Edit: I also need something like this for my upcoming printing API. I'm trying to make patterns with letters in a string.
This is a read-only snapshot of the ComputerCraft forums,
taken in April 2020.
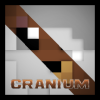
[Lua][Help] String pattern matching
Started by Cranium, 17 September 2012 - 02:55 PMPosted 17 September 2012 - 04:55 PM
I'm trying to make a game of Hangman. Problem is, I do not know how I am going to have it detect any letters the user types. I want to be able to add a word in, and have it be cut apart into separate letters, and have everyone guess it. I know that I need to use something like string.match, or string.gmatch, but the only tutorials i found separate whole words out. To get the letters of a word into a table would help a lot. My reference material was here: http://lua-users.org...atternsTutorial
Edit: I also need something like this for my upcoming printing API. I'm trying to make patterns with letters in a string.
Edit: I also need something like this for my upcoming printing API. I'm trying to make patterns with letters in a string.
Edited on 17 September 2012 - 02:58 PM
Posted 17 September 2012 - 05:17 PM
try this to make an array of the letters in the word…
you can also use string.sub to pull letters out..
local word="hangman"
local letters={}
string.gsub(word,"%a",function(letter) letters[#letters+1]=letter end)
you can also use string.sub to pull letters out..
letters={}
for i=1,#letters do
letters[i]=string.sub(letters,i,i)
end
Posted 17 September 2012 - 05:19 PM
well this is probably an bad workaround but try this:
function seprate(input)
local chars = {}
for x=1,string.len(input) do
table.insert(chars,string.sub(input,x,x))
end
return chars
end
Posted 17 September 2012 - 05:24 PM
Thanks for the help, guys. Although I would like to know how you got to those ideas. For example, why string.sub? I am self taught, and many things are still eluding me with Lua.
Posted 17 September 2012 - 05:30 PM
i also taught lua to myself and at the point that i did that code (its quite old) i didn't knew much about string functions so that was the only thing i knew how to use
Posted 17 September 2012 - 05:33 PM
try this to make an array of the letters in the word…local word="hangman" local letters={} string.gsub(word,"%a",function(letter) letters[#letters+1]=letter end)
you can also use string.sub to pull letters out..letters={} for i=1,#letters do letters[i]=string.sub(letters,i,i) end
why are you editing my resolution into yours ?
Posted 17 September 2012 - 05:36 PM
I ws already editing while you were posting :)/>/>
Posted 17 September 2012 - 05:41 PM
Another version:
local word = "some word"
local letters = {}
for letter in string.gmatch(word, ".") do
table.insert(letters, letter)
end
So many options. Lua is great! :)/>/>Posted 17 September 2012 - 05:47 PM
i call python :)/>/>Another version:So many options. Lua is great! :D/>/>local word = "some word" local letters = {} for letter in string.gmatch(word, ".") do table.insert(letters, letter) end