Can you help me please ?
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
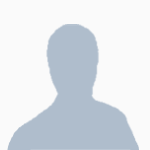
Getting variables and some other stuff from text file
Started by flighteur, 31 August 2012 - 10:22 AMPosted 31 August 2012 - 12:22 PM
I know for a fact that computercraft can create and write to files, but I don't know how to retrieve informations from that file, such as coordinates for turtles, block, etc..
Can you help me please ?
Can you help me please ?
Posted 31 August 2012 - 12:31 PM
if the file with the coords is like this :
x = 1
y = 2
z = 3
then open the file then use a for loop to add each line to a table which will then let you cut out the first 4 characters and leave you with the number of each line or example
x = 1
y = 2
z = 3
then open the file then use a for loop to add each line to a table which will then let you cut out the first 4 characters and leave you with the number of each line or example
nLines = (numberOfLines)
tLines = {} -- table of lines
variable = fs.open("filename", "r") --(you can use io.open aswel but i prefer the fs api)
for i = 1,nLines do -- loops for nLines times
variable2 = variable.read() -- reads the line
if variable2 ~= "" or nil then -- checks if the line is blank or non-existent
variable3 = string.sub(variable2, 5,5) -- cuts out the x = or y = and so on...
table.insert(tLines, variable3) -- inserts the modified line into the table
end
end
i think this should work but it is untested so there might be errors…Posted 31 August 2012 - 12:40 PM
better idea, use a table to store the co-ordinates, so:
mycoords={}
mycoords[1]={['x']=20,['y']=25}
mycoords[2]={['x']=,10['y']=30}
then to store it you simply write the output of textutils.serialize(mycoords) to a file, to get all your data back use
mycoords=textutils.unserialize(io.open('yourfilename','r'):readall)
Posted 31 August 2012 - 01:06 PM
I think the best way of going about this would be to store variables as awsumben said, while reading and interpreting them using string.gmatch instead of string.sub.
in this case, it'd output
x1
y2
z3
You could also store them as a lua-formatted table of variables as KaoS said, and load them using loadfile() and pcall() so that the program doesn't crash.
file = fs.open('your/file/path','r')
local data = file.readAll()
for index,value in data:gmatch('([%w_])%s-=%s-(%d)') do
print(index, value)
end
in this case, it'd output
x1
y2
z3
You could also store them as a lua-formatted table of variables as KaoS said, and load them using loadfile() and pcall() so that the program doesn't crash.
-- the file:
return {
x = 1;
y = 2;
z = 3;
}
-- the file loader
local vars = pcall(loadfile('your/file/path'))
However I'm not even sure if loadstring and loadfile are both available, haha.Posted 31 August 2012 - 01:10 PM
Anover question How can I create a file ? (yes, I think it's a sutpid question but i don't know how to create one.)
Posted 31 August 2012 - 01:12 PM
Pretty sure you can just use fs.write() to a file location that doesn't exist yet.
Posted 31 August 2012 - 01:15 PM
thanks
Posted 31 August 2012 - 01:15 PM
use this to create a file:
k = fs.open("filename", "w")
k.close()
this will also reset a file that exists
k = fs.open("filename", "w")
k.close()
this will also reset a file that exists