Thanks!
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
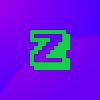
Is it possible to run two loops in one file?
Started by MarcoPolo0306, 17 April 2018 - 10:46 PMPosted 18 April 2018 - 12:46 AM
For example, having buttons at the same time as running a textbox, time shower, etc.
Thanks!
Thanks!
Posted 18 April 2018 - 12:58 AM
Posted 18 April 2018 - 05:24 PM
Also if you just need to capture multiple events(e.g. Click event for Buttons, Key events for textboxes, timer events, etc.) you can use something like this:
while true do
e = {os.pullEvent()}
if e[1] == "key" then
--Handle key event
local key = e[2]
elseif e[1] == "timer" then
--Handle timer event
local timerID = e[2]
end
--ETC.
end
Edited on 20 April 2018 - 04:05 PM
Posted 18 April 2018 - 06:08 PM
The os.pullEvent stuff makes no sense.Also if you just need to capture multiple events(e.g. Click event for Buttons, Key events for textboxes, timer events, etc.) you can use something like this:while true do e = {os.pullEvent} if e[1] == "key" then --Handle key event local key = e[2] elseif e[1] == "timer" then --Handle timer event local timerID = e[2] end --ETC. end
- you need to call the function, not just use the function in the table
- this function will only get the event that you specify as argument, and you didn't specify one.
- I think you want to use os.pullEventRaw to get every event type without filter
Edited on 18 April 2018 - 04:09 PM
Posted 18 April 2018 - 06:25 PM
The os.pullEvent stuff makes no sense.Also if you just need to capture multiple events(e.g. Click event for Buttons, Key events for textboxes, timer events, etc.) you can use something like this:while true do e = {os.pullEvent} if e[1] == "key" then --Handle key event local key = e[2] elseif e[1] == "timer" then --Handle timer event local timerID = e[2] end --ETC. end
Don't take this personally ;)/>, its just a critic on the code.
- you need to call the function, not just use the function in the table
- this function will only get the event that you specify as argument, and you didn't specify one.
- I think you want to use os.pullEventRaw to get every event type without filter
The only error I see in Luca_S' code was the lack of brackets after os.pullEvent. It should look like this…
local e = { os.pullEvent() }
I'm not sure I understand your second point, but if I am understanding correctly then I think you are misunderstanding what is happening with the code. Using e as a table to capture all the values returned by os.pullEvent() is perfectly valid and would work fine as posted in Luca_S' code block. I prefer to use separate variables, but capturing everything in a table works just as well. os.pullEvent() doesn't require an event/filter be specified.
As for capturing all events without filter (except terminate events), you don't need os.pullEventRaw - that's only ever necessary (as far as I know) if you need/want to capture or ignore terminate events. For most cases, os.pullEvent() is fine for pulling events without using a filter.
My apologies if I've misunderstood what you were saying.
Having said all that - without having a better idea of what OP is trying to do, I can't say which approach would be better (parallel api or just a looped event listener).
Edited on 18 April 2018 - 04:28 PM
Posted 19 April 2018 - 10:53 AM
As for capturing all events without filter (except terminate events), you don't need os.pullEventRaw - that's only ever necessary (as far as I know) if you need/want to capture or ignore terminate events.
Just to be clear on this, while it's true that both os.pullEvent() and os.pullEventRaw() may return most event types when called without a filter, os.pullEventRaw() doesn't "ignore" terminate events. In fact, if they show up in the event queue, it'll return them even if you pass in a filter asking for a different type!
Posted 20 April 2018 - 06:07 PM
Added the brackets, thanks for pointing that out. To be honest, I think your other points show that you lack an understanding of what the difference between os.pullEvent and os.pullEventRaw is.The os.pullEvent stuff makes no sense.Also if you just need to capture multiple events(e.g. Click event for Buttons, Key events for textboxes, timer events, etc.) you can use something like this:while true do e = {os.pullEvent} if e[1] == "key" then --Handle key event local key = e[2] elseif e[1] == "timer" then --Handle timer event local timerID = e[2] end --ETC. end
Don't take this personally ;)/>, its just a critic on the code.
- you need to call the function, not just use the function in the table
- this function will only get the event that you specify as argument, and you didn't specify one.
- I think you want to use os.pullEventRaw to get every event type without filter
I added this solution because in some cases this might be better than using the Parallel API as was suggested before.
Posted 21 April 2018 - 06:45 AM
Yeah, I was completely wrong about the os.pullEvent stuff. It just didn't make much sense to me that there where two functions that do the exact same thing, just that os.pullEventRaw gets terminate events too. I thought os.pullEventRaw gets events raw without a filter, and os.pullEvent gets a specifc event.To be honest, I think your other points show that you lack an understanding of what the difference between os.pullEvent and os.pullEventRaw is.
Edited on 21 April 2018 - 04:46 AM