–Cheeky P.S: the api for moniters is here: http://www.computercraft.info/wiki/index.php?title=Peripheral_(API)#Monitor
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
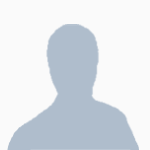
help with monitors.
Started by cheekycharlie101, 02 August 2012 - 06:59 PMPosted 02 August 2012 - 08:59 PM
ok so im a noob at computer craft. i can code a passworded door and thats about it. anyway, i want to know how i can make my text be centred in the middle of the monitor. im not sure how to put text on new lines or how to put it in the middle. please could someoen tell me. a awesome guy named kopola (or something, couldent remeber his actual namexD) helped me a lot. but i couldent figure this out. i also checked this api but i dident get very far with it, pleaseee can someone help me thanks
–Cheeky P.S: the api for moniters is here: http://www.computercraft.info/wiki/index.php?title=Peripheral_(API)#Monitor
–Cheeky P.S: the api for moniters is here: http://www.computercraft.info/wiki/index.php?title=Peripheral_(API)#Monitor
Posted 02 August 2012 - 09:10 PM
Well, as far as I know, there's no easy way to set text to the middle of a monitor, but if you work out how many characters fit across the screen at your chosen text size, divide it by two, you get the number of characters on one half of the screen. Then, if you divide the number of characters in your chosen text by two and subtract that number from the number of characters on one half of the screen, you know how far across the screen you need to set the cursor before you write the text to the monitor.
It sounds a little long, but if you know what text you will output, you can do it that way.
I'd like to know if there is a way to shorten this process, but I've never had to try before.
It sounds a little long, but if you know what text you will output, you can do it that way.
I'd like to know if there is a way to shorten this process, but I've never had to try before.
Posted 02 August 2012 - 09:15 PM
Okay, so for making a new line, you can use the escape character "n".
For centering the text, that takes some code.
This is a very simple method, and only works if the string would normally fit in the space of the monitor. I would write the rest, but I think this will get you started.
EDIT: Whoops! Small error. Code should work now.
For centering the text, that takes some code.
function centerTextMon(mon, inString) -- mon is the wrapped monitor (peripheral.wrap(side)), inString is the string to be written, centered
monX, monY = mon.getSize()
newX = string.len(inString)/2
newX = (monX/2) - newX
mon.setCursorPos (newX, newY) -- Set your own height
end
This is a very simple method, and only works if the string would normally fit in the space of the monitor. I would write the rest, but I think this will get you started.
EDIT: Whoops! Small error. Code should work now.
Posted 02 August 2012 - 09:15 PM
Copied from HERE :
local tText = {
"First line", -- you can change the lines here
"Second line",
"Some more",
"lines here"
-- and you can add more if you want, just dont forget to put the commas (,) at the end of the lines (except for the last one)
}
local sSide = "left" --change to side of your monitor
local nTextSize = 1 -- change the text size here (from 1 to 5)
local function printCenter(mon, t)
local w, h = mon.getSize()
local y = math.floor((h / 2) - (#t / 2)) - 1
for i, line in ipairs(t) do
local x = math.floor((w / 2) - (#line / 2))
mon.setCursorPos(x, y + i)
mon.write(line)
end
end
local mon = peripheral.wrap(sSide)
mon.setTextScale(nTextSize)
printCenter(mon, tText)
You should be able to enter anything in that top section, and have it automatically centered.Posted 02 August 2012 - 09:18 PM
This function should do what you want:
To make it print to a monitor, you need to redirect the output to it first:
Edit:
Wow, a little late :ph34r:/>/>
local function printCenter(s, centerY)
local w, h = term.getSize()
local x, y = term.getCursorPos()
x = math.floor((w / 2) - (#s / 2))
if centerY then
y = math.floor(h / 2)
end
term.setCursorPos(x, y)
print(s)
end
It takes a string and an optional boolean as parameters. If the second parameter is true it will center it vertically.To make it print to a monitor, you need to redirect the output to it first:
local mon = peripheral.wrap("<side>")
term.redirect(mon)
printCenter("Some Text", true) -- print something to the middle of the monitor
term.restore()
Edit:
Wow, a little late :ph34r:/>/>
Posted 03 August 2012 - 02:55 PM
i tried your method because it look simplest. but got this error: bios:206: [string "test}:16: '<eof>' expectedCopied from HERE :You should be able to enter anything in that top section, and have it automatically centered.local tText = { "First line", -- you can change the lines here "Second line", "Some more", "lines here" -- and you can add more if you want, just dont forget to put the commas (,) at the end of the lines (except for the last one) } local sSide = "left" --change to side of your monitor local nTextSize = 1 -- change the text size here (from 1 to 5) local function printCenter(mon, t) local w, h = mon.getSize() local y = math.floor((h / 2) - (#t / 2)) - 1 for i, line in ipairs(t) do local x = math.floor((w / 2) - (#line / 2)) mon.setCursorPos(x, y + i) mon.write(line) end end local mon = peripheral.wrap(sSide) mon.setTextScale(nTextSize) printCenter(mon, tText)
Posted 03 August 2012 - 03:13 PM
OOPS, had an extra "end" in there. Here you go:
local tText = {
"First line", -- you can change the lines here
"Second line",
"Some more",
"lines here"
-- and you can add more if you want, just dont forget to put the commas (,) at the end of the lines (except for the last one)
}
local sSide = "left" --change to side of your monitor
local nTextSize = 1 -- change the text size here (from 1 to 5)
local function printCenter(mon, t)
local w, h = mon.getSize()
local y = math.floor((h / 2) - (#t / 2)) - 1
for i, line in ipairs(t) do
local x = math.floor((w / 2) - (#line / 2))
mon.setCursorPos(x, y + i)
mon.write(line)
end --there was an extra end here
local mon = peripheral.wrap(sSide)
mon.setTextScale(nTextSize)
printCenter(mon, tText)
Should work now.Posted 03 August 2012 - 03:43 PM
No, it was fine (I wrote that code, and it works), now you'r missing and end to close the function. It should work like before.OOPS, had an extra "end" in there. Here you go:Should work now.local tText = { "First line", -- you can change the lines here "Second line", "Some more", "lines here" -- and you can add more if you want, just dont forget to put the commas (,) at the end of the lines (except for the last one) } local sSide = "left" --change to side of your monitor local nTextSize = 1 -- change the text size here (from 1 to 5) local function printCenter(mon, t) local w, h = mon.getSize() local y = math.floor((h / 2) - (#t / 2)) - 1 for i, line in ipairs(t) do local x = math.floor((w / 2) - (#line / 2)) mon.setCursorPos(x, y + i) mon.write(line) end --there was an extra end here local mon = peripheral.wrap(sSide) mon.setTextScale(nTextSize) printCenter(mon, tText)
Posted 03 August 2012 - 03:48 PM
Then I'm just derpy…. I didn't see the function you had. Pay no attention to me, I'm just ready to get out of work already…..
Posted 03 August 2012 - 03:57 PM
i shall return today my friend i shall returnok so im a noob at computer craft. i can code a passworded door and thats about it. anyway, i want to know how i can make my text be centred in the middle of the monitor. im not sure how to put text on new lines or how to put it in the middle. please could someoen tell me. a awesome guy named kopola (or something, couldent remeber his actual namexD) helped me a lot. but i couldent figure this out. i also checked this api but i dident get very far with it, pleaseee can someone help me thanks
–Cheeky P.S: the api for moniters is here: http://www.computerc...l_(API)#Monitor