otherwise is running the program?
yes
… and what if you simply have the turtle roam around using a simple loop (forward, turn left, forward, turn left, forward, turn left…)? Odds are you can at least narrow this down to the use of a specific function.
In terms of tweaking the above script, while I doubt it'll affect your frame rate at all, a few optimisations come to mind. In particular, I don't like your canForward() function - if you have access to turtle.getItemDetail() then you have access to turtle.inspect(), so there's no need for all this slot-changing and block-comparing every single time the turtle wants to move anywhere. Let's reduce it:local diggableBlocks = {["minecraft:dirt"] = true, ["minecraft:stone"] = true, ["minecraft:gravel"] = true} -- Add whatever blocks you like to the list. function canForward() local found, block = turtle.inspect() if not found then return true end return diggableBlocks[block.name] -- Returns either true or nil, depending on whether the name exists in the table or not. end
while true do
turtle.turnleft()
end
drops my fps down to 70 and anything with computercraft is laggy temp reduced frames while in computercraft ui, any turtle action reduced frames …
yeah that was a temp solution to allow the program to be adapted to work in a variety of situations (ver 0.0.1) the plan was to rewrite the program to go through a setup process (have the user place blocks in front and log them instead of using inv slots)
-sorry for taking so long to reply
Edited on 11 January 2018 - 10:12 PM
Posted 12 January 2018 - 11:48 AM
What if the turtle breaks and replaces one light without moving?
Given that you had lag when the turtle didn't replace the light, I think it might be the turtle moving. The above request is to double check.
Given that you had lag when the turtle didn't replace the light, I think it might be the turtle moving. The above request is to double check.
Posted 12 January 2018 - 09:47 PM
What if the turtle breaks and replaces one light without moving?
Given that you had lag when the turtle didn't replace the light, I think it might be the turtle moving. The above request is to double check.
while true do
turtle.digDown()
turtle.placeDown()
end
fps drops down to 70 fps
Posted 14 January 2018 - 10:59 PM
Is the issue still present without other mods? Especially mods which effect rendering ect. such as Optifine, Fastcraft, FPSBoost, ect.
Posted 14 January 2018 - 11:47 PM
Is the issue still present without other mods? Especially mods which effect rendering ect. such as Optifine, Fastcraft, FPSBoost, ect.
I updated all the mods to the current versions. Optifine was not updated but ctm was. The blocks that I used as the floor is a connected texture block from chisel. After the mods were updated it does not lag as much as before while the turtle is running. I have also noticed something else. No mater what my fps is locked at it always only drops about 10-15 fps (locked 120 -> 110-105, locked 180 -> 170-165 etc.)
Posted 15 January 2018 - 11:44 AM
So you have not tested this without other mods yet? I would recomend that you do, it may not be computercraft which is the root cause of the issue.
Posted 15 January 2018 - 10:48 PM
So you have not tested this without other mods yet? I would recomend that you do, it may not be computercraft which is the root cause of the issue.
yes i tested it without ctm and optifine no change
Posted 16 January 2018 - 11:00 AM
Uninstall every mod other than CC.
Posted 16 January 2018 - 11:03 AM
The one and only way to play Minecraft.Uninstall every mod other than CC.
In all seriousness, do you think you could upload your mod pack and world somewhere? I'd be happy to run a profiler over it and see what's at fault. I don't think it's CC, but you never know with modded…
Posted 17 January 2018 - 03:07 AM
um okThe one and only way to play Minecraft.Uninstall every mod other than CC.
In all seriousness, do you think you could upload your mod pack and world somewhere? I'd be happy to run a profiler over it and see what's at fault. I don't think it's CC, but you never know with modded…
[twitch profile version]
http://www.mediafire.com/file/d3y5afehvvbaduz/Direwolf20_Tech_Only_1.12-1.4.0.zip
[non-twitch version]
http://www.mediafire.com/folder/4khaq1spf6fhg/Minecraft_Mod_Pack_1.12_(DireWolf)
Edited on 17 January 2018 - 02:09 AM